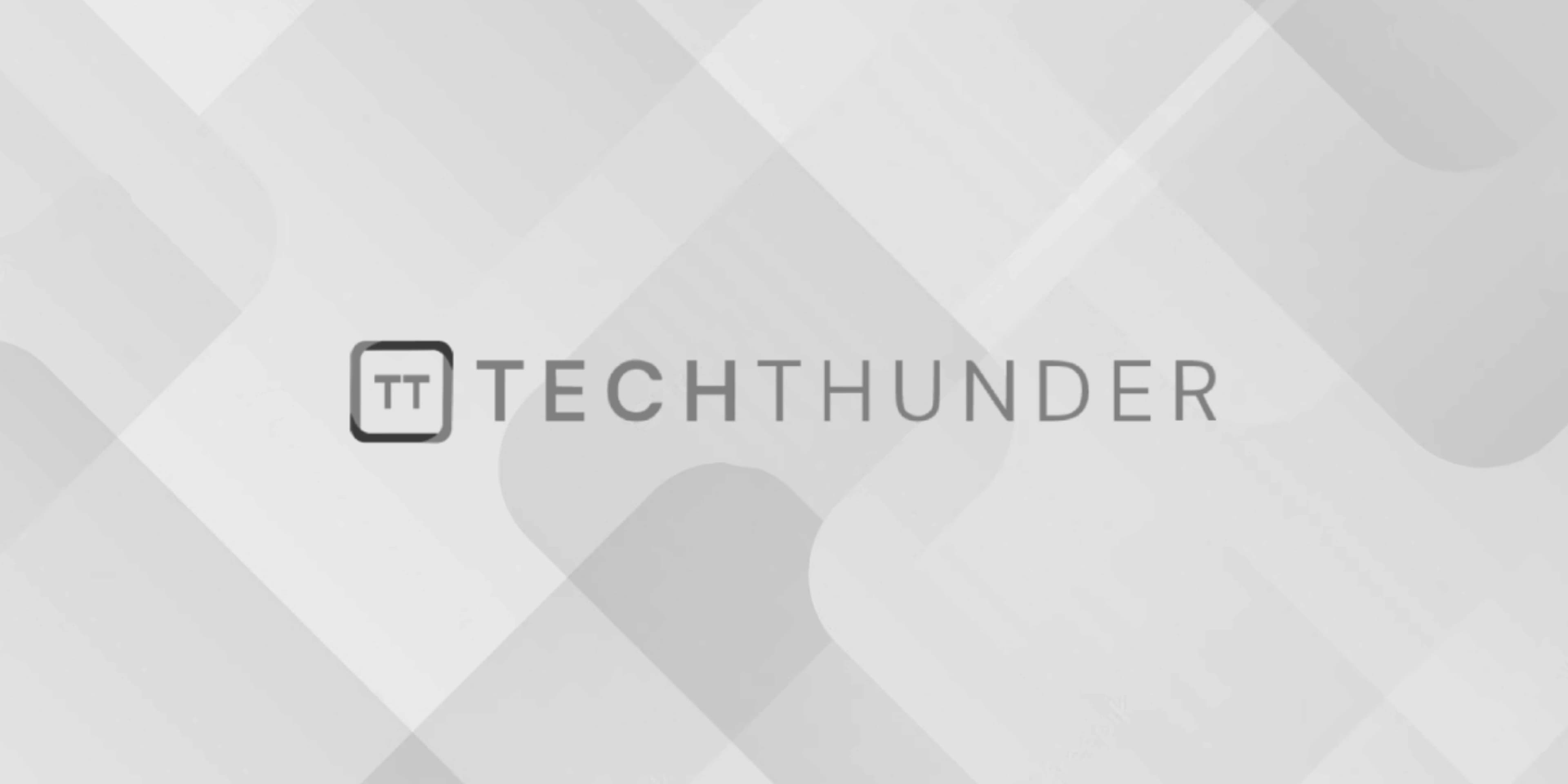
Custom CheckBox in Android
To create a custom CheckBox
in Android, you can define a custom XML layout for the checkbox and then use that layout to create instances of the custom CheckBox
in your code. Here’s how you can do it:
Create a Custom Layout for the CheckBox: In your project’s res/layout
directory, create an XML layout file for your custom CheckBox
. For example, let’s create a file named custom_checkbox.xml
:
<!-- res/layout/custom_checkbox.xml -->
<CheckBox
xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/custom_checkbox"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:button="@drawable/custom_checkbox_selector"
android:text="Custom CheckBox"
android:textSize="16sp"
android:paddingStart="8dp"
android:paddingEnd="8dp"
android:layout_gravity="center_vertical"
android:gravity="center_vertical"
android:drawablePadding="8dp"
/>
In this example, we define a custom layout for the CheckBox
. We set attributes like android:button
to a custom drawable for the checkbox’s appearance, and android:text
for the checkbox label. You can customize these attributes as needed.
