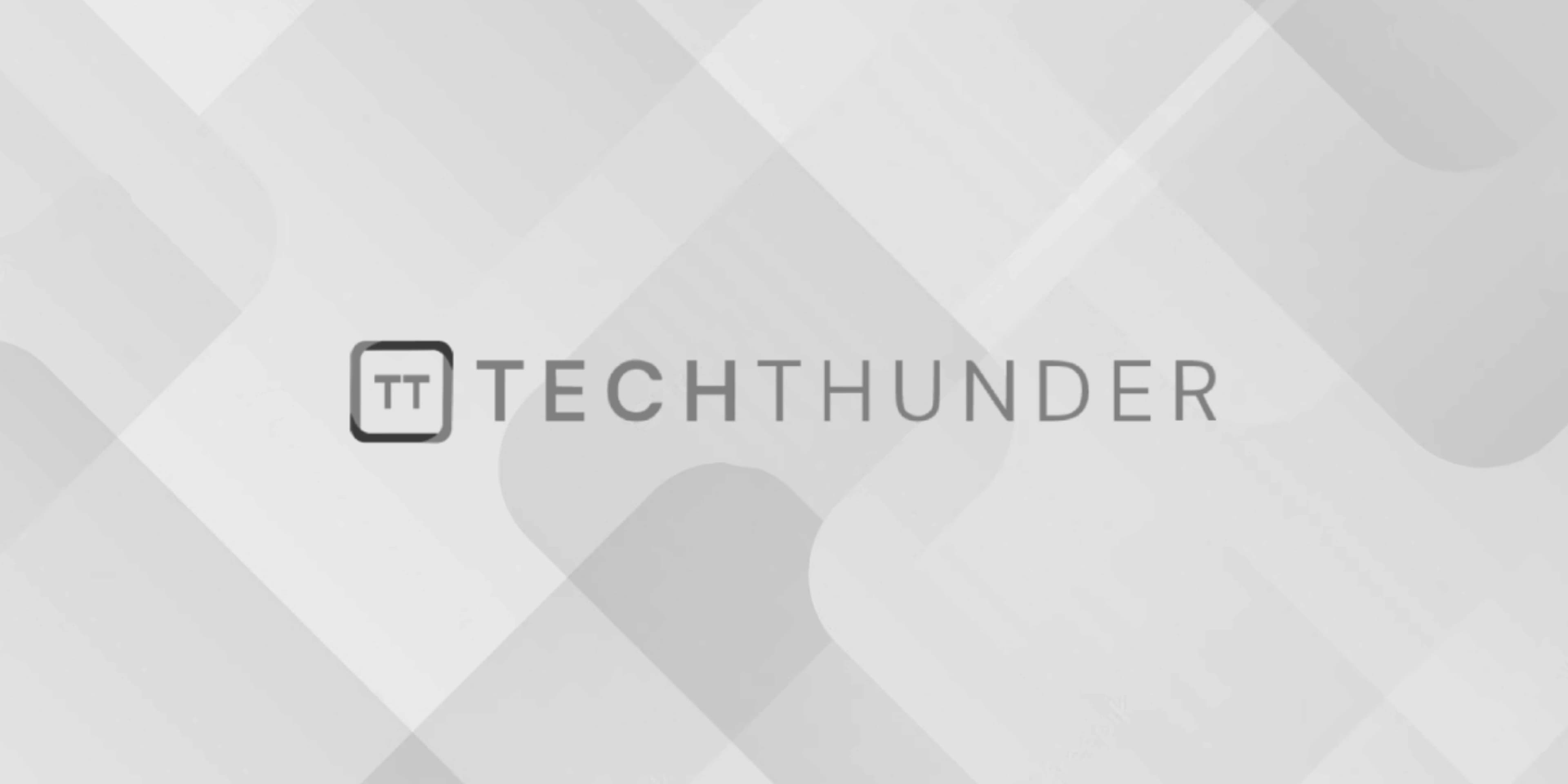
Using Google reCAPTCHA in Android
Using Google reCAPTCHA in Android is a way to protect your mobile app from automated bots and ensure the security of various actions, such as user registration, form submissions, or preventing spam. Google’s reCAPTCHA service provides a simple and effective way to integrate this security feature into your Android app. Here’s how to use Google reCAPTCHA in an Android app:
1. Sign Up for reCAPTCHA:
- Go to the Google reCAPTCHA website and sign in with your Google account.
- Click on the “+ Admin Console” button to create a new site.
- Follow the prompts to register your site and generate reCAPTCHA API keys (Site Key and Secret Key). You’ll need these keys to integrate reCAPTCHA into your Android app.
2. Add the reCAPTCHA Dependency:
- In your Android app project, open the
build.gradle
file (usually found in the app module) and add the following dependency:implementation 'com.google.android.gms:play-services-safetynet:17.0.0'
- Sync your project to download the dependency.
3. Integrate reCAPTCHA in Your App:
- In your Android app, decide where you want to add reCAPTCHA, such as on a registration or contact form.
- Add the reCAPTCHA widget to your layout XML file. For example:
<com.google.android.gms.safetynet.RecaptchaSafetyNetView android:id="@+id/recaptcha_view" android:layout_width="wrap_content" android:layout_height="wrap_content" />
4. Initialize reCAPTCHA:
- In your Java or Kotlin code, initialize the reCAPTCHA widget and configure it with your Site Key from step 1.
RecaptchaSafetyNetView recaptchaView = findViewById(R.id.recaptcha_view); recaptchaView.setSiteKey("YOUR_SITE_KEY");
5. Verify reCAPTCHA Response:
- When a user submits a form or takes an action that requires reCAPTCHA verification, you should verify the reCAPTCHA response on the server side.
- Send the reCAPTCHA response token to your server and use the reCAPTCHA API to verify it. Google provides documentation on how to verify reCAPTCHA responses on the server.
6. Handle Verification Results:
- After verifying the reCAPTCHA response on your server, you will receive a verification result. Depending on the result, you can proceed with the requested action or show an error message to the user.
7. Handle Error Cases:
- Implement error handling to deal with situations where reCAPTCHA verification fails or the user does not complete the reCAPTCHA challenge.
8. UI Customization (Optional):
- You can customize the appearance of the reCAPTCHA widget to match your app’s design by using XML attributes or programmatically setting properties.
9. Testing:
- Before deploying your app with reCAPTCHA, thoroughly test it to ensure that reCAPTCHA verification works as expected.
Remember to follow Google’s reCAPTCHA usage guidelines and adhere to their terms of service when integrating reCAPTCHA into your Android app. By implementing reCAPTCHA, you can enhance the security of your app and protect it from automated bot attacks.