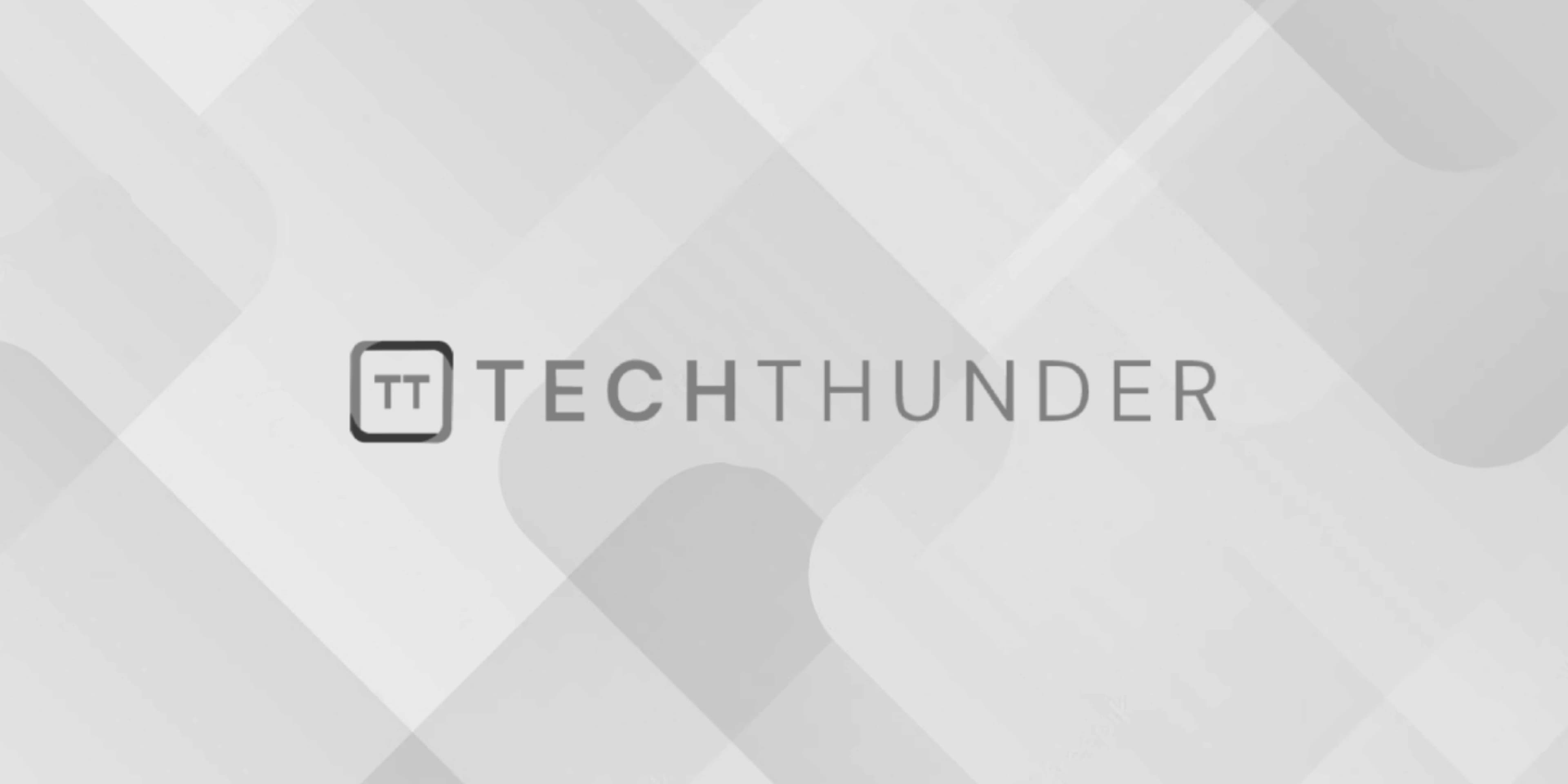
Internal Storage in Android
Internal storage in Android refers to the storage space that is available on the device’s internal memory. It is private to the app, meaning that only the app that created it can access the files stored in internal storage. Internal storage is typically used for storing app-specific data and files that should not be accessible to other apps or the user.
Here’s how you can work with internal storage in Android:
1. Write to Internal Storage:
To write data to internal storage, you can use the FileOutputStream
class. Here’s an example of how to write a text file:
String filename = "myfile.txt";
String content = "Hello, this is some content.";
try {
FileOutputStream fos = openFileOutput(filename, Context.MODE_PRIVATE);
fos.write(content.getBytes());
fos.close();
} catch (Exception e) {
e.printStackTrace();
}
In this code:
openFileOutput(filename, mode)
opens a file in internal storage with the given filename and mode.Context.MODE_PRIVATE
ensures that only the app can access the file.
2. Read from Internal Storage:
To read data from internal storage, you can use the FileInputStream
class. Here’s an example of how to read the previously created text file:
String filename = "myfile.txt";
String content = "";
try {
FileInputStream fis = openFileInput(filename);
InputStreamReader isr = new InputStreamReader(fis);
BufferedReader br = new BufferedReader(isr);
StringBuilder sb = new StringBuilder();
String line;
while ((line = br.readLine()) != null) {
sb.append(line);
}
content = sb.toString();
br.close();
isr.close();
fis.close();
} catch (Exception e) {
e.printStackTrace();
}
3. Delete a File from Internal Storage:
To delete a file from internal storage, you can use the deleteFile
method:
String filename = "myfile.txt";
boolean deleted = deleteFile(filename);
if (deleted) {
// File deleted successfully
} else {
// File not found or unable to delete
}
4. Check if a File Exists:
You can check if a file exists in internal storage using the getFileStreamPath
method:
String filename = "myfile.txt";
File file = getFileStreamPath(filename);
if (file.exists()) {
// File exists
} else {
// File does not exist
}
5. File Storage Location:
Files stored in internal storage are typically located in the app’s data directory:
/data/data/com.example.yourapp/files/
Keep in mind that files in internal storage are removed when the user uninstalls the app. If you need to store data that should persist even after the app is uninstalled or if you need to share data between apps, you should consider using external storage or other data storage options provided by Android.