Create a Custom Drawable for the Checkbox: In your project’s res/drawable
directory, create a custom XML drawable for the checkbox appearance. Let’s create a file named custom_checkbox_selector.xml
:
<!-- res/drawable/custom_checkbox_selector.xml -->
<selector xmlns:android="http://schemas.android.com/apk/res/android">
<!-- Checked state -->
<item android:drawable="@drawable/ic_checkbox_checked" android:state_checked="true" />
<!-- Unchecked state -->
<item android:drawable="@drawable/ic_checkbox_unchecked" />
</selector>
This XML defines a selector that changes the appearance of the CheckBox
based on its checked state. It uses two custom drawables, ic_checkbox_checked
and ic_checkbox_unchecked
, which should be created as PNG or vector drawables.
Create Custom Checkbox Drawables: In your res/drawable
directory, create the custom checkbox drawables (ic_checkbox_checked
and ic_checkbox_unchecked
). These should be PNG or vector drawable files representing the checkbox in its checked and unchecked states. Here’s an example of a simple checkbox icon:
ic_checkbox_checked.xml
(for the checked state):
<!-- res/drawable/ic_checkbox_checked.xml -->
<vector xmlns:android="http://schemas.android.com/apk/res/android"
android:width="24dp"
android:height="24dp"
android:viewportWidth="24.0"
android:viewportHeight="24.0">
<!-- Customize the fill color -->
<path
android:fillColor="#FF0000" // Customize the fill color
android:pathData="M12,2C6.48,2 2,6.48 2,12s4.48,10 10,10 10-4.48 10-10S17.52,2 12,2zm-1,14-4-4 1.41-1.41L11,14.17l6.59-6.59L18,9z" />
</vector>
ic_checkbox_unchecked.xml
(for the unchecked state):
<!-- res/drawable/ic_checkbox_unchecked.xml -->
<vector xmlns:android="http://schemas.android.com/apk/res/android"
android:width="24dp"
android:height="24dp"
android:viewportWidth="24.0"
android:viewportHeight="24.0">
<!-- Customize the fill color -->
<path
android:fillColor="#000000" // Customize the fill color
android:pathData="M19,5v14H5V5h14m0-2H5c-1.11,0-2,0.89-2,2v14c0,1.11,0.89,2,2,2h14c1.11,0,2-0.89,2-2V5c0-1.11-0.89-2-2-2z" />
</vector>
Use the Custom CheckBox in Your Layout: In your main layout XML file, include the custom CheckBox
:
<!-- Include the custom CheckBox -->
<include layout="@layout/custom_checkbox" />
Access the Custom CheckBox in Code: In your Java or Kotlin code (usually within your activity or fragment), obtain a reference to the custom CheckBox
:
CheckBox customCheckBox = findViewById(R.id.custom_checkbox);
You can now use the customCheckBox
object as you would with any other CheckBox
to set its state or handle click events.
With these steps, you’ve created and used a custom CheckBox
in your Android app, allowing you to customize its appearance to fit your design requirements.