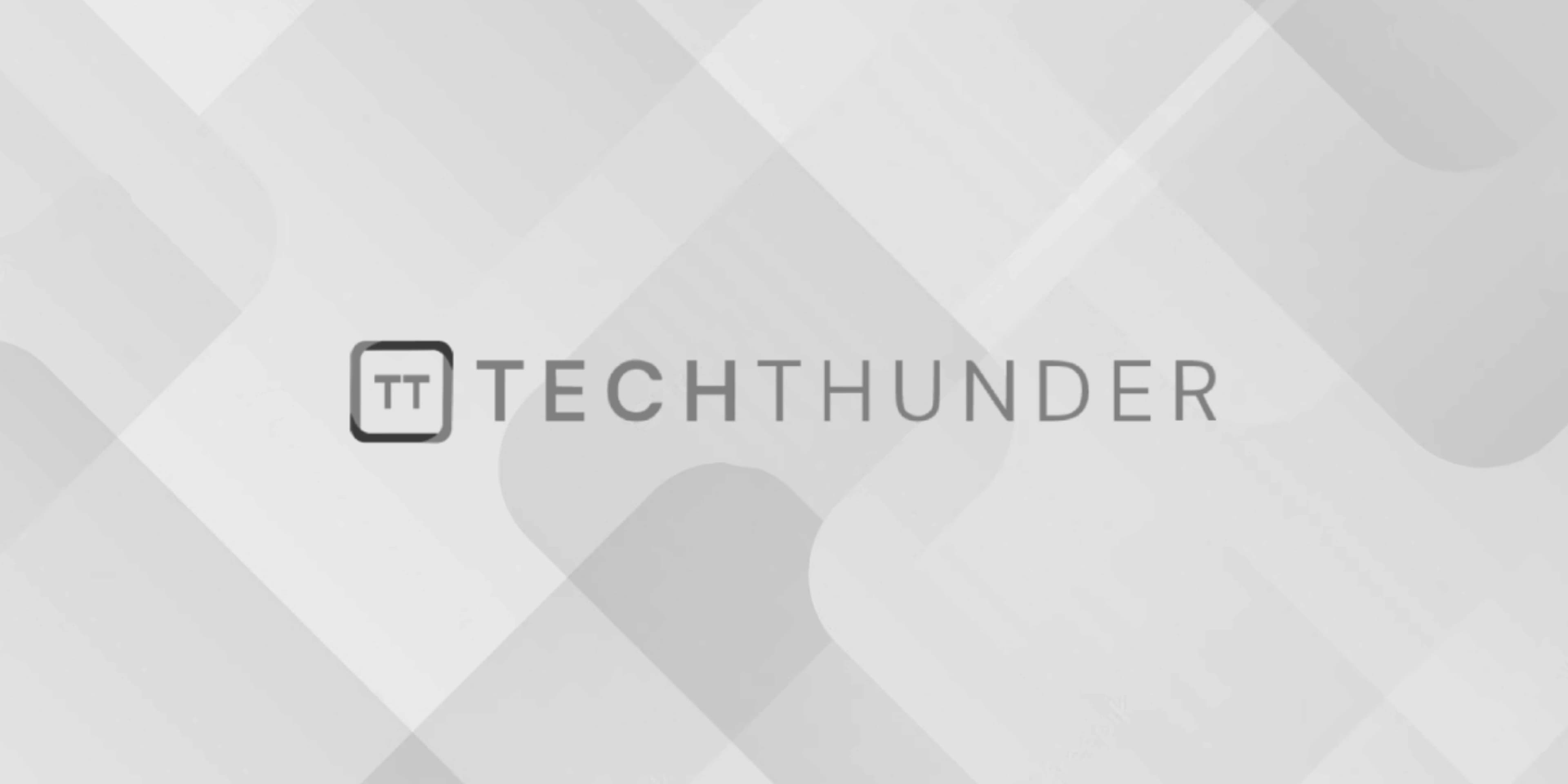
TabLayout in Android
The Android TabLayout
is a part of the Android Design Support Library and is used to implement tabbed layouts in your app’s user interface. Tabs are commonly used to organize content into distinct sections, making it easy for users to switch between different views or categories within an activity.
Here’s how you can use TabLayout
in Android:
- Include the Design Support Library: To use
TabLayout
, you need to include the Android Design Support Library in your app’s dependencies. Add the following dependency to your app’sbuild.gradle
file:
implementation 'com.google.android.material:material:1.4.0' // Use the latest version available
Sync your project with Gradle after adding the dependency.
- XML Layout: In your XML layout file, you can include a
TabLayout
along with aViewPager
to display the content associated with each tab. Here’s an example layout:
<RelativeLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<com.google.android.material.tabs.TabLayout
android:id="@+id/tab_layout"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_alignParentTop="true"
app:tabMode="fixed" />
<androidx.viewpager.widget.ViewPager
android:id="@+id/view_pager"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_below="@id/tab_layout" />
</RelativeLayout>
In this layout, the TabLayout
is positioned at the top, and a ViewPager
is used to display the content associated with each tab below it.
- Create Fragments: You’ll need to create separate fragments for each tab’s content. These fragments represent the different views or sections that users can switch between.
- Java Code: In your activity, you need to set up the
TabLayout
andViewPager
and connect them. Here’s a basic example:
// Find the TabLayout and ViewPager by their IDs
TabLayout tabLayout = findViewById(R.id.tab_layout);
ViewPager viewPager = findViewById(R.id.view_pager);
// Create an instance of the adapter that will manage the fragments
MyPagerAdapter pagerAdapter = new MyPagerAdapter(getSupportFragmentManager());
// Set the adapter to the ViewPager
viewPager.setAdapter(pagerAdapter);
// Connect the TabLayout with the ViewPager
tabLayout.setupWithViewPager(viewPager);
In this code, MyPagerAdapter
is a custom adapter that you’ll need to create to manage the fragments associated with each tab. You should also set up the titles for the tabs using tabLayout.addTab()
if needed.
- Customize and Add Content: Customize your
MyPagerAdapter
to return the appropriate fragment for each tab. You can also customize the appearance and behavior of your tabs by setting attributes and listeners on theTabLayout
andViewPager
as needed. - Handle Tab Selection: If you want to perform actions when a tab is selected, you can add an
OnTabSelectedListener
to theTabLayout
. This allows you to respond to tab selection events and update the content accordingly.
tabLayout.addOnTabSelectedListener(new TabLayout.OnTabSelectedListener() {
@Override
public void onTabSelected(TabLayout.Tab tab) {
// Handle tab selection
}
@Override
public void onTabUnselected(TabLayout.Tab tab) {
// Handle tab unselection
}
@Override
public void onTabReselected(TabLayout.Tab tab) {
// Handle tab reselection (e.g., scroll to top)
}
});
With these steps, you can create a tabbed layout in your Android app using TabLayout
and ViewPager
. Users can switch between different sections or views by tapping on the tabs, making it a convenient way to organize and present content in your app.