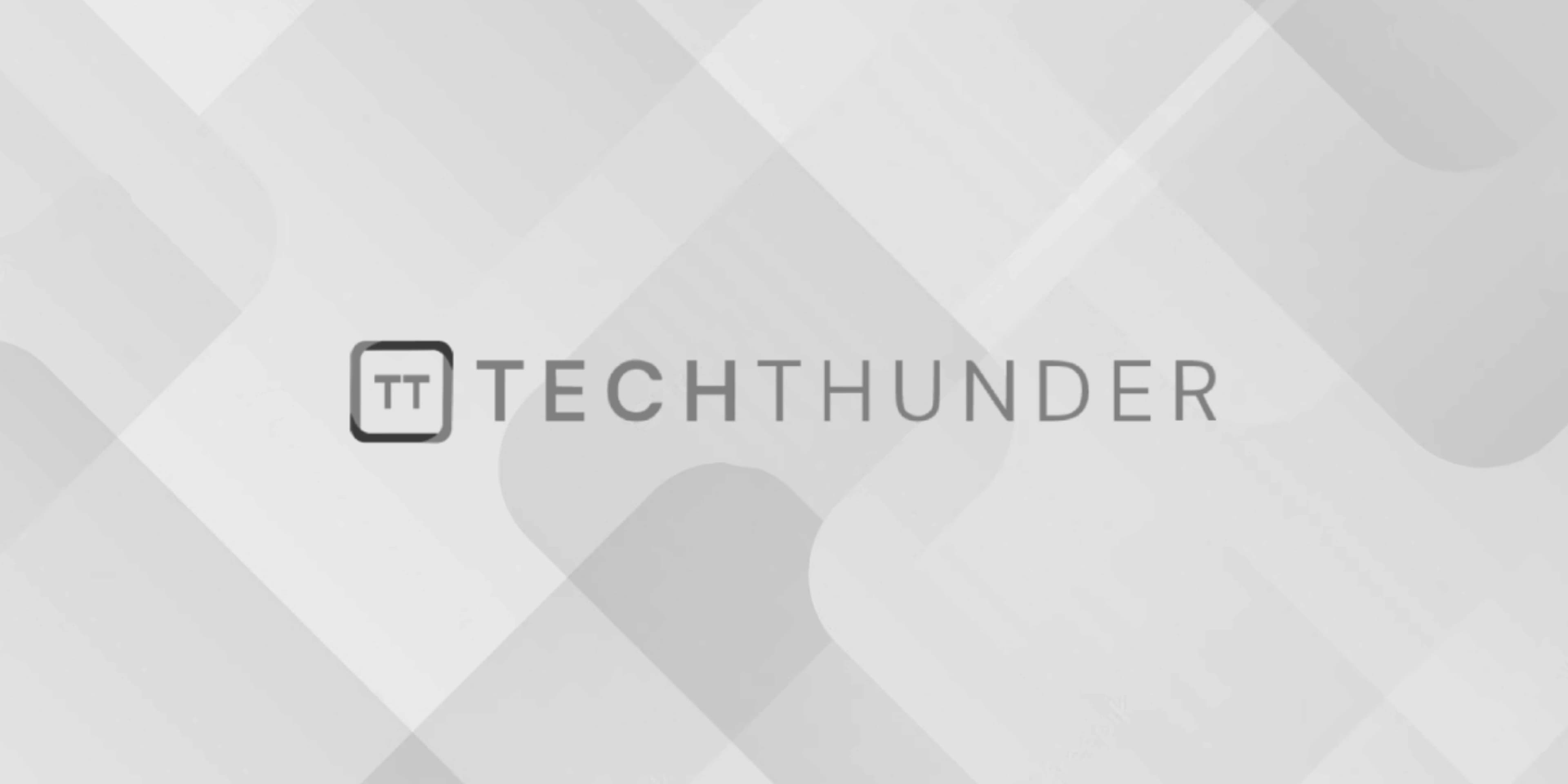
Vertical ScrollView in Android
The Android ScrollView
is a view container that allows its child views to be scrolled vertically, typically when the content within the view is too tall to fit within the screen’s height. It provides a vertical scrollable area for displaying content like long lists, text, images, or other UI elements.
Here’s how you can use a ScrollView
in your Android app:
XML Layout:
- Create a layout XML file where you want to include the
ScrollView
.
<ScrollView
android:id="@+id/scroll_view"
android:layout_width="match_parent"
android:layout_height="match_parent">
<!-- Child views go here, such as TextViews, ImageViews, or other UI elements -->
</ScrollView>
Inside the ScrollView
, you’ll typically include child views that you want to be vertically scrollable.
Child Views:
- Inside the
ScrollView
, add child views that you want to be vertically scrollable. These child views can be any UI elements likeTextView
,ImageView
,Button
, or layouts likeLinearLayout
,RelativeLayout
, etc.
<ScrollView
android:id="@+id/scroll_view"
android:layout_width="match_parent"
android:layout_height="match_parent">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical">
<!-- Child views go here -->
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="This is a long text that can be scrolled vertically." />
<ImageView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:src="@drawable/image1" />
<!-- Add more child views as needed -->
</LinearLayout>
</ScrollView>
In this example, a LinearLayout
is used as a container for vertically arranging the child views.
Programmatic Access:
- In your Java code, you can programmatically access the
ScrollView
and its child views using their IDs:
ScrollView scrollView = findViewById(R.id.scroll_view);
// Access child views within the ScrollView
TextView textView = findViewById(R.id.text_view);
ImageView imageView = findViewById(R.id.image_view);
// ...
Scrolling Programmatically:
- You can programmatically scroll the
ScrollView
to a specific position using methods likescrollTo()
orsmoothScrollTo()
:
scrollView.scrollTo(0, 200); // Scrolls to a specific Y position
Adjustments and Styling:
- You can adjust the width, height, and styling of the
ScrollView
and its child views to fit your layout and design requirements.
The ScrollView
allows you to create vertically scrollable content within your Android app, making it useful for displaying long text, lists, or any content that doesn’t fit entirely on the screen.