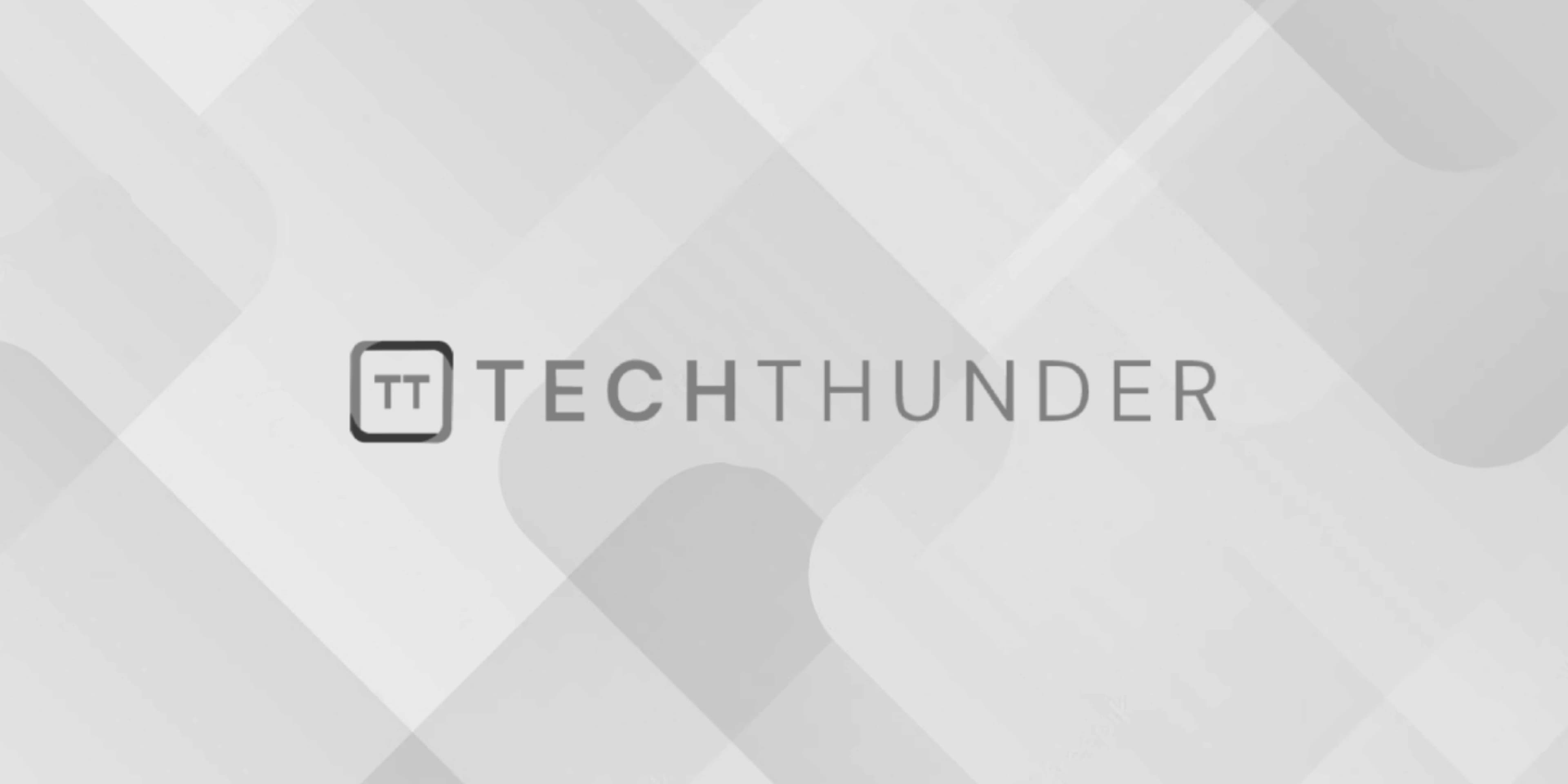
Option Menu in Android
The Android options menu is a user interface component that typically appears at the top of an activity’s screen when the user presses the device’s menu button or taps the “…” (three dots) icon in the app’s action bar (if available). The options menu provides a set of actions or options that are relevant to the current activity, allowing users to perform various tasks within the app.
Here’s how you can create and use an options menu in Android:
Create a Menu Resource: First, create a menu resource XML file in the res/menu
directory of your Android project. This file defines the items that will appear in the options menu. For example, create a file named menu_main.xml
:
<!-- res/menu/menu_main.xml -->
<menu xmlns:android="http://schemas.android.com/apk/res/android">
<item
android:id="@+id/action_settings"
android:title="Settings" />
<item
android:id="@+id/action_about"
android:title="About" />
</menu>
In this example, the menu defines two items: “Settings” and “About.”
Override onCreateOptionsMenu()
: In your activity, override the onCreateOptionsMenu()
method to inflate the menu resource and make the options menu visible:
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.menu_main, menu);
return true;
}
R.menu.menu_main
is the reference to the XML menu resource you created earlier.
Override onOptionsItemSelected()
: Override the onOptionsItemSelected()
method to handle the selection of menu items:
@Override
public boolean onOptionsItemSelected(MenuItem item) {
switch (item.getItemId()) {
case R.id.action_settings:
// Handle the "Settings" item click
return true;
case R.id.action_about:
// Handle the "About" item click
return true;
default:
return super.onOptionsItemSelected(item);
}
}
In this method, you can perform actions or navigate to different parts of your app based on the selected menu item’s ID.
Display the Options Menu: The options menu will automatically be displayed when the user presses the menu button on their device or taps the “…” icon in the action bar (if present).
Options menus provide a user-friendly way to offer additional functionality and actions within your app. They are commonly used for settings, help, about, and other actions that are relevant to the current screen or activity. If you’re using the modern Android Toolbar instead of the legacy ActionBar, the process is similar, but you’ll inflate the menu using the onCreateOptionsMenu()
method of the Toolbar.