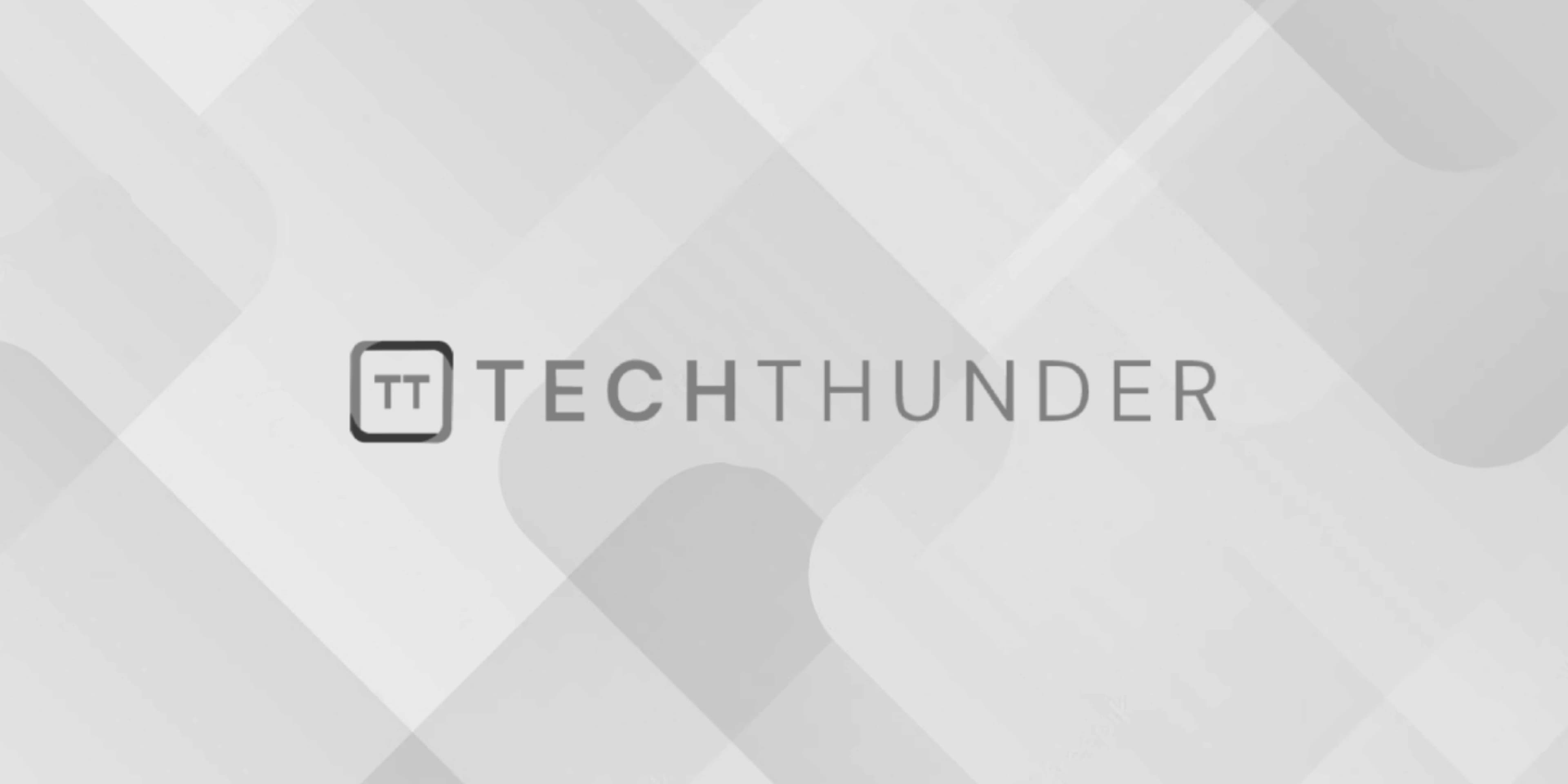
Swipe Del RecyclerView in Android
Swiping in a RecyclerView
in Android is often used to implement features like swipe-to-delete or swipe-to-archive for items in a list. To achieve this, you can use a combination of ItemTouchHelper
and ItemTouchHelper.SimpleCallback
. Here’s a step-by-step guide on how to implement swipe-to-delete functionality in a RecyclerView
:
- Add Dependencies:
Open your app’sbuild.gradle
file and add the following dependencies if you haven’t already:
implementation 'androidx.recyclerview:recyclerview:1.2.1'
- Create Your RecyclerView:
In your XML layout file, add aRecyclerView
widget. For example:
<androidx.recyclerview.widget.RecyclerView
android:id="@+id/recyclerView"
android:layout_width="match_parent"
android:layout_height="match_parent"
/>
- Create an Adapter:
Create an adapter for yourRecyclerView
to display the data. You can useRecyclerView.Adapter
to achieve this. - Implement Swipe-to-Delete Callback:
Create a class that extendsItemTouchHelper.SimpleCallback
to handle the swipe-to-delete functionality. Override the necessary methods, such asonSwiped
to delete items andonMove
for item reordering (if needed). Here’s an example:
public class SwipeToDeleteCallback extends ItemTouchHelper.SimpleