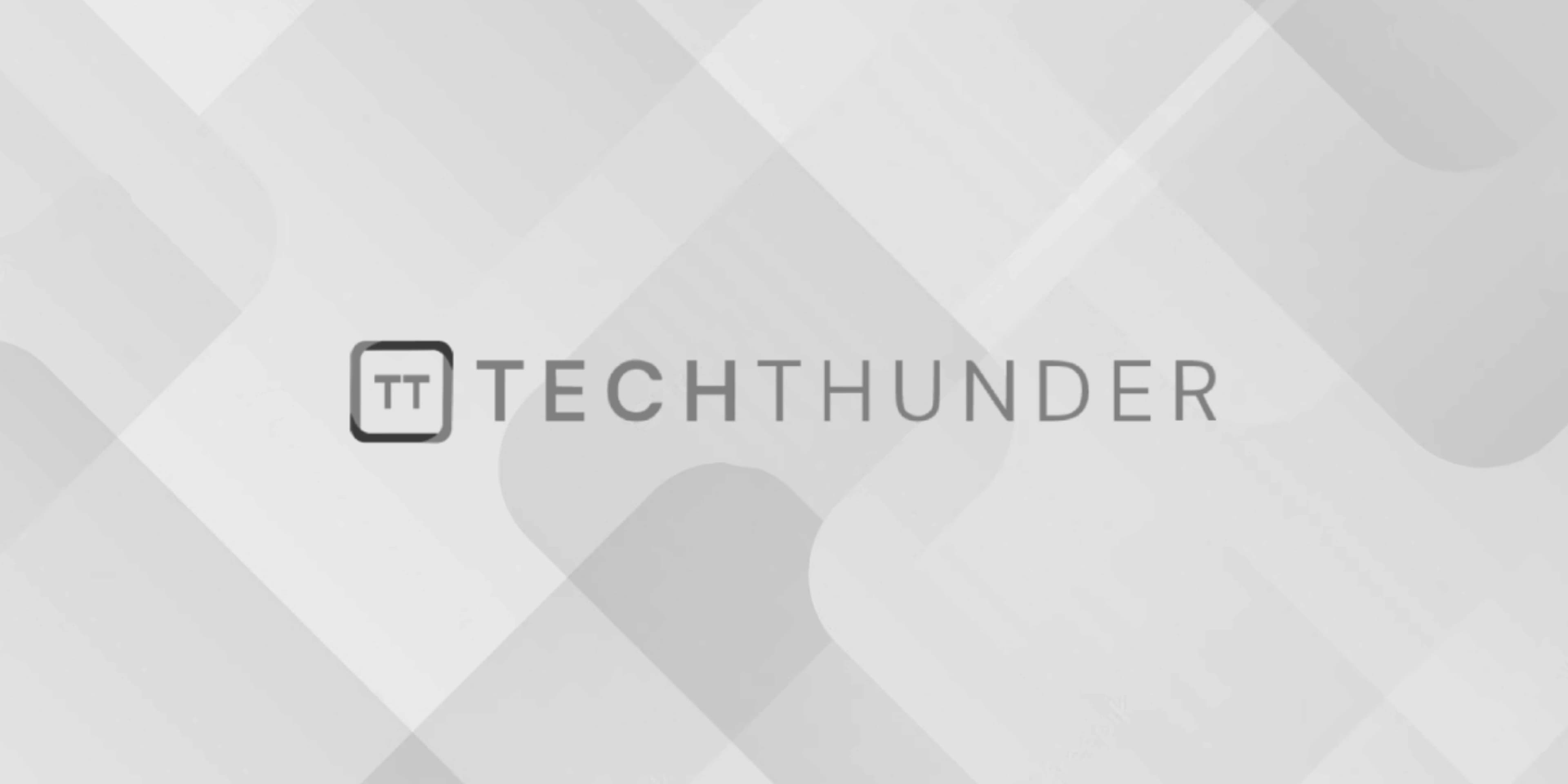
Banner Ads2 in Android
Integrating banner ads in an Android app is a common way to monetize your app’s user base. Banner ads are smaller ads that are typically displayed at the top or bottom of the screen. In this guide, I’ll walk you through the steps to integrate banner ads into your Android app using Google AdMob.
1. Set Up an AdMob Account:
If you haven’t already, create an AdMob account (part of Google AdSense) at AdMob. Once your account is set up, create an AdMob ad unit for banner ads.
2. Add the AdMob SDK to Your Project:
Open your app’s build.gradle
file (usually located in the “app” module) and add the AdMob dependency:
implementation 'com.google.android.gms:play-services-ads:20.2.0'
Sync your project to download the dependency.
3. Initialize AdMob in Your App:
In your app’s Application
class or the onCreate
method of your main activity, initialize the AdMob SDK with your AdMob app ID:
import com.google.android.gms.ads.MobileAds;
public class MyApplication extends Application {
@Override
public void onCreate() {
super.onCreate();
// Initialize AdMob
MobileAds.initialize(this, "YOUR_ADMOB_APP_ID");
}
}
Replace "YOUR_ADMOB_APP_ID"
with your actual AdMob app ID.
4. Add Banner Ad to Your Layout:
In your layout XML file where you want to display the banner ad, add a com.google.android.gms.ads.AdView
element:
<com.google.android.gms.ads.AdView
android:id="@+id/adView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="bottom|center"
ads:adSize="BANNER"
ads:adUnitId="YOUR_BANNER_AD_UNIT_ID" />
Replace "YOUR_BANNER_AD_UNIT_ID"
with the actual ad unit ID you created in AdMob.
5. Load Banner Ad in Your Activity:
In your activity, you need to load the banner ad by finding the AdView
and loading it with an ad request:
import com.google.android.gms.ads.AdRequest;
import com.google.android.gms.ads.AdView;
public class YourActivity extends AppCompatActivity {
private AdView adView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_your);
// Find the AdView by its ID
adView = findViewById(R.id.adView);
// Load the ad with an ad request
AdRequest adRequest = new AdRequest.Builder().build();
adView.loadAd(adRequest);
}
@Override
protected void onDestroy() {
// Destroy the AdView when your activity is destroyed to free up resources
if (adView != null) {
adView.destroy();
}
super.onDestroy();
}
}
6. Test with Test Ads:
During development and testing, it’s important to use test ads to avoid generating invalid traffic. AdMob provides test ad unit IDs that you can use for testing. To enable test ads, use a test device or an emulator. Be sure to remove test ads before publishing your app.
7. Publish Your App:
Before publishing your app with banner ads, ensure that it complies with AdMob policies and guidelines. AdMob has strict policies regarding ad content, placement, and user experience.
That’s it! You’ve successfully integrated banner ads into your Android app using AdMob. Users will see banner ads at the designated location in your app, helping you monetize your app’s user base.