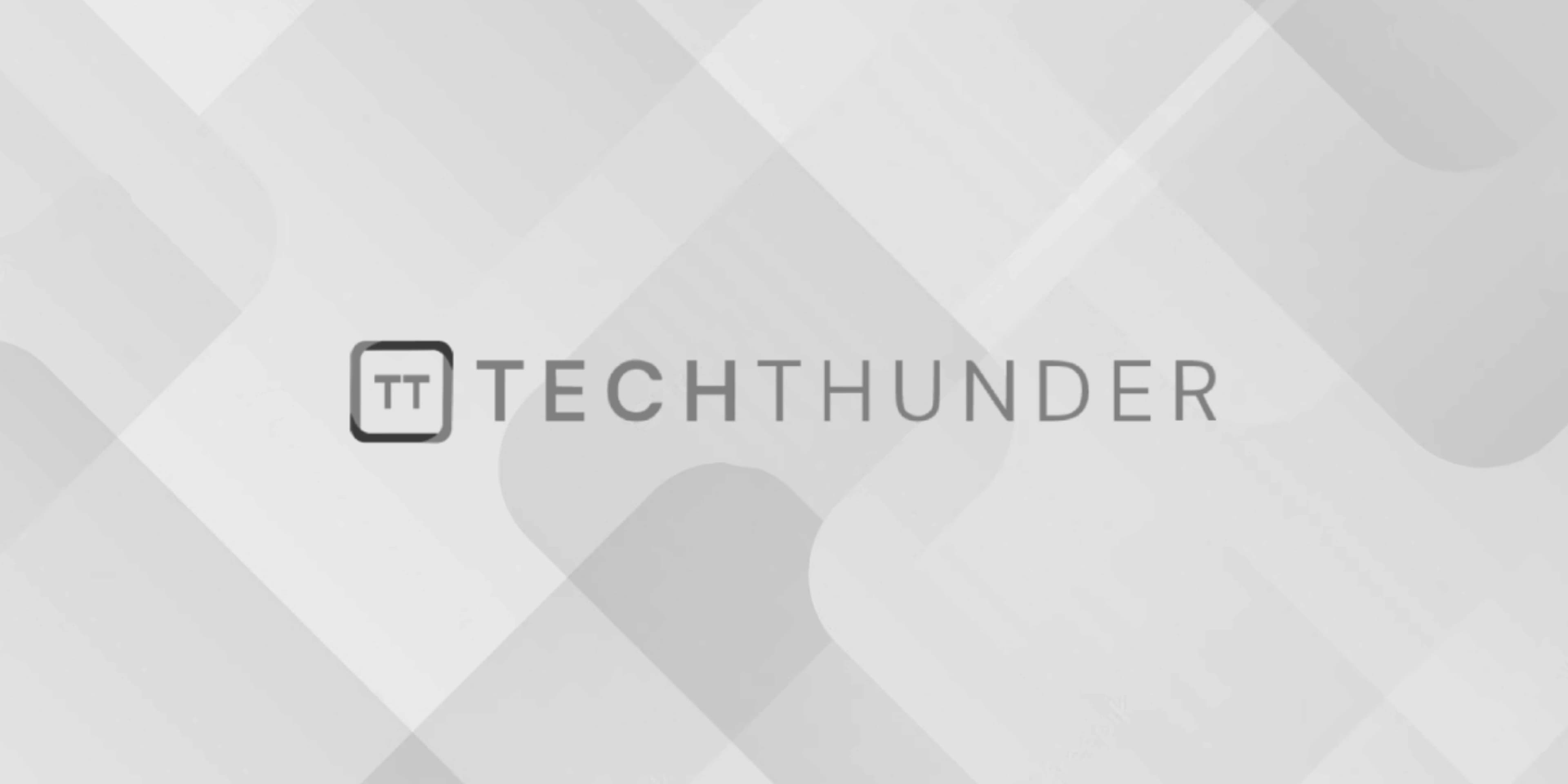
ViewStub in Android
The ViewStub
is a lightweight and efficient way to load and inflate views lazily in Android. It allows you to define and include views in your layout XML but doesn’t inflate them immediately when the layout is inflated. Instead, it defers the inflation until the view is actually needed. This can help improve the performance of your app by reducing the initial layout inflation time and memory usage.
Here’s how to use ViewStub
in Android:
- Add a ViewStub to Your Layout: In your XML layout file, include a
ViewStub
element where you want to load a view lazily. You can define the layout that theViewStub
will inflate using theandroid:layout
attribute:
<ViewStub
android:id="@+id/view_stub"
android:layout="@layout/your_lazy_layout"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:inflatedId="@+id/inflated_view" />
Replace your_lazy_layout
with the layout resource you want to inflate lazily when needed.
- Reference the ViewStub in Java/Kotlin: In your Java or Kotlin code, you need to obtain a reference to the
ViewStub
using its ID. You can do this in your activity or fragment:
ViewStub viewStub = findViewById(R.id.view_stub);
- Inflate the View When Needed: You can inflate the view associated with the
ViewStub
when it’s needed, typically triggered by a user action or some condition in your code. To do this, call theinflate()
method on theViewStub
:
View inflatedView = viewStub.inflate();
The inflate()
method returns the inflated view, and you can manipulate it like any other view.
- Customize the Inflated View: After inflating the view, you can customize its content or appearance programmatically. For example:
TextView textView = inflatedView.findViewById(R.id.text_view);
textView.setText("This is the lazy-loaded view.");
You can access and manipulate the child views within the inflated view using their IDs.
- Optional: Set an OnInflateListener: You can set an
OnInflateListener
on theViewStub
to perform actions when the view is inflated. This listener can be useful if you need to initialize or customize the inflated view further:
viewStub.setOnInflateListener(new ViewStub.OnInflateListener() {
@Override
public void onInflate(ViewStub stub, View inflated) {
// Perform actions when the view is inflated
}
});
ViewStub
is especially useful when you have views in your layout that are not always needed, such as optional sections, dialogs, or components that appear conditionally. It helps reduce the initial layout complexity and memory usage by loading views only when they are required, which can lead to improved app performance.