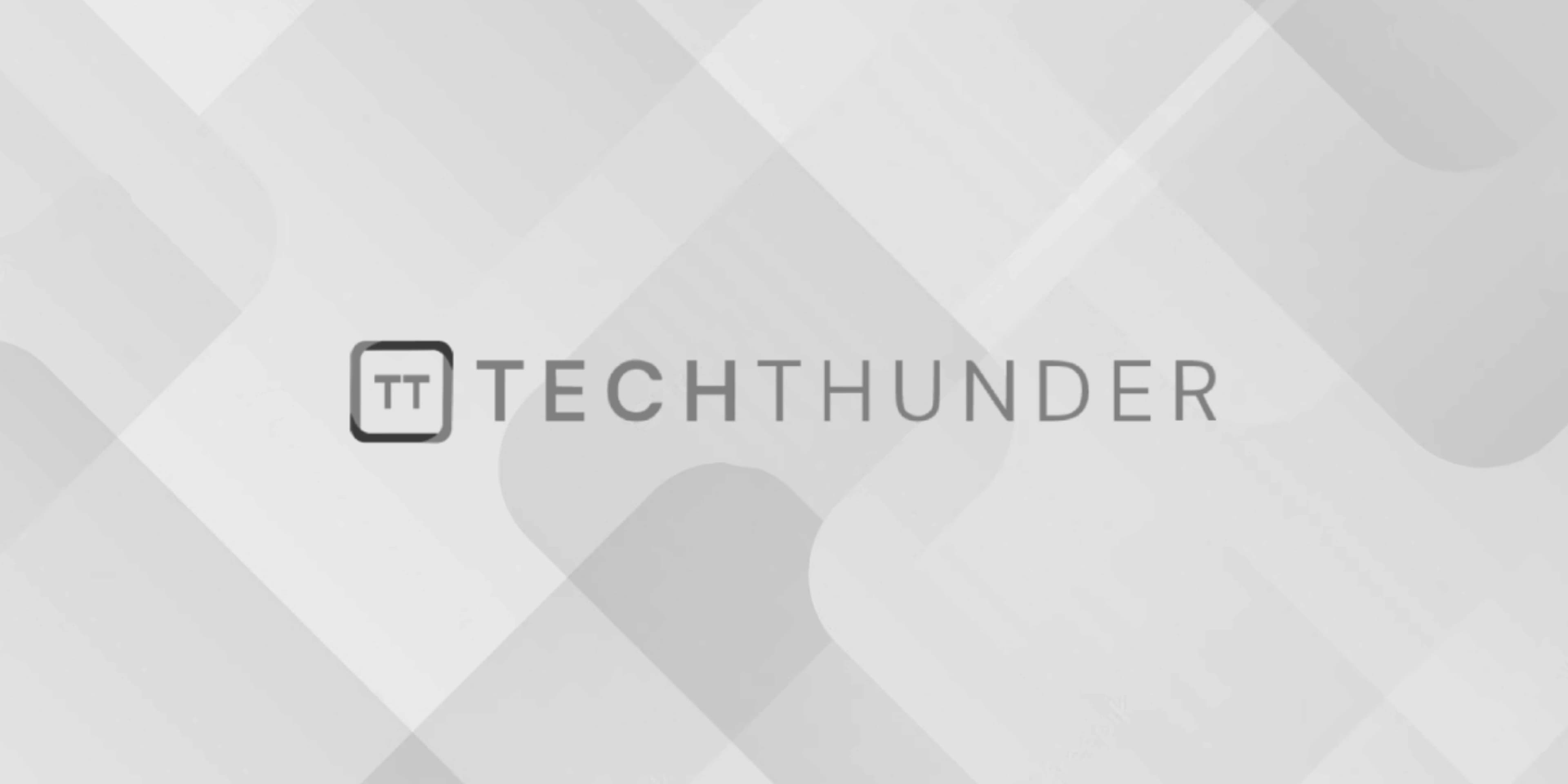
Horizontal ScrollView in Android
The Android HorizontalScrollView
is a view container that allows its child views to be scrolled horizontally, typically when the content within the view is too wide to fit within the screen’s width. It provides a horizontal scrollable area for displaying content like images, text, or other UI elements.
Here’s how you can use a HorizontalScrollView
in your Android app:
- XML Layout:
- Create a layout XML file where you want to include the
HorizontalScrollView
.
<HorizontalScrollView
android:id="@+id/horizontal_scroll_view"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<!-- Child views go here, such as ImageViews, TextViews, or other UI elements -->
<!-- These child views should be placed inside a horizontal LinearLayout or RelativeLayout -->
</HorizontalScrollView>
Inside the HorizontalScrollView
, you’ll typically include a layout container (e.g., LinearLayout
or RelativeLayout
) to hold your child views.
- Child Views:
- Inside the
HorizontalScrollView
, add child views that you want to be horizontally scrollable. These child views can be any UI elements likeImageView
,TextView
,Button
, etc.
<HorizontalScrollView
android:id="@+id/horizontal_scroll_view"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<LinearLayout
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:orientation="horizontal">
<!-- Child views go here -->
<ImageView
android:layout_width="100dp"
android:layout_height="100dp"
android:src="@drawable/image1" />
<ImageView
android:layout_width="100dp"
android:layout_height="100dp"
android:src="@drawable/image2" />
<!-- Add more child views as needed -->
</LinearLayout>
</HorizontalScrollView>
In this example, a LinearLayout
is used as a container for horizontally arranging the child ImageViews
.
- Programmatic Access:
- In your Java code, you can programmatically access the
HorizontalScrollView
and its child views using their IDs:
HorizontalScrollView horizontalScrollView = findViewById(R.id.horizontal_scroll_view);
// Access child views within the HorizontalScrollView
ImageView imageView1 = findViewById(R.id.image_view_1);
ImageView imageView2 = findViewById(R.id.image_view_2);
// ...
- Scrolling Programmatically:
- You can programmatically scroll the
HorizontalScrollView
to a specific position using methods likescrollTo()
orsmoothScrollTo()
:
horizontalScrollView.scrollTo(200, 0); // Scrolls to a specific X position
- Adjustments and Styling:
- You can adjust the width, height, and styling of the
HorizontalScrollView
and its child views to fit your layout and design requirements.
The HorizontalScrollView
allows you to create horizontally scrollable content within your Android app, making it useful for displaying wide images, lists, or any content that doesn’t fit entirely on the screen.