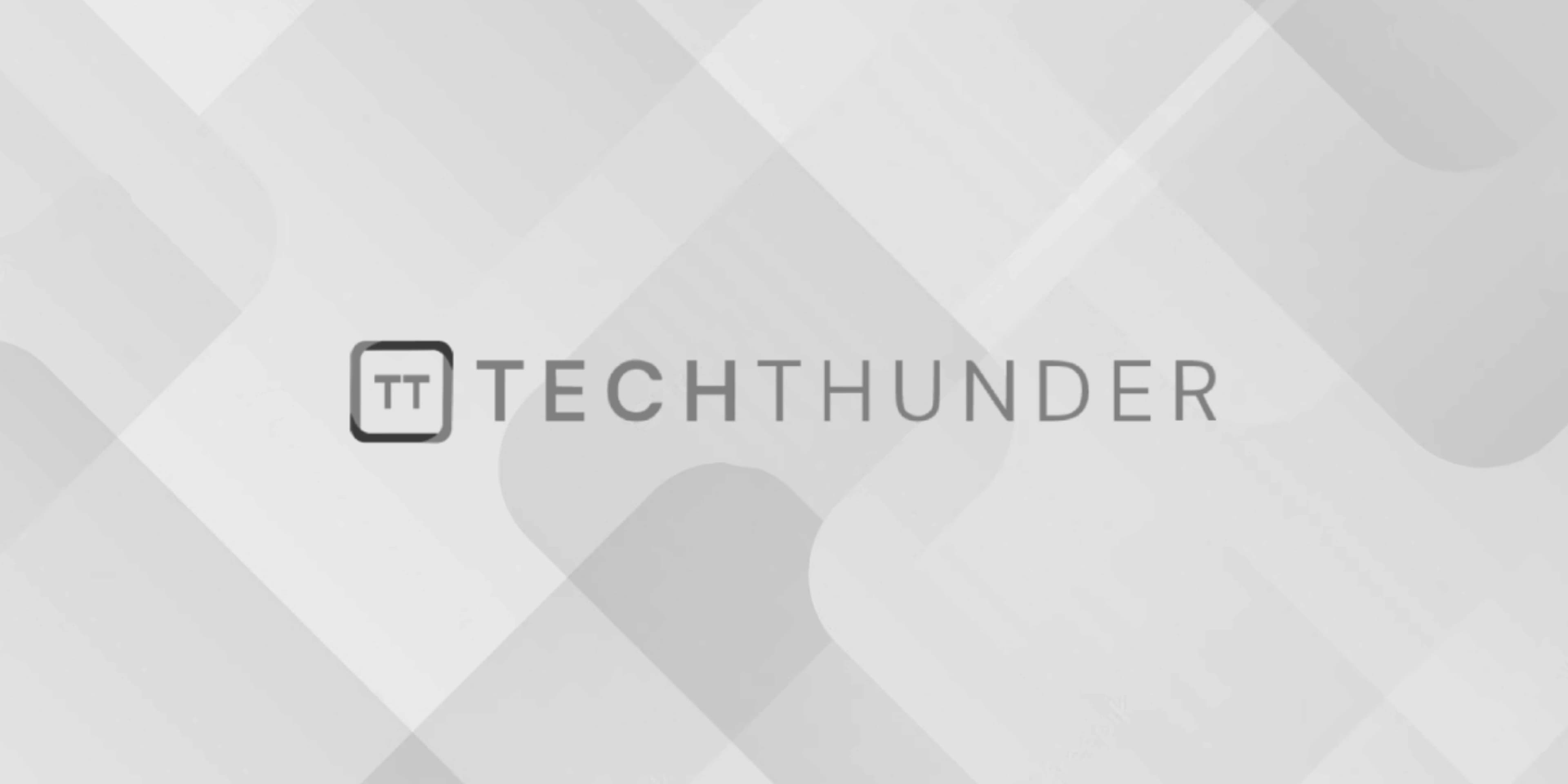
Current Location in Android
Obtaining the current location in an Android app typically involves using the device’s GPS, network, or fused location services. To get the current location, follow these steps:
1. Add Location Permissions to AndroidManifest.xml:
In your app’s AndroidManifest.xml file, add the necessary location permissions:
<uses-permission android:name="android.permission.ACCESS_FINE_LOCATION" />
<uses-permission android:name="android.permission.ACCESS_COARSE_LOCATION" />
You’ll need ACCESS_FINE_LOCATION
for precise GPS-based location and ACCESS_COARSE_LOCATION
for network-based location.
2. Check and Request Permissions at Runtime (For Android 6.0+):
If your app targets Android 6.0 (API level 23) or higher, you need to request location permissions at runtime. You can use the following code snippet to check and request permissions:
if (ContextCompat.checkSelfPermission(this, Manifest.permission.ACCESS_FINE_LOCATION) != PackageManager.PERMISSION_GRANTED) {
// Request the permission.
ActivityCompat.requestPermissions(this, new String[]{Manifest.permission.ACCESS_FINE_LOCATION}, REQUEST_LOCATION_PERMISSION);
} else {
// Permission is already granted; proceed with location access.
}
Don’t forget to handle the permission results in the onRequestPermissionsResult
callback.
3. Create a Location Request:
To retrieve the current location, create a LocationRequest
object to specify the desired update interval and accuracy:
LocationRequest locationRequest = LocationRequest.create()
.setPriority(LocationRequest.PRIORITY_HIGH_ACCURACY)
.setInterval(10000) // 10 seconds
.setFastestInterval(5000); // 5 seconds
4. Implement a Location Callback:
You’ll need to implement a LocationCallback
to handle location updates:
private final LocationCallback locationCallback = new LocationCallback() {
@Override
public void onLocationResult(LocationResult locationResult) {
if (locationResult == null) {
return;
}
Location location = locationResult.getLastLocation();
// Use the location data here.
}
};
5. Request Location Updates:
Use the FusedLocationProviderClient
to request location updates:
FusedLocationProviderClient fusedLocationClient = LocationServices.getFusedLocationProviderClient(this);
fusedLocationClient.requestLocationUpdates(locationRequest, locationCallback, null);
6. Handle Location Updates and Errors:
In the onLocationResult
callback, you can handle location updates and any errors that may occur during location retrieval.
7. Test on Emulators and Devices:
Test your location-related code on physical devices and emulators with various Android versions and settings to ensure compatibility and accuracy.
8. Privacy Considerations:
Respect user privacy and obtain user consent before collecting and using location data. Follow privacy best practices and comply with data protection regulations.
9. Handle Stopping Location Updates:
To save battery life and data usage, remember to stop location updates when they are no longer needed. You can do this by calling fusedLocationClient.removeLocationUpdates(locationCallback)
.
The location retrieval might take some time, especially when using GPS. Also, location accuracy may vary depending on the device’s capabilities and environmental factors. Ensure that your app gracefully handles location data availability and accuracy.