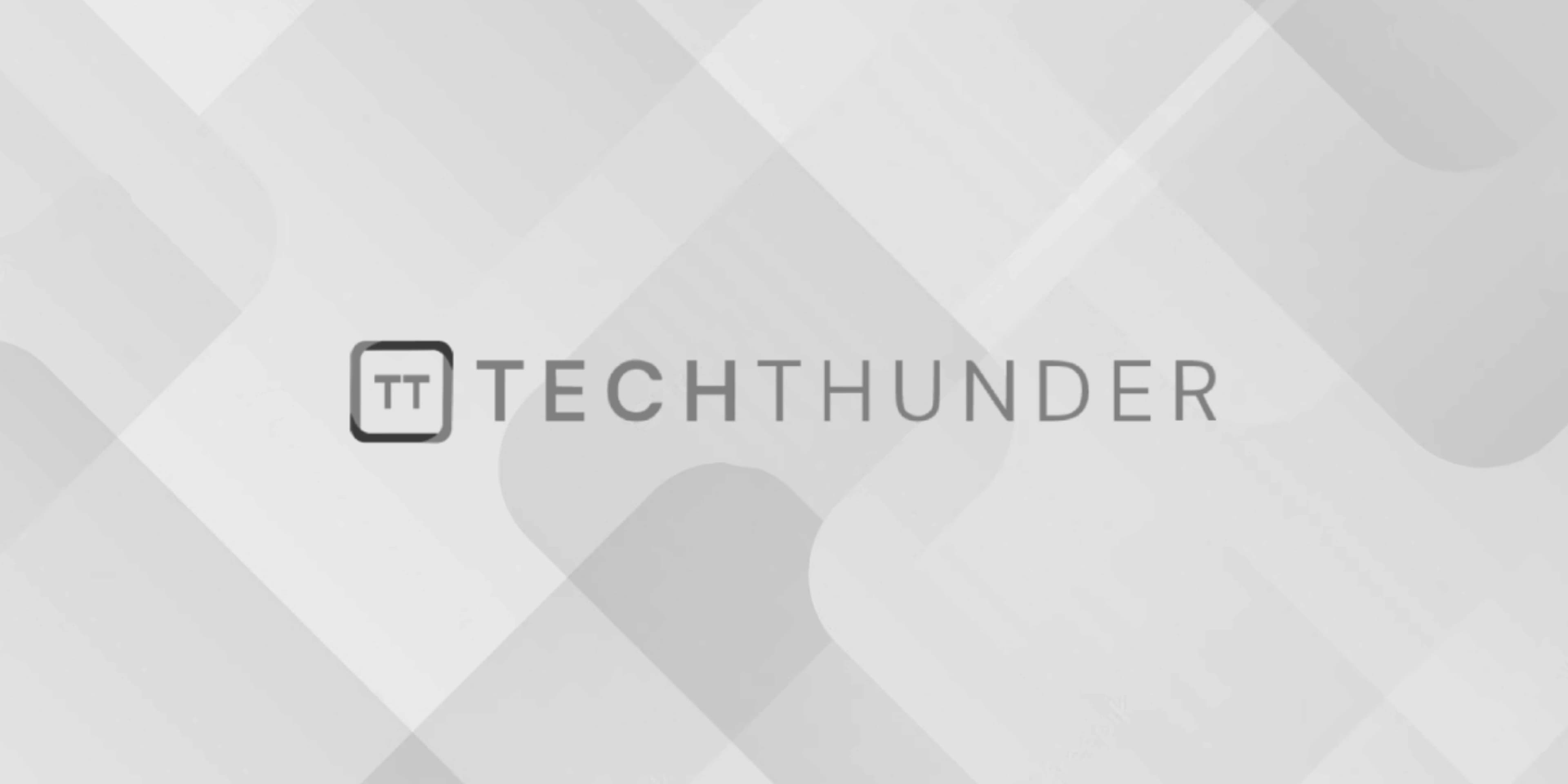
Android Google Admob
AdMob is Google’s advertising platform for mobile apps. It allows app developers to monetize their apps by displaying ads from various advertisers. AdMob provides a way to earn revenue through in-app advertising and is widely used by Android app developers. Here’s a high-level overview of how to integrate AdMob into your Android app:
1. Set Up an AdMob Account:
Before you can start using AdMob in your Android app, you need to sign up for an AdMob account. You can do this by visiting the AdMob website, and signing in with your Google account. Follow the steps to create your AdMob account.
2. Create an AdMob App:
After setting up your AdMob account, you’ll need to create an AdMob app for your Android application. In the AdMob dashboard, click “Apps” and then “Add App.” Follow the prompts to add your app and provide necessary details.
3. Add Ad Units:
Ad units are the placeholders where ads will be displayed within your app. You can create various types of ad units, including banner ads, interstitial ads, and rewarded ads. To create ad units, go to the “Ad units” section in your AdMob dashboard and follow the instructions to create the ad units you need for your app.
4. Integrate the AdMob SDK:
To show ads in your Android app, you need to integrate the AdMob SDK (Software Development Kit) into your project. Here are the steps:
- Open your app’s
build.gradle
file (usually located in the “app” module) and add the AdMob dependency:implementation 'com.google.android.gms:play-services-ads:20.2.0'
- Sync your project with Gradle to download the dependency.
5. Initialize AdMob in Your App:
In your app’s code, typically in your main activity or application class, initialize the AdMob SDK by calling MobileAds.initialize()
with your AdMob App ID. The App ID can be found in your AdMob dashboard.
import com.google.android.gms.ads.MobileAds;
public class MyApplication extends Application {
@Override
public void onCreate() {
super.onCreate();
// Initialize the AdMob SDK
MobileAds.initialize(this, "YOUR_ADMOB_APP_ID");
}
}
6. Display Ads in Your App:
Now that you’ve integrated AdMob, you can display ads in your app by adding ad views to your layout XML files or programmatically creating ad views. The specific implementation will depend on the type of ad unit you want to use.
- Banner Ads: To display banner ads, add an
AdView
widget to your layout XML file. Make sure to specify your ad unit ID:
<com.google.android.gms.ads.AdView
android:id="@+id/adView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
ads:adSize="BANNER"
ads:adUnitId="YOUR_BANNER_AD_UNIT_ID"
/>
- In your code, load and display the ad:
AdView adView = findViewById(R.id.adView);
AdRequest adRequest = new AdRequest.Builder().build();
adView.loadAd(adRequest);
- Interstitial Ads: Interstitial ads are full-screen ads that can be displayed at natural breaks in your app’s flow, such as between levels in a game. To display interstitial ads, create an
InterstitialAd
object and load and show the ad when appropriate. - Rewarded Ads: Rewarded ads are used to reward users for watching a video ad. You can implement rewarded ads to incentivize users to engage with ads in your app.
7. Test Ads During Development:
During development and testing, you should use test ads to avoid generating invalid traffic. Test ads are provided by AdMob and won’t impact your account’s ad serving performance.
8. AdMob Policies and Compliance:
Ensure that your app complies with AdMob policies and guidelines. AdMob has strict policies regarding ad content, placement, and user experience. Violating these policies can result in ad serving limitations or account suspension.
9. Monitor Ad Performance:
Use the AdMob dashboard to monitor the performance of your ads, including ad impressions, clicks, and revenue. This information can help you optimize your ad strategy and maximize your earnings.
Integrating AdMob into your Android app can provide a source of revenue if you have a substantial user base. However, it’s important to strike a balance between monetization and user experience to ensure that ads do not negatively impact your app’s usability and appeal to users.