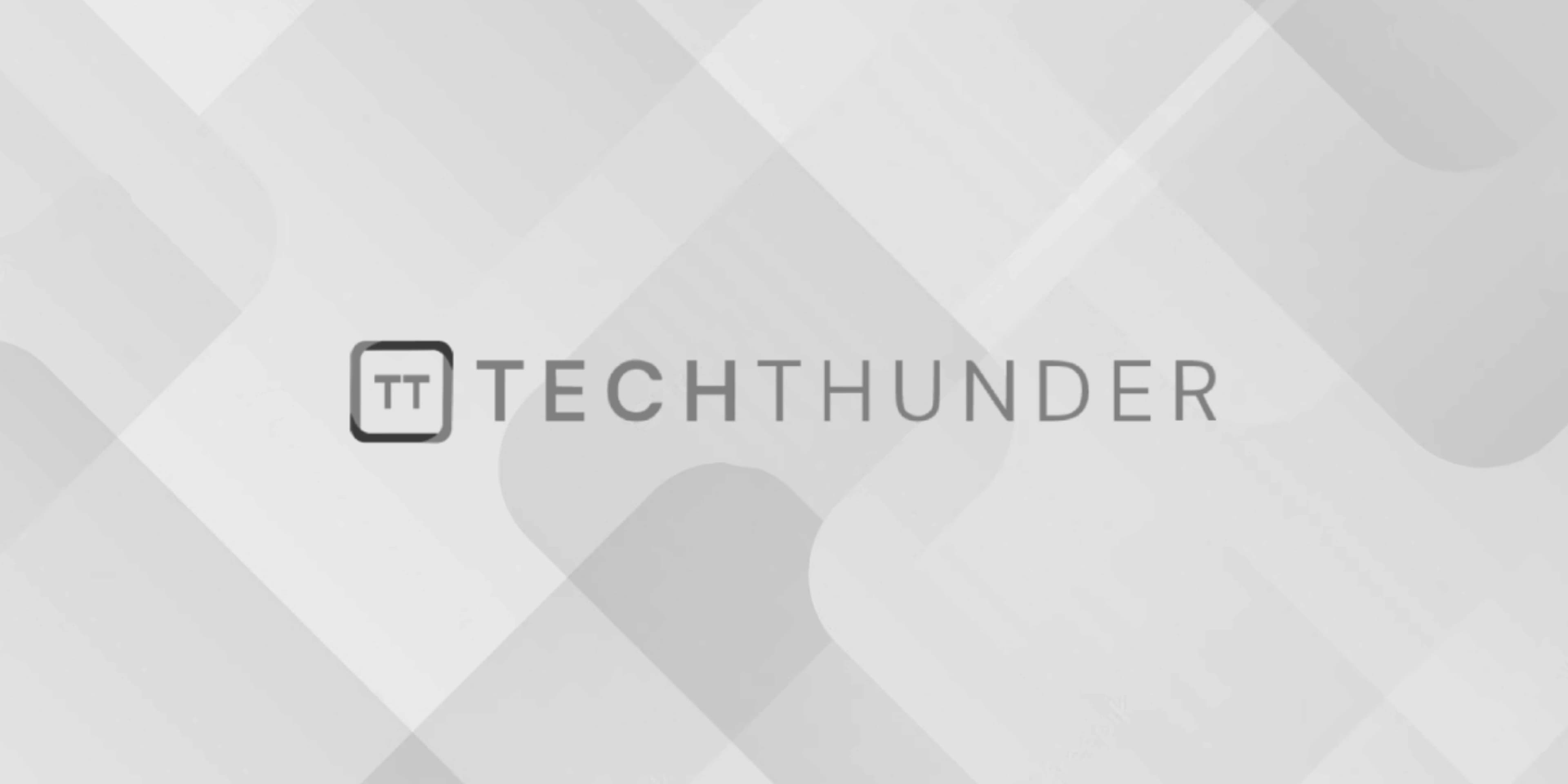
CheckBox in Android
The Android CheckBox
is a user interface component that allows users to toggle a binary choice on or off. It’s typically used to collect yes/no or true/false input from users. Here’s how to use a CheckBox
in Android:
Add the CheckBox
to Your Layout: In your XML layout file, add a CheckBox
element where you want to include the binary choice functionality:
<CheckBox
android:id="@+id/check_box"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Check me" />
android:id
: Assign a unique ID to theCheckBox
for later reference.android:layout_width
andandroid:layout_height
: Adjust these attributes based on your layout requirements.android:text
: Specify the label text for theCheckBox
.
Access the CheckBox
in Your Activity or Fragment: In your Java or Kotlin code (usually within your activity or fragment), obtain a reference to the CheckBox
using its ID:
CheckBox checkBox = findViewById(R.id.check_box);
Handle Check State Changes: To respond to changes in the check state (checked or unchecked) made by the user, you can set an OnCheckedChangeListener
:
checkBox.setOnCheckedChangeListener(new CompoundButton.OnCheckedChangeListener() {
@Override
public void onCheckedChanged(CompoundButton buttonView, boolean isChecked) {
// Handle the check state change here
// 'isChecked' is true if the CheckBox is checked, false if unchecked
}
});
The onCheckedChanged
method is called when the user checks or unchecks the CheckBox
. You can perform actions based on the isChecked
value.
Run Your App: When you run your app, the CheckBox
will be displayed with the specified label text. Users can tap on it to toggle its check state. The onCheckedChanged
method will be called when the user changes the check state.
Customization (Optional): You can customize the appearance and behavior of the CheckBox
by setting various attributes in XML or programmatically. For example, you can set the initial check state, change the label text, and more.
<!-- Customizing the CheckBox -->
<CheckBox
android:id="@+id/check_box"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Custom Label"
android:checked="true" // Set the initial check state
android:textSize="18sp" // Set the text size
android:textColor="@color/checkbox_text_color" // Set the text color
android:buttonTint="@color/checkbox_color" // Set the checkbox color
android:layout_gravity="center"
/>
You can customize these attributes to match your app’s design and requirements.
You can easily implement a CheckBox
in your Android app to collect binary choice input from users and respond to check state changes as needed.