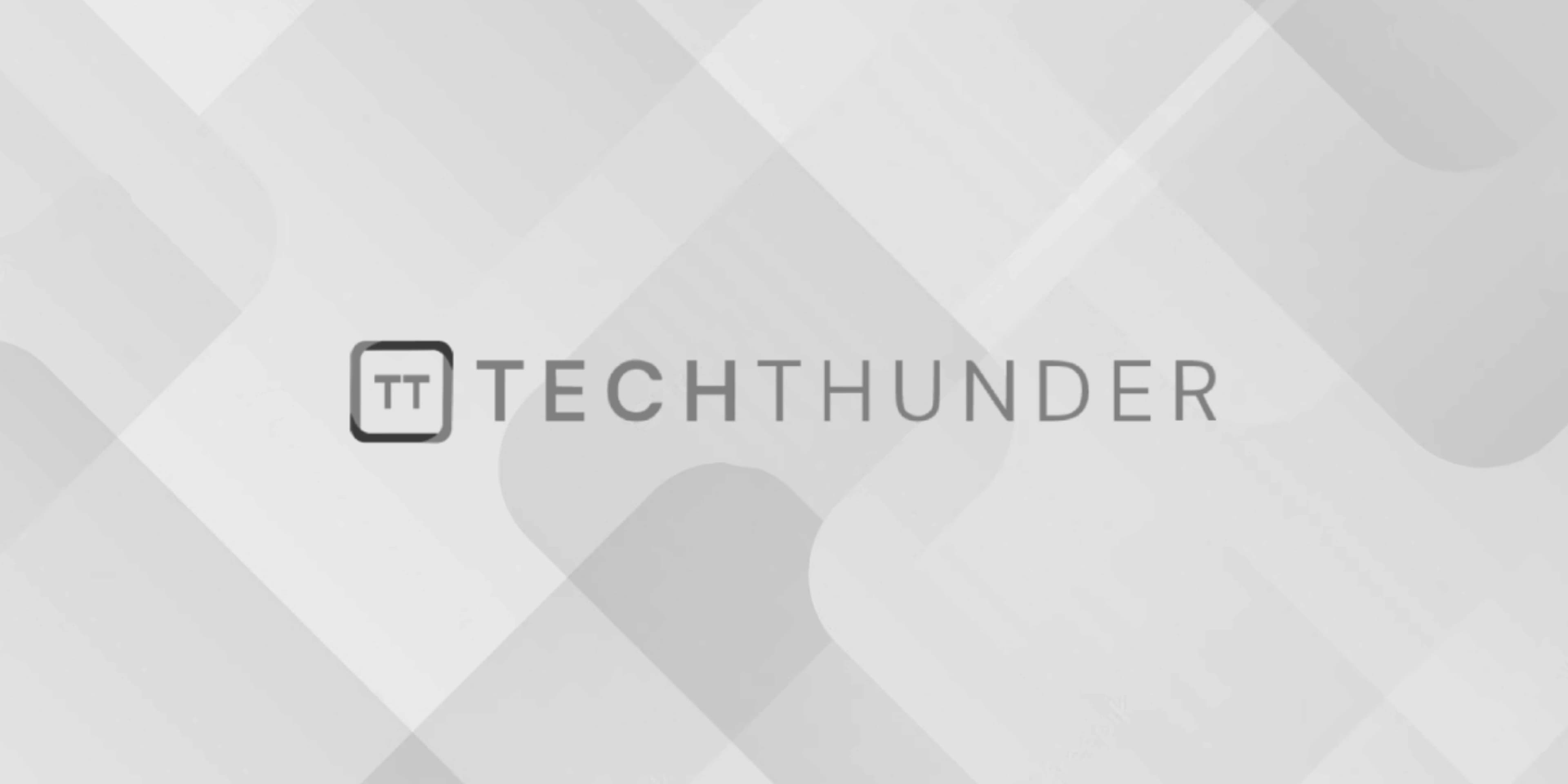
Phone Call in Android
The initiating phone calls from an Android app can be done using the ACTION_CALL
or ACTION_DIAL
intent. Here’s how you can make a phone call in Android:
1. Add the CALL_PHONE Permission:
In your AndroidManifest.xml
, add the CALL_PHONE
permission:
<uses-permission android:name="android.permission.CALL_PHONE" />
2. Check for Permission (if targeting Android 6.0 and above):
If your app targets Android 6.0 (API level 23) or higher, you need to request the CALL_PHONE
permission at runtime. Here’s how you can request it:
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.M) {
if (ContextCompat.checkSelfPermission(this, Manifest.permission.CALL_PHONE) != PackageManager.PERMISSION_GRANTED) {
ActivityCompat.requestPermissions(this, new String[]{Manifest.permission.CALL_PHONE}, REQUEST_CALL_PHONE);
return;
}
}
Make sure to handle the permission request result in the onRequestPermissionsResult
method.
3. Make the Phone Call:
You can use an Intent
to initiate a phone call. You have two options:
- ACTION_CALL: This action initiates a call directly. The user may need to confirm the call.
String phoneNumber = "1234567890"; // Replace with the phone number you want to call
Uri uri = Uri.parse("tel:" + phoneNumber);
Intent callIntent = new Intent(Intent.ACTION_CALL, uri);
if (ActivityCompat.checkSelfPermission(this, Manifest.permission.CALL_PHONE) == PackageManager.PERMISSION_GRANTED) {
startActivity(callIntent);
}
- ACTION_DIAL: This action opens the dialer with the phone number pre-filled, allowing the user to initiate the call.
String phoneNumber = "1234567890"; // Replace with the phone number you want to call
Uri uri = Uri.parse("tel:" + phoneNumber);
Intent dialIntent = new Intent(Intent.ACTION_DIAL, uri);
startActivity(dialIntent);
4. Handle Permission Result (if requested):
If you requested the CALL_PHONE
permission at runtime, handle the permission result in the onRequestPermissionsResult
method:
@Override
public void onRequestPermissionsResult(int requestCode, String[] permissions, int[] grantResults) {
if (requestCode == REQUEST_CALL_PHONE) {
if (grantResults.length > 0 && grantResults[0] == PackageManager.PERMISSION_GRANTED) {
// Permission granted, you can make the phone call now
} else {
// Permission denied, handle accordingly
Toast.makeText(this, "Permission denied. Cannot make a call.", Toast.LENGTH_SHORT).show();
}
}
}
5. Testing:
Test your app on a physical Android device to ensure that it can make phone calls as intended.
Remember to handle permission requests and the user’s choice to ensure that your app works correctly on different Android versions. Additionally, be cautious when initiating phone calls programmatically to avoid unintentional dialing.