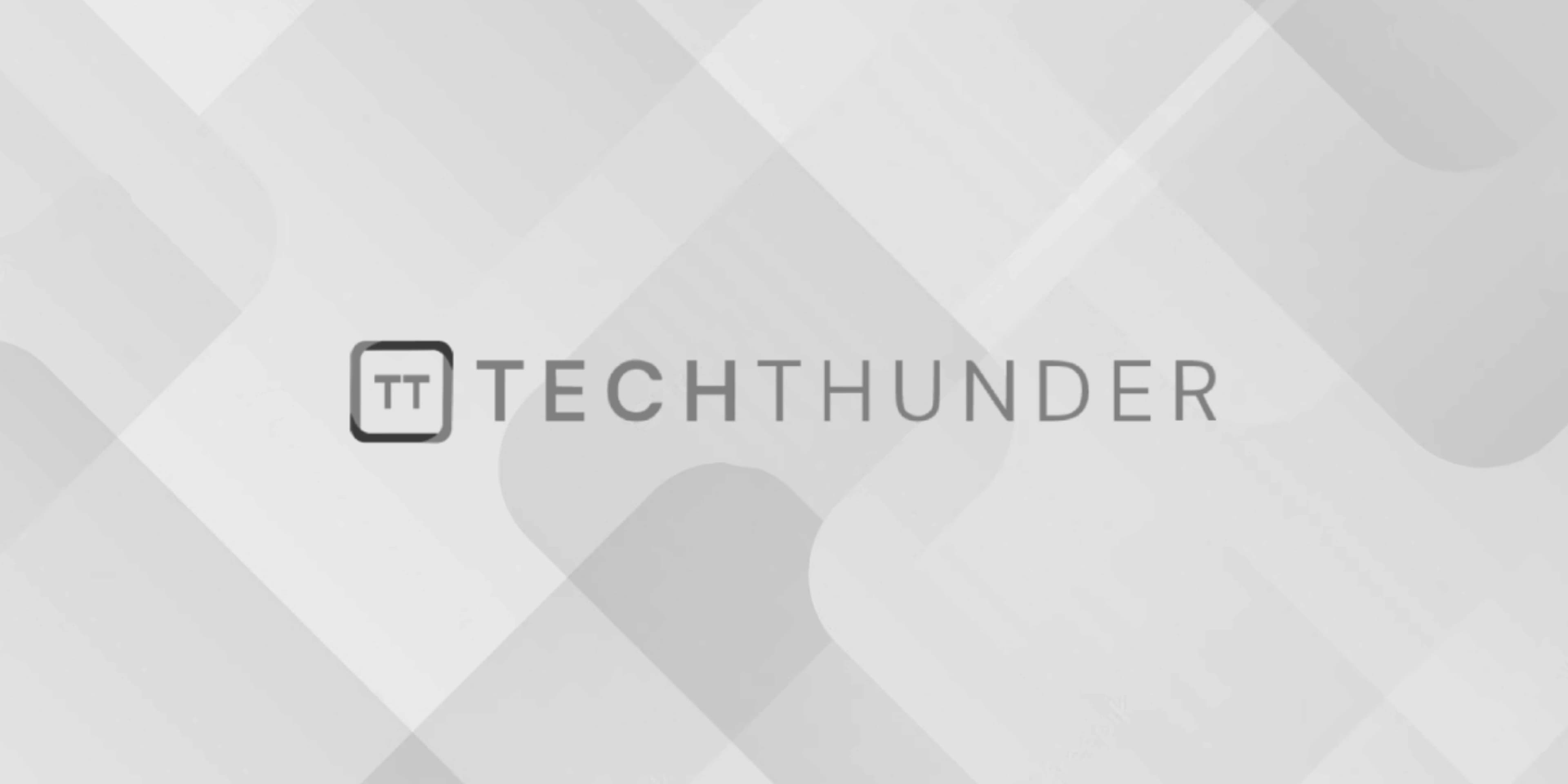
AlertDialog in Android
The Android AlertDialog
is a dialog box that is used to display important information, ask for user confirmation, or prompt the user for a decision. AlertDialogs are a common UI component in Android apps for various purposes, such as displaying messages, asking for user input, or presenting options. Here’s how to create and use an AlertDialog
in Android:
- Create an AlertDialog.Builder: To create an
AlertDialog
, you typically use theAlertDialog.Builder
class. Here’s how to create an instance of it:
AlertDialog.Builder alertDialogBuilder = new AlertDialog.Builder(this);
Replace this
with your activity or context.
- Set Dialog Properties: Customize the appearance and behavior of the AlertDialog using methods like
setTitle
,setMessage
,setPositiveButton
,setNegativeButton
, etc. For example:
alertDialogBuilder.setTitle("Alert Title")
.setMessage("This is an important message.")
.setPositiveButton("OK", new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog, int which) {
// Perform the action when the "OK" button is clicked
}
})
.setNegativeButton("Cancel", new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog, int which) {
// Perform the action when the "Cancel" button is clicked
}
});
You can customize the title, message, and button labels according to your needs. You can also add more buttons or actions as necessary.
- Create the AlertDialog: After configuring the AlertDialogBuilder, create an
AlertDialog
by calling thecreate()
method:
AlertDialog alertDialog = alertDialogBuilder.create();
- Show the AlertDialog: To display the
AlertDialog
to the user, call theshow()
method:
alertDialog.show();
The AlertDialog will now be displayed on the screen with the specified properties and buttons.
Here’s the complete example:
AlertDialog.Builder alertDialogBuilder = new AlertDialog.Builder(this);
alertDialogBuilder.setTitle("Alert Title")
.setMessage("This is an important message.")
.setPositiveButton("OK", new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog, int which) {
// Perform the action when the "OK" button is clicked
}
})
.setNegativeButton("Cancel", new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog, int which) {
// Perform the action when the "Cancel" button is clicked
}
});
AlertDialog alertDialog = alertDialogBuilder.create();
alertDialog.show();
This code creates a simple AlertDialog
with a title, message, and “OK” and “Cancel” buttons. You can customize the dialog’s appearance and behavior by adding more options and button actions as needed.