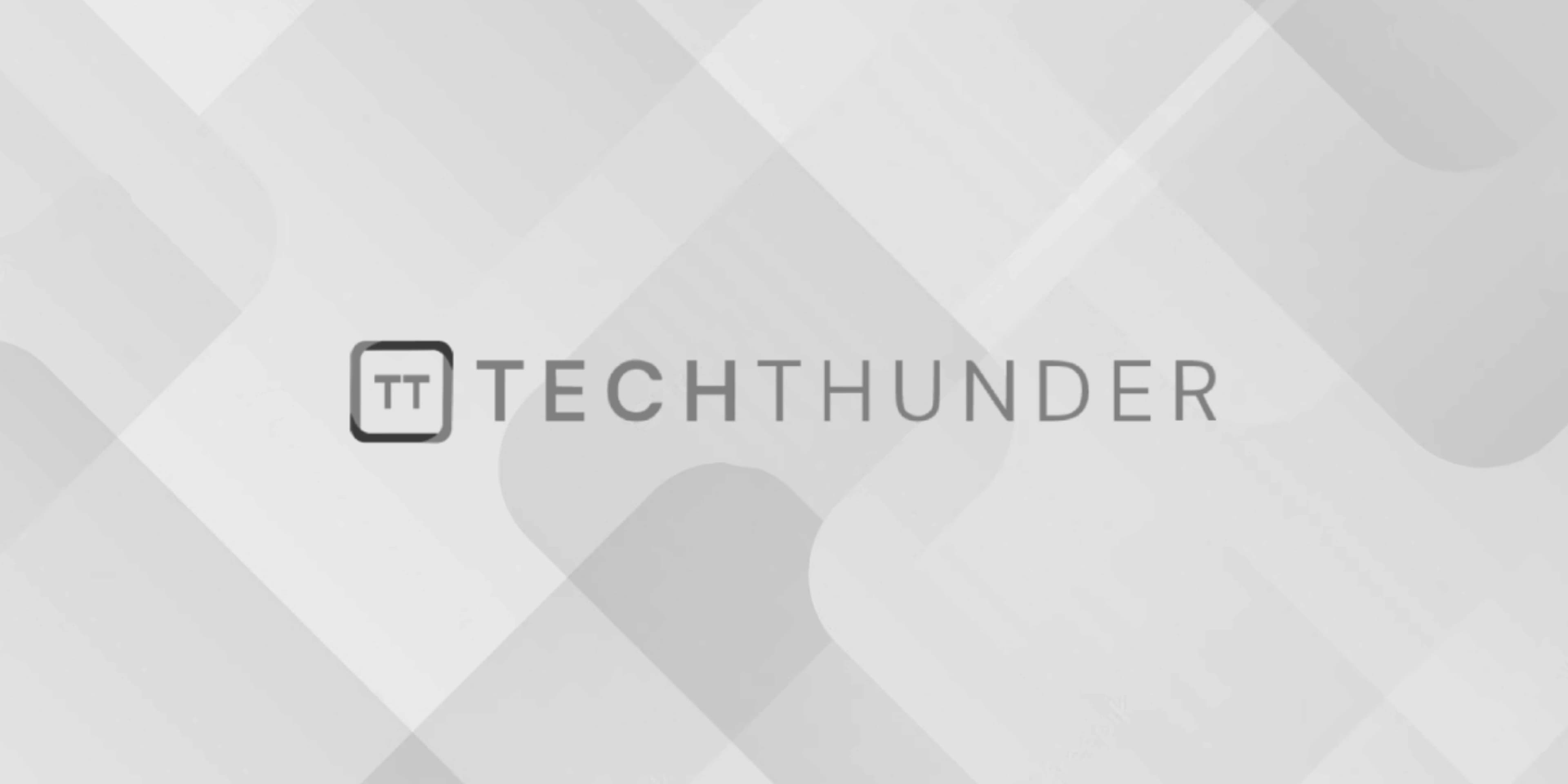
Screen Orientation in Android
Screen orientation in Android refers to the direction in which the screen of a mobile device is currently positioned relative to the device’s physical orientation. Android provides support for different screen orientations, primarily portrait and landscape, to allow your app’s user interface to adapt to the way the user is holding the device. Here’s how you can work with screen orientations in Android:
Portrait Orientation:
- In portrait orientation, the screen is taller than it is wide.
- This is the default orientation for most Android devices, and it’s often used for activities like reading or making phone calls.
Landscape Orientation:
- In landscape orientation, the screen is wider than it is tall.
- Landscape orientation is useful for activities like watching videos, playing games, or using apps that benefit from a wider display.
Auto-Rotation:
- By default, most Android devices support auto-rotation, which means the screen will automatically switch between portrait and landscape orientations based on how the user holds the device.
- Users can enable or disable auto-rotation in their device’s settings.
Controlling Screen Orientation in Android:
To control screen orientation in your Android app, you have several options:
Manifest File:
- You can set the preferred screen orientation for an activity in the app’s AndroidManifest.xml file using the
android:screenOrientation
attribute. - For example, to lock an activity in portrait mode, you can use:
<activity android:name=".YourActivity" android:screenOrientation="portrait">
- To lock an activity in landscape mode:
<activity android:name=".YourActivity" android:screenOrientation="landscape">
Programmatic Control:
- You can also control screen orientation programmatically in your activity’s Java or Kotlin code.
- To lock the screen to portrait mode programmatically, use:
setRequestedOrientation(ActivityInfo.SCREEN_ORIENTATION_PORTRAIT);
- To lock the screen to landscape mode programmatically, use:
setRequestedOrientation(ActivityInfo.SCREEN_ORIENTATION_LANDSCAPE);
- To allow auto-rotation, use:
setRequestedOrientation(ActivityInfo.SCREEN_ORIENTATION_SENSOR);
Handling Orientation Changes:
- By default, when the screen orientation changes (e.g., from portrait to landscape or vice versa), Android may destroy and recreate your activity.
- You can handle orientation changes by saving and restoring your activity’s state using methods like
onSaveInstanceState
andonRestoreInstanceState
.
Handling Configuration Changes:
- You can also handle configuration changes (including screen orientation changes) by specifying in your manifest that you want to handle certain configuration changes yourself. This is done using the
android:configChanges
attribute:<activity android:name=".YourActivity" android:configChanges="orientation|screenSize">
- Then, override the
onConfigurationChanged
method in your activity to handle the changes.
Controlling screen orientation is important to ensure that your app’s user interface remains usable and visually appealing regardless of how the user holds the device. Depending on your app’s requirements, you can choose the approach that best suits your needs, whether it’s locking the orientation, allowing auto-rotation, or handling orientation changes gracefully.