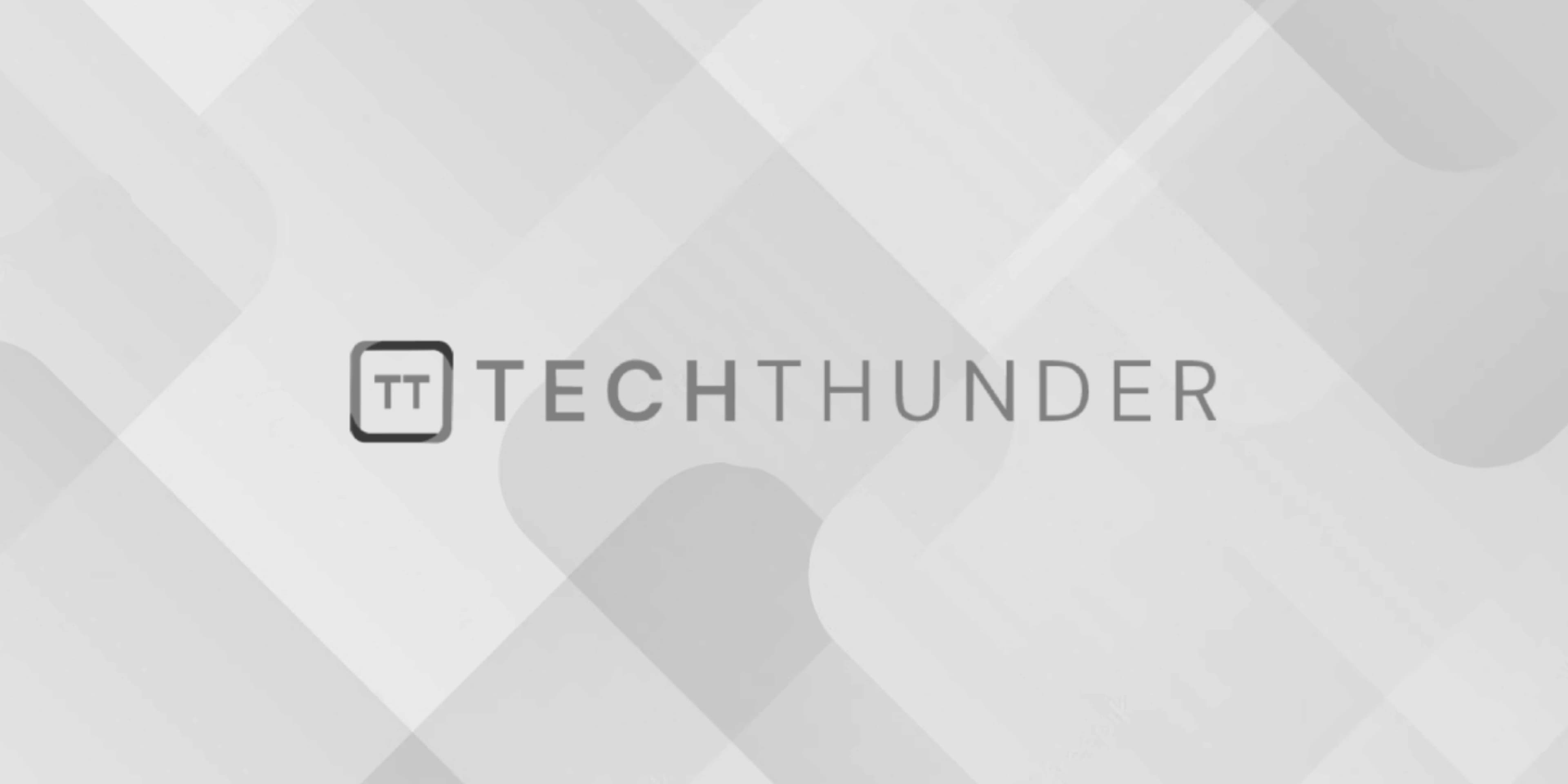
84 views
IP Address Format and Table in Android
The Android, you can work with IP addresses using the InetAddress
class and format them as needed. Here’s how you can format an IP address and create a simple table to display IP-related information in an Android app:
- Format an IP Address: You can format an IP address in Android using the
InetAddress
class. Here’s an example of how to do this:
try {
// Replace "ipString" with your IP address as a string
String ipString = "192.168.0.1";
// Convert the IP address string to an InetAddress object
InetAddress ipAddress = InetAddress.getByName(ipString);
// Format the IP address as a string
String formattedIp = ipAddress.getHostAddress();
// Use the formattedIp as needed in your app
} catch (UnknownHostException e) {
// Handle exceptions if the IP address is invalid
e.printStackTrace();
}
- Create a Table to Display IP Information: To create a table to display IP-related information, you can use Android’s
TableLayout
andTableRow
elements. Here’s an example of how to create a simple table to display IP information:
<!-- res/layout/ip_info_table.xml -->
<TableLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<TableRow>
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="IP Address:"
android:paddingEnd="10dp"
android:gravity="end"
android:labelFor="@id/ip_address_value" />
<TextView
android:id="@+id/ip_address_value"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="192.168.0.1" />
</TableRow>
<!-- Add more rows for additional IP-related information as needed -->
</TableLayout>
You can then inflate this layout in your Android activity or fragment and add it to your view as needed.
- Inflate the Table Layout in Activity: In your Android activity, you can inflate the
ip_info_table.xml
layout and add it to your view like this:
setContentView(R.layout.ip_info_table);
You can also find views within the table and update their values programmatically using the findViewById
method.
This example demonstrates how to format an IP address and create a basic table layout to display IP-related information in an Android app. You can extend this concept to display more details about IP addresses or customize the table’s appearance as per your app’s requirements.