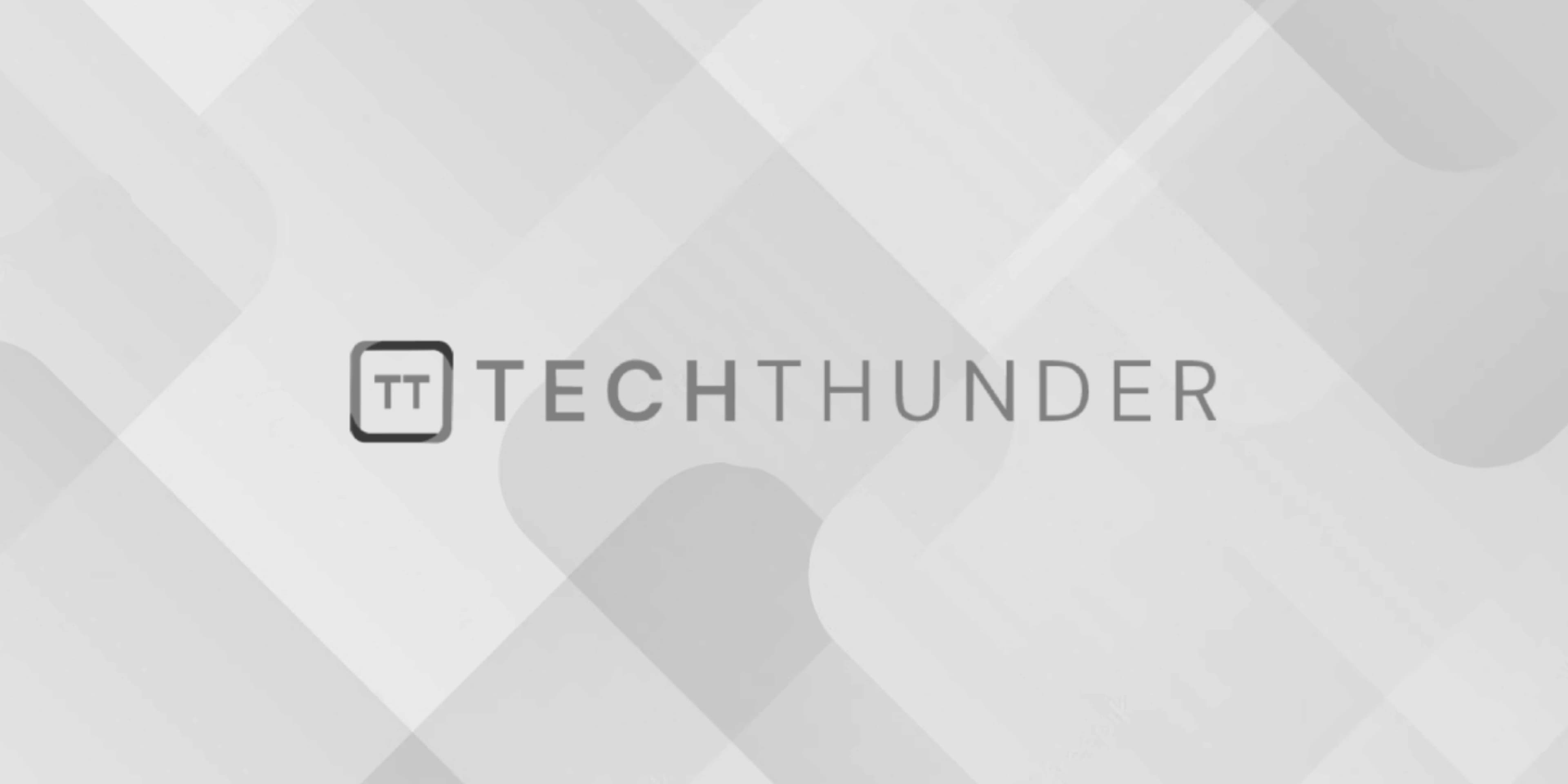
292 views
Java Data Types
The Java data types are used to categorize and specify the type of data a variable can hold. Java provides two categories of data types:
- Primitive Data Types:
- These are the fundamental data types in Java, representing basic values.
- They have fixed memory sizes and are more efficient in terms of memory usage and performance.
- There are eight primitive data types in Java:
- byte: 8-bit signed integer. Range: -128 to 127.
- short: 16-bit signed integer. Range: -32,768 to 32,767.
- int: 32-bit signed integer. Range: -2^31 to (2^31 – 1).
- long: 64-bit signed integer. Range: -2^63 to (2^63 – 1).
- float: 32-bit floating-point. Used for single-precision floating-point numbers.
- double: 64-bit floating-point. Used for double-precision floating-point numbers.
- char: 16-bit Unicode character.
- boolean: Represents true or false values.
- Reference Data Types:
- Reference data types refer to objects, arrays, and classes, and they do not store the actual data but hold references to memory locations where data is stored.
- Reference data types have a default value of
null
if not initialized.
Here’s an example that shows the usage of both primitive and reference data types in Java:
public class DataTypesExample {
public static void main(String[] args) {
// Primitive data types
byte myByte = 127;
short myShort = 32000;
int myInt = 100000;
long myLong = 123456789L;
float myFloat = 3.14f;
double myDouble = 2.71828;
char myChar = 'A';
boolean myBoolean = true;
// Reference data types
String myString = "Hello, Java!";
int[] myArray = {1, 2, 3, 4, 5};
MyClass myObject = new MyClass();
// Using reference data types
System.out.println(myString);
System.out.println(myArray[0]);
myObject.printMessage();
}
}
class MyClass {
void printMessage() {
System.out.println("This is a custom class method.");
}
}
It’s important to choose the appropriate data type for your variables based on the data they will hold and the required memory usage and performance characteristics. Understanding data types is fundamental to Java programming and ensures that your code is both efficient and correct.