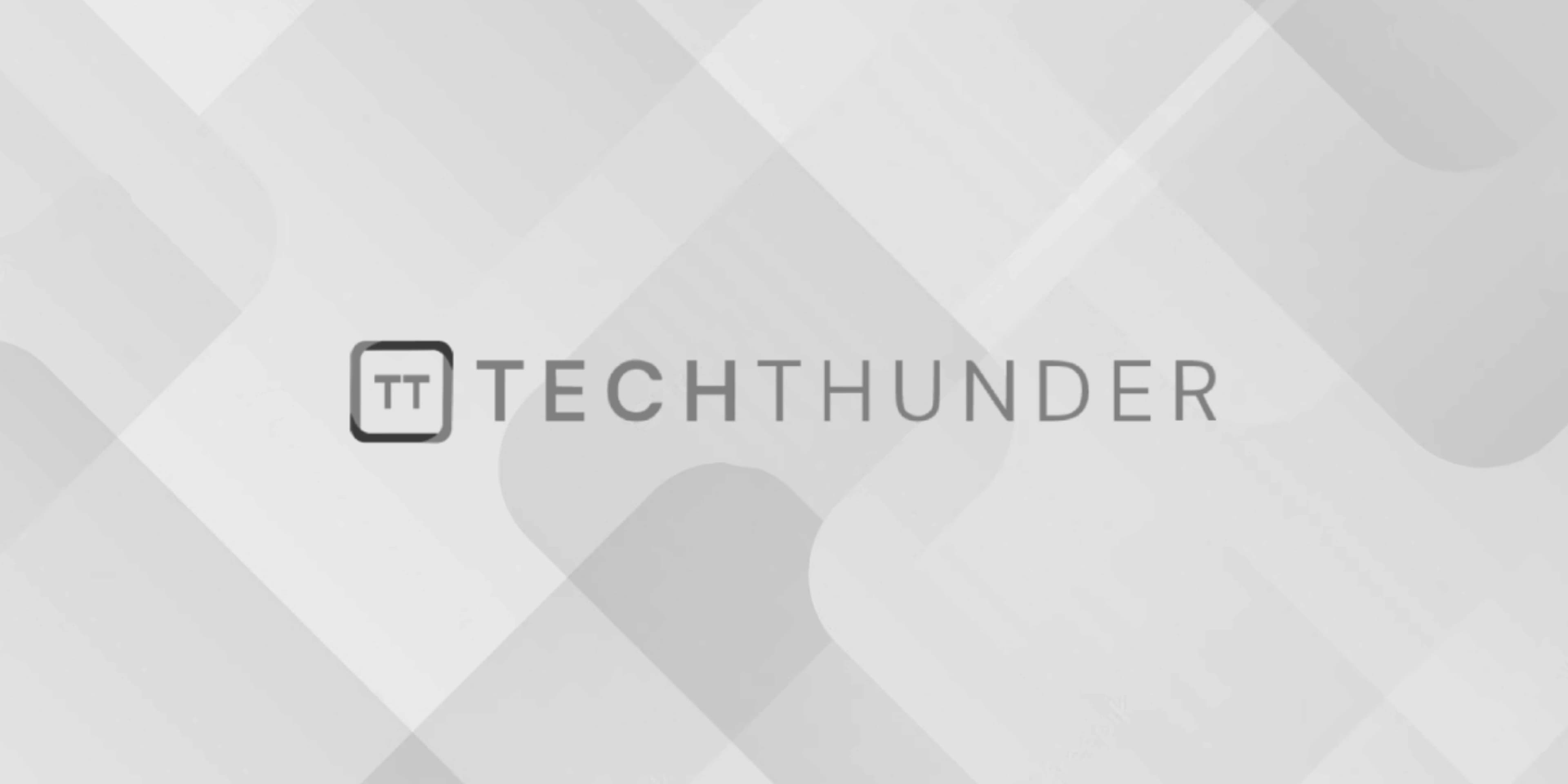
WebView in Android
A WebView
in Android is a user interface component that allows you to display web content within your app. You can use it to load and render web pages, HTML content, or even display web-based applications within your Android application. Here’s how to use a WebView
in Android:
Add the WebView
to Your Layout: In your XML layout file, add a WebView
element where you want to display the web content:
<WebView
android:id="@+id/webview"
android:layout_width="match_parent"
android:layout_height="match_parent"
/>
This sets up the WebView
to occupy the entire available space.
Load Web Content: In your Java or Kotlin code (usually within your activity or fragment), obtain a reference to the WebView
using its ID:
WebView webView = findViewById(R.id.webview);
You can then load web content into the WebView
. Here are a few examples:
- Load a Web Page:
webView.loadUrl("https://www.example.com");
- Load HTML Content:
String htmlContent = "<html>
<body>
<h1>Hello, WebView!</h1>
</body>
</html>";
webView.loadData(htmlContent, "text/html", "UTF-8");
- Load Web Content from a File:
webView.loadUrl("file:///android_asset/my_webpage.html");
Make sure the HTML file is located in theassets
folder of your app.
- WebView Settings (Optional): You can configure various settings for the
WebView
, such as enabling JavaScript, handling redirects, and setting up a customWebViewClient
for handling page navigation events. Here’s an example of enabling JavaScript:
WebSettings webSettings = webView.getSettings();
webSettings.setJavaScriptEnabled(true);
Enabling JavaScript allows web pages that rely on JavaScript to function properly within the WebView
.
Handle WebView Navigation: You can set up a WebViewClient
to handle navigation events within the WebView
. This allows you to intercept and handle URLs or control the behavior of navigation within your app. For example:
webView.setWebViewClient(new WebViewClient() {
@Override
public boolean shouldOverrideUrlLoading(WebView view, String url) {
// Handle URL loading within the WebView
view.loadUrl(url);
return true; // Return true to indicate that the URL has been handled
}
});
This code ensures that URLs are loaded within the WebView
instead of launching an external browser.
Run Your App: When you run your app, the WebView
will display the specified web content. Users can interact with the web content as if they were using a web browser.
Permissions (if required): Depending on your app’s requirements, you may need to add permissions to your AndroidManifest.xml file. For example, if your web content requires internet access, add the following permission:
<uses-permission android:name="android.permission.INTERNET" />
Make sure to request any required permissions from the user at runtime if your target Android version requires it.
You can easily incorporate a WebView
into your Android app to display web content or web-based applications. Customizing the WebView
settings and handling navigation events allows you to create a seamless web experience within your app.