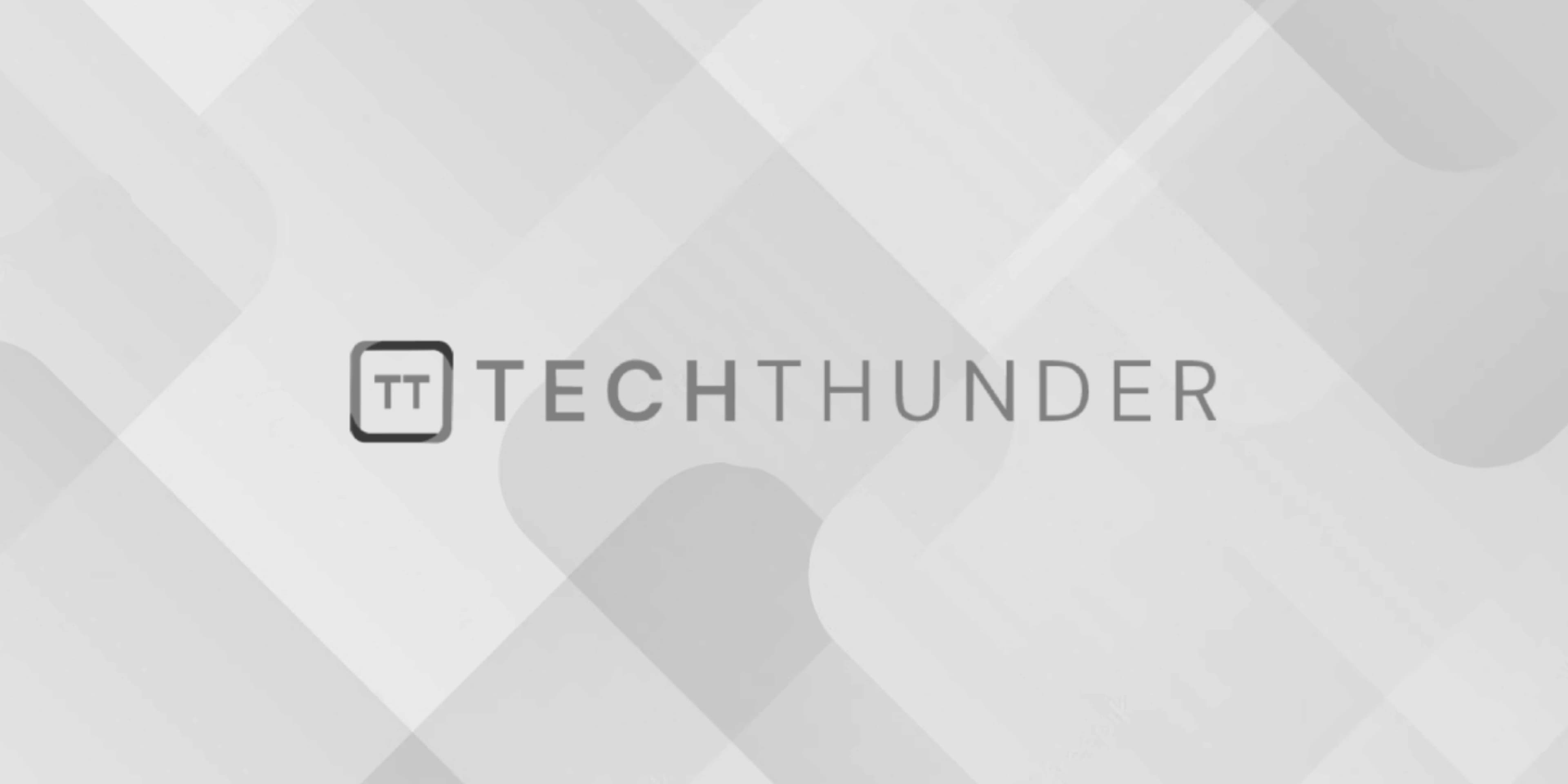
ToggleButton in Android
The Android ToggleButton
is a user interface component that allows users to toggle between two states, typically on and off. It is visually represented as a button that can be pressed to change its state. You can use a ToggleButton
to collect binary choices or to allow users to enable or disable a feature or setting. Here’s how to use a ToggleButton
in Android:
Add the ToggleButton
to Your Layout: In your XML layout file, add a ToggleButton
element where you want to include the binary choice functionality:
<ToggleButton
android:id="@+id/toggle_button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textOn="ON" // Text when the button is in the 'on' state
android:textOff="OFF" // Text when the button is in the 'off' state
android:layout_gravity="center"
/>
android:id
: Assign a unique ID to theToggleButton
for later reference.android:layout_width
andandroid:layout_height
: Adjust these attributes based on your layout requirements.android:textOn
andandroid:textOff
: Specify the text that appears on the button when it’s in the ‘on’ and ‘off’ states, respectively.
Access the ToggleButton
in Your Activity or Fragment: In your Java or Kotlin code (usually within your activity or fragment), obtain a reference to the ToggleButton
using its ID:
ToggleButton toggleButton = findViewById(R.id.toggle_button);
Handle State Changes: To respond to changes in the toggle state made by the user, you can set an OnCheckedChangeListener
:
toggleButton.setOnCheckedChangeListener(new CompoundButton.OnCheckedChangeListener() {
@Override
public void onCheckedChanged(CompoundButton buttonView, boolean isChecked) {
// Handle the toggle state change here
// 'isChecked' is true if the button is in the 'on' state, false if 'off'
}
});
The onCheckedChanged
method is called when the user toggles the ToggleButton
. You can perform actions based on the isChecked
value.
Run Your App: When you run your app, the ToggleButton
will be displayed with the specified text labels. Users can tap on it to toggle its state between ‘on’ and ‘off’. The onCheckedChanged
method will be called when the user changes the state.
Customization (Optional): You can customize the appearance and behavior of the ToggleButton
by setting various attributes in XML or programmatically. For example, you can set the initial state, change the text labels, and more.
<!-- Customizing the ToggleButton -->
<ToggleButton
android:id="@+id/toggle_button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textOn="Enabled"
android:textOff="Disabled"
android:checked="true" // Set the initial state
android:textSize="18sp"
android:textColor="@color/toggle_button_text_color"
android:backgroundTint="@color/toggle_button_background_color"
android:layout_gravity="center"
/>
You can customize these attributes to match your app’s design and requirements.
You can easily implement a ToggleButton
in your Android app to collect binary choice input from users and respond to state changes as needed.