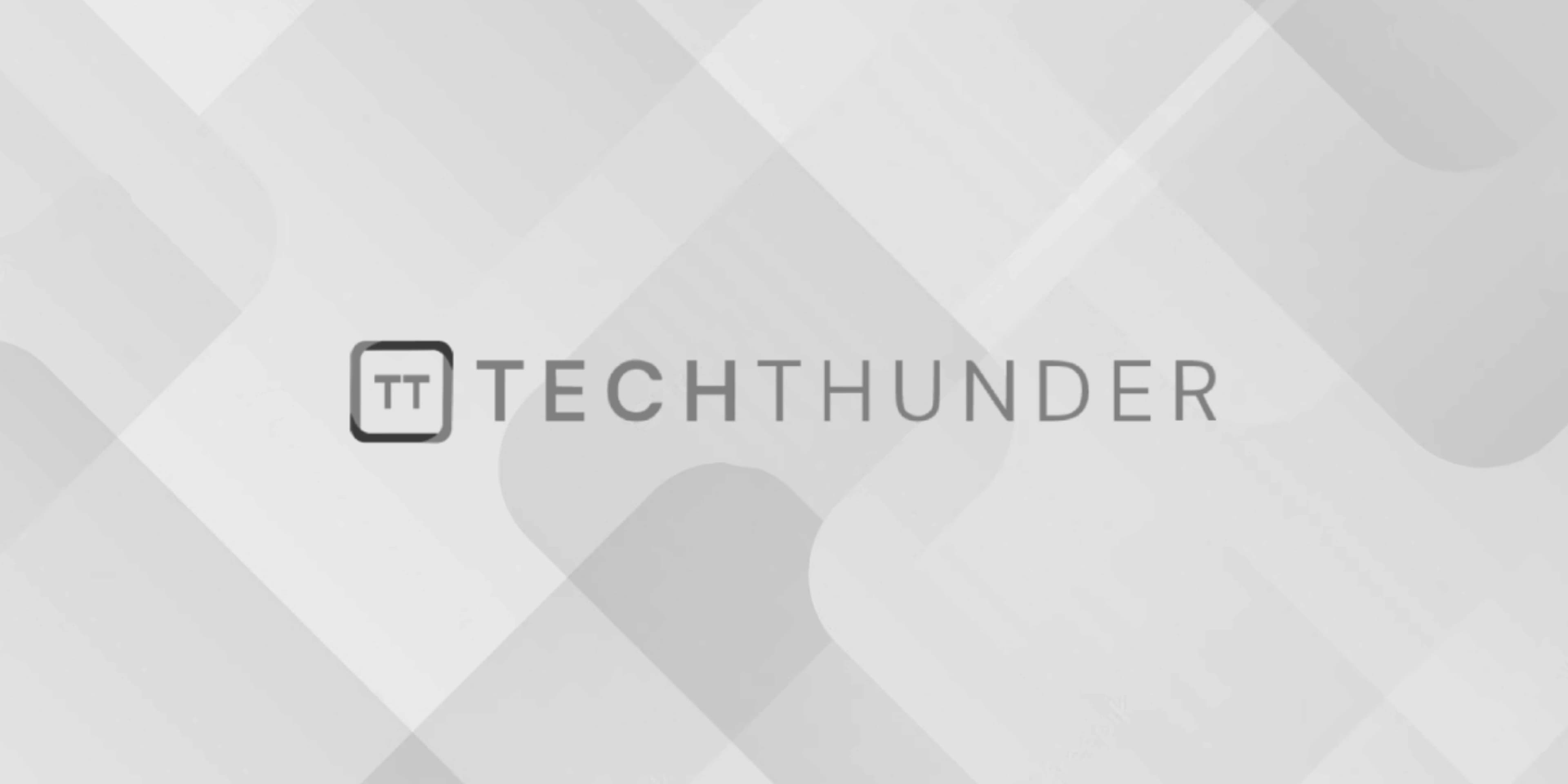
TelephonyManager in Android
The TelephonyManager
class in Android is a system service that provides access to information about the telephony services on the device, including information about the SIM card, network state, and call state. You can use it to perform tasks related to telephony services, such as getting device information, monitoring network and call state changes, and accessing SIM card details. Here are some common use cases for TelephonyManager
:
1. Getting Device Information:
You can use TelephonyManager
to retrieve information about the device’s telephony capabilities, such as the device’s IMEI (International Mobile Equipment Identity) number, IMSI (International Mobile Subscriber Identity) number, and phone type (GSM or CDMA).
TelephonyManager telephonyManager = (TelephonyManager) getSystemService(Context.TELEPHONY_SERVICE);
// Get the IMEI number
String imei = telephonyManager.getImei();
// Get the IMSI number
String imsi = telephonyManager.getSubscriberId();
// Get the phone type (GSM or CDMA)
int phoneType = telephonyManager.getPhoneType();
2. Monitoring Network State:
You can use TelephonyManager
to monitor changes in the network state, such as when the device switches between different network types (e.g., from 3G to 4G) or when it loses or gains network connectivity.
TelephonyManager telephonyManager = (TelephonyManager) getSystemService(Context.TELEPHONY_SERVICE);
// Set up a PhoneStateListener to listen for network state changes
PhoneStateListener phoneStateListener = new PhoneStateListener() {
@Override
public void onDataConnectionStateChanged(int state) {
// Handle network state changes
}
};
telephonyManager.listen(phoneStateListener, PhoneStateListener.LISTEN_DATA_CONNECTION_STATE);
3. Monitoring Call State:
You can use TelephonyManager
to monitor changes in the call state, such as when a call is incoming, outgoing, or idle. This is useful for implementing call-related features in your app.
TelephonyManager telephonyManager = (TelephonyManager) getSystemService(Context.TELEPHONY_SERVICE);
// Set up a PhoneStateListener to listen for call state changes
PhoneStateListener phoneStateListener = new PhoneStateListener() {
@Override
public void onCallStateChanged(int state, String phoneNumber) {
// Handle call state changes
}
};
telephonyManager.listen(phoneStateListener, PhoneStateListener.LISTEN_CALL_STATE);
4. Accessing SIM Card Information:
You can use TelephonyManager
to access information about the SIM card, such as the SIM serial number, carrier name, and country code.
TelephonyManager telephonyManager = (TelephonyManager) getSystemService(Context.TELEPHONY_SERVICE);
// Get the SIM serial number
String simSerialNumber = telephonyManager.getSimSerialNumber();
// Get the carrier name
String carrierName = telephonyManager.getNetworkOperatorName();
// Get the country code
String countryCode = telephonyManager.getSimCountryIso();
5. Check Network Roaming Status:
You can use TelephonyManager
to check whether the device is currently roaming on a different network.
TelephonyManager telephonyManager = (TelephonyManager) getSystemService(Context.TELEPHONY_SERVICE);
boolean isRoaming = telephonyManager.isNetworkRoaming();
Keep in mind that you should request the necessary permissions, such as READ_PHONE_STATE
and READ_SMS
, to use some of the functionalities provided by TelephonyManager
. Additionally, you should handle permission checks and runtime permissions in your app to ensure compatibility with Android’s permission model.