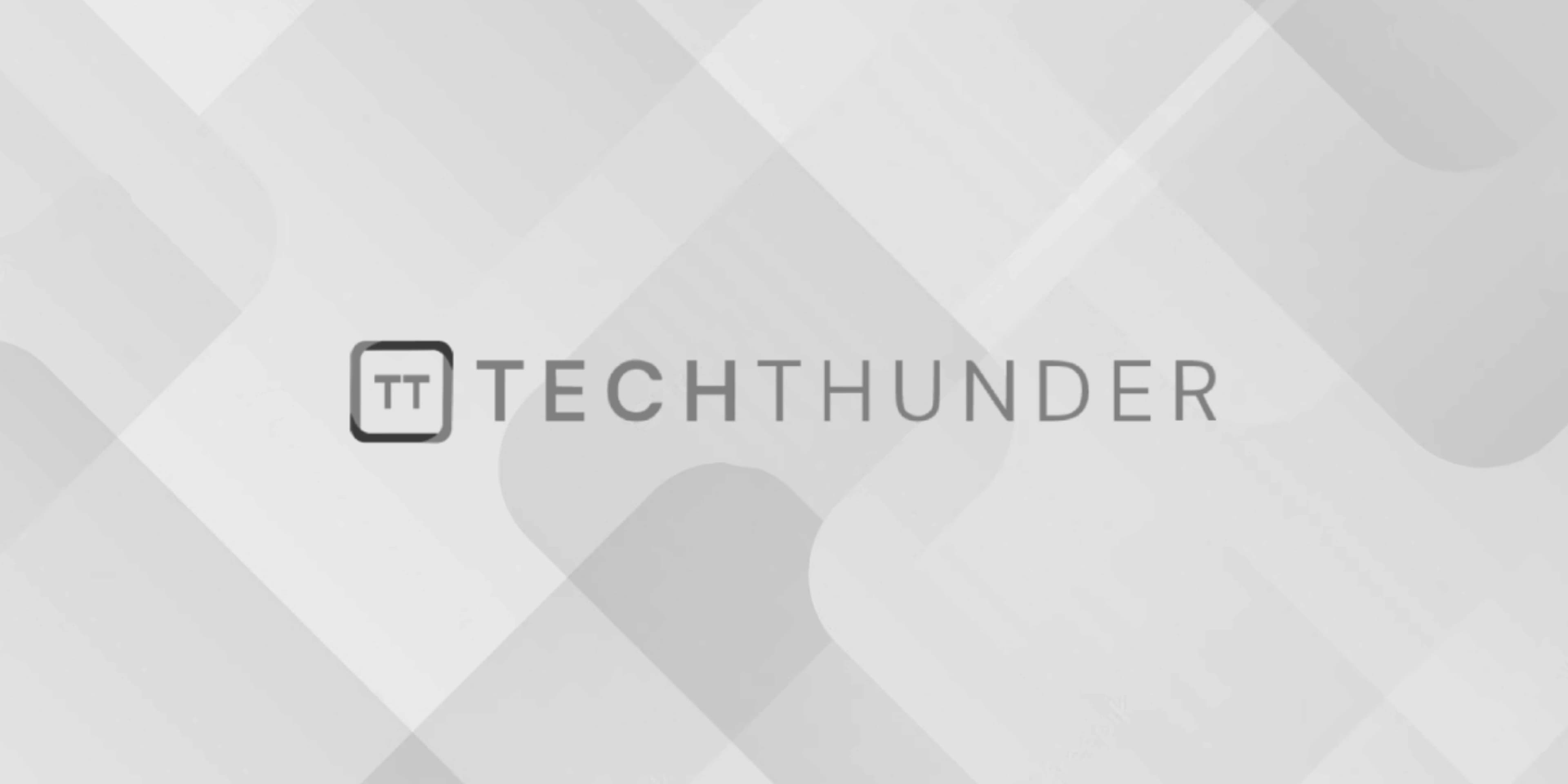
217 views
SeekBar in Android
The Android SeekBar
is a user interface element that allows users to adjust a numeric value within a specified range by dragging a thumb along a horizontal or vertical track. It is often used for settings that involve selecting values like volume, brightness, or progress in media playback.
Here’s how you can implement a SeekBar
in Android:
- Add a SeekBar to Your Layout: In your XML layout file, add a
SeekBar
element where you want to include the SeekBar:
XML
<SeekBar
android:id="@+id/seek_bar"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_margin="16dp"
android:max="100" // Maximum value for the SeekBar
android:progress="50" // Initial progress value
android:thumb="@drawable/custom_thumb" // Optional custom thumb drawable
android:thumbOffset="0dp" // Optional thumb offset
android:layout_gravity="center_vertical"
/>
android:id
: Assign a unique ID to the SeekBar for later reference.android:layout_width
andandroid:layout_height
: Adjust these attributes according to your layout requirements.android:max
: Set the maximum value for the SeekBar.android:progress
: Set the initial progress value.android:thumb
: Optionally, you can specify a custom drawable for the thumb (the draggable part of the SeekBar).android:thumbOffset
: Optionally, you can set an offset for the thumb drawable.android:layout_gravity
: Adjust the gravity to position the SeekBar within its parent.
- Access the SeekBar in Your Activity or Fragment: In your Java or Kotlin code (usually within your activity or fragment), you need to obtain a reference to the
SeekBar
using its ID:
Java
SeekBar seekBar = findViewById(R.id.seek_bar);
- Set a SeekBarChangeListener: To respond to changes in the SeekBar’s progress, you can set a
SeekBar.OnSeekBarChangeListener
. This listener provides callbacks for tracking the SeekBar’s progress as the user interacts with it.
Java
seekBar.setOnSeekBarChangeListener(new SeekBar.OnSeekBarChangeListener() {
@Override
public void onProgressChanged(SeekBar seekBar, int progress, boolean fromUser) {
// Called when the SeekBar's progress changes.
// The 'progress' parameter contains the current progress value.
// 'fromUser' indicates whether the change was initiated by the user.
// You can respond to the progress change here.
}
@Override
public void onStartTrackingTouch(SeekBar seekBar) {
// Called when the user starts touching the SeekBar.
}
@Override
public void onStopTrackingTouch(SeekBar seekBar) {
// Called when the user stops touching the SeekBar.
}
});
In the onProgressChanged
method, you can respond to progress changes and perform actions based on the SeekBar’s current value. For example, you can update the volume, adjust brightness, or control media playback.
- Handle SeekBar Events: Inside the
onProgressChanged
method, you can access the current progress value using theprogress
parameter. You can use this value to perform actions based on the SeekBar’s position. For example:
Java
@Override
public void onProgressChanged(SeekBar seekBar, int progress, boolean fromUser) {
// Respond to the SeekBar's progress change
if (fromUser) {
// The progress change was initiated by the user
// Update the volume, brightness, or perform other actions
}
}
- Run Your App: When you run your app, you will see the SeekBar element on the screen. Users can drag the thumb along the track to select a value within the specified range, and your code can respond to the changes in progress as needed.
By following these steps, you can easily implement a SeekBar
in your Android app to allow users to adjust numeric values interactively.