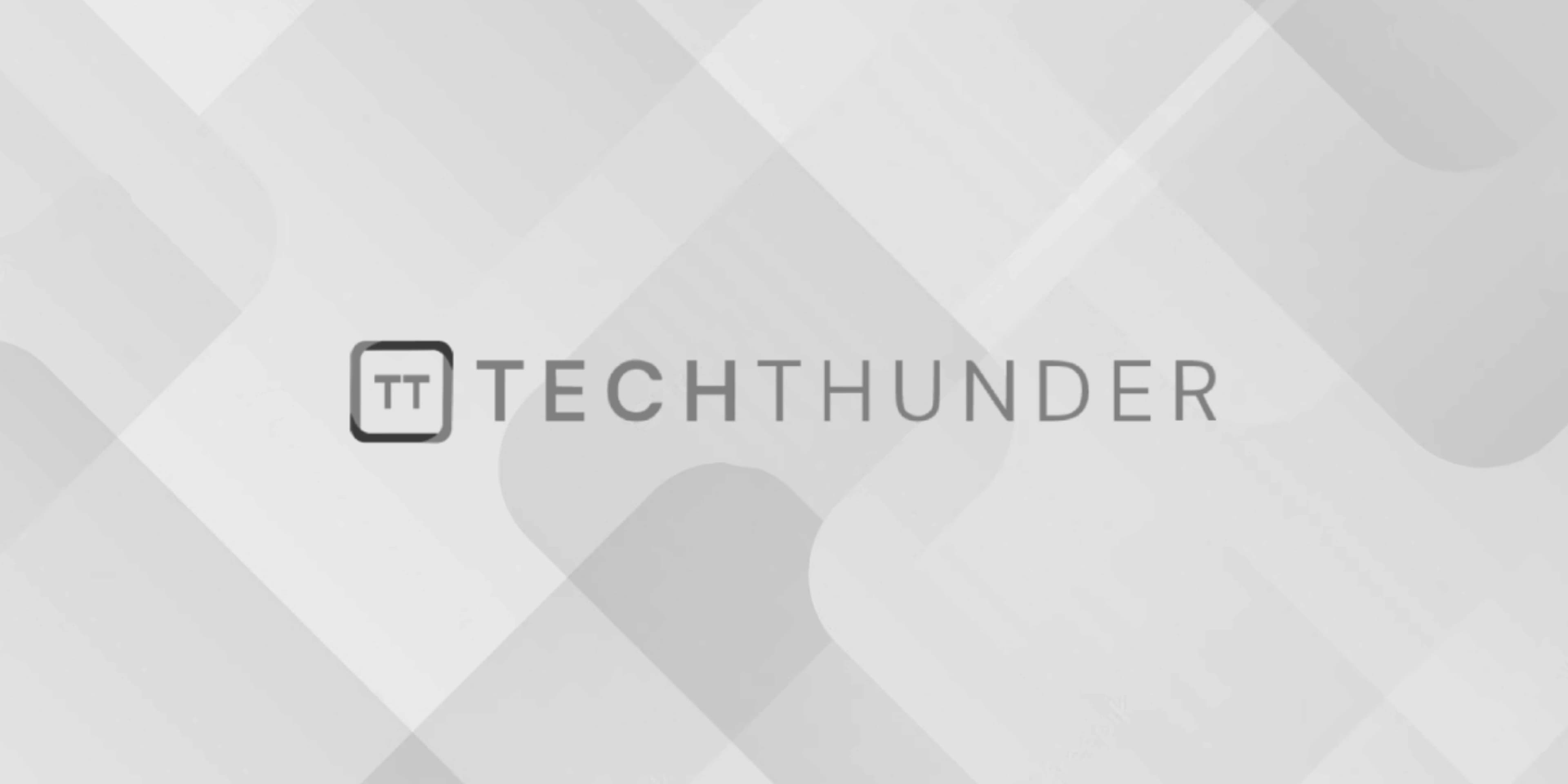
226 views
Java Control Statements
Control statements in Java are used to control the flow of a program. They allow you to make decisions, repeat statements, and break the flow of code into different branches. Java provides several control statements, including:
- Conditional Statements:
- Conditional statements allow you to make decisions in your code. They execute different blocks of code based on certain conditions.
if
Statement:- Executes a block of code if a specified condition is true.
if (condition) { // Code to execute if the condition is true }
if-else
Statement:- Executes one block of code if a condition is true and another block if it’s false.
if (condition) { // Code to execute if the condition is true } else { // Code to execute if the condition is false }
else-if
Statement:- Allows you to test multiple conditions and execute code blocks accordingly.
if (condition1) { // Code to execute if condition1 is true } else if (condition2) { // Code to execute if condition2 is true } else { // Code to execute if none of the conditions are true }
- Switch Statement:
- Evaluates an expression and executes code based on the value of the expression.
java switch (expression) { case value1: // Code to execute if expression equals value1 break; case value2: // Code to execute if expression equals value2 break; default: // Code to execute if none of the cases match }
- Evaluates an expression and executes code based on the value of the expression.
- Looping Statements:
- Looping statements are used to repeat a block of code multiple times.
for
Loop:- Executes a block of code a specific number of times.
for (initialization; condition; update) { // Code to repeat }
while
Loop:- Executes a block of code as long as a specified condition is true.
while (condition) { // Code to repeat }
do-while
Loop:- Similar to a
while
loop, but it guarantees that the block of code is executed at least once before checking the condition.
do { // Code to execute at least once } while (condition);
- Similar to a
- Enhanced
for
Loop (For-each Loop):- Simplifies iteration through collections and arrays.
java for (Type variable : collection) { // Code to process each element }
- Simplifies iteration through collections and arrays.
- Branching Statements:
- Branching statements alter the normal flow of program execution.
break
Statement:- Exits the loop or switch statement.
for (int i = 0; i < 10; i++) { if (i == 5) { break; // Exits the loop when i equals 5 } }
continue
Statement:- Skips the current iteration of a loop and continues with the next iteration.
for (int i = 0; i < 10; i++) { if (i == 5) { continue; // Skips the current iteration when i equals 5 } }
return
Statement:- Exits from a method and optionally returns a value to the calling code.
These control statements are essential for implementing decision-making, loops, and managing the flow of your Java programs. They provide the means to make your code more flexible and responsive to different situations.