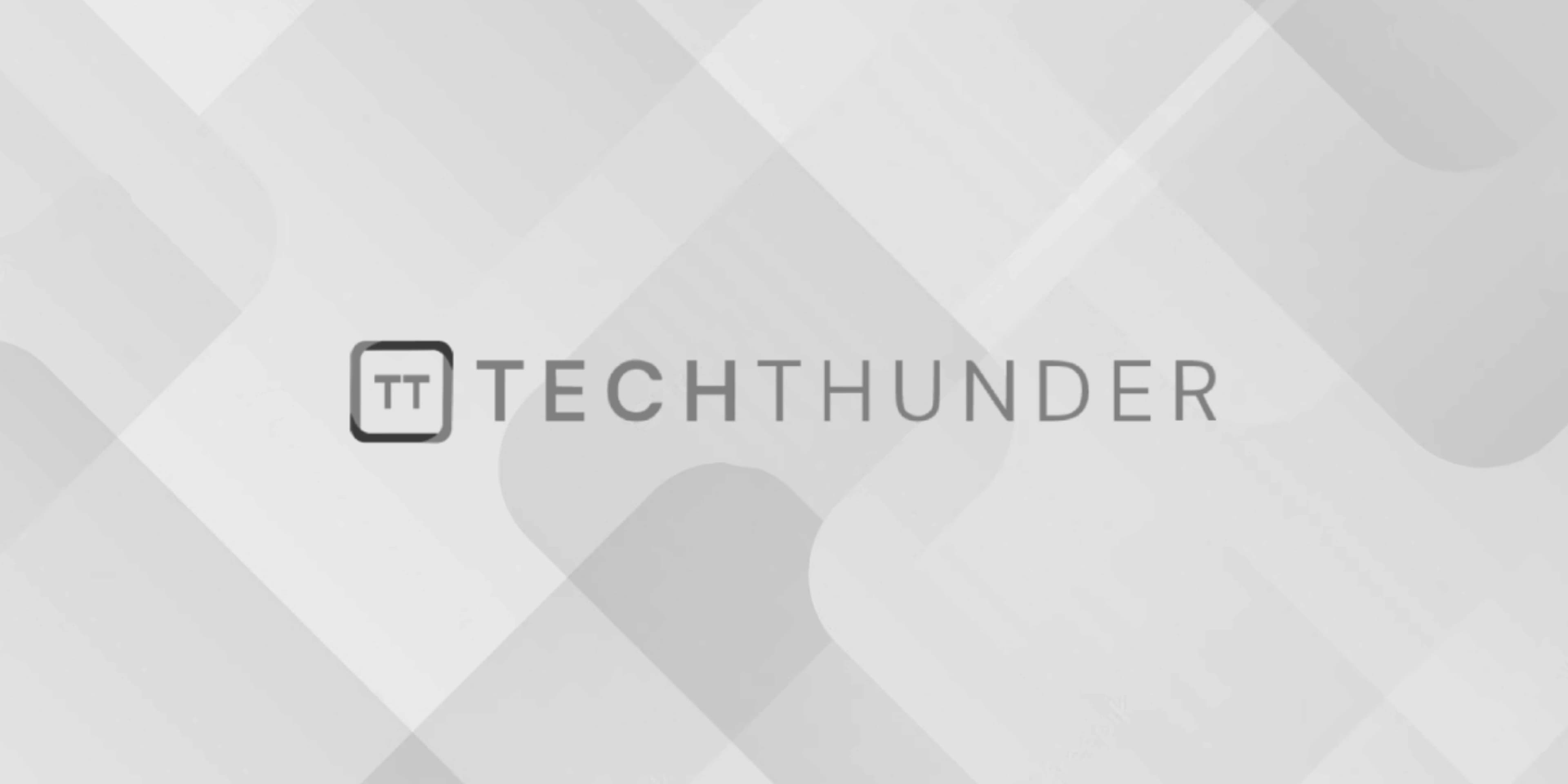
Search Location in Android
Searching for locations in Android typically involves using location-based services, such as geocoding or reverse geocoding, to find places based on user input or device coordinates. Here are the steps to search for a location in an Android app:
1. Obtain Permissions:
First, ensure that your app has the necessary permissions to access the device’s location. You can request location permissions in the AndroidManifest.xml file and request them at runtime if targeting Android 6.0 (API level 23) or higher.
<uses-permission android:name="android.permission.ACCESS_FINE_LOCATION" />
2. Use Google Play Services (Recommended):
Google Play Services provides the Fused Location Provider API, which simplifies location-based operations. To use it, add the following dependency to your app’s build.gradle file:
implementation 'com.google.android.gms:play-services-location:20.2.0'
3. Create a Location Request:
To get the user’s current location, create a LocationRequest
object specifying the update interval, priority, and other settings. For example:
LocationRequest locationRequest = LocationRequest.create()
.setPriority(LocationRequest.PRIORITY_HIGH_ACCURACY)
.setInterval(10000) // 10 seconds
.setFastestInterval(5000); // 5 seconds
4. Request Location Updates:
You can use the FusedLocationProviderClient
to request location updates:
FusedLocationProviderClient fusedLocationClient = LocationServices.getFusedLocationProviderClient(this);
fusedLocationClient.requestLocationUpdates(locationRequest, locationCallback, Looper.getMainLooper());
locationCallback
should be an instance of LocationCallback
where you handle location updates.
5. Geocoding and Reverse Geocoding:
To search for a location based on user input or to retrieve location information from coordinates, you can use geocoding and reverse geocoding services.
- Geocoding is the process of converting a textual location (e.g., an address) into geographic coordinates (latitude and longitude). You can use the
Geocoder
class for geocoding:
Geocoder geocoder = new Geocoder(this);
List<Address> addresses = geocoder.getFromLocationName("Eiffel Tower, Paris", 1);
- Reverse Geocoding is the process of converting geographic coordinates into a human-readable address. You can use the
Geocoder
class for reverse geocoding:
Geocoder geocoder = new Geocoder(this);
List<Address> addresses = geocoder.getFromLocation(latitude, longitude, 1);
6. Handle Permissions and Errors:
Handle cases where the user denies location permissions or when location services are disabled. Display appropriate messages or dialogs to guide the user in enabling location services.
7. Test on Emulators and Devices:
Test your location-related code on physical devices and emulators with various Android versions and settings to ensure compatibility and accuracy.
8. Privacy Considerations:
Respect user privacy and obtain user consent before collecting and using location data. Follow privacy best practices and comply with data protection regulations.
The exact implementation details may vary based on your app’s requirements and the Android API version you are targeting. Always refer to the official Android documentation and consider using Android’s permission system and best practices for location-based services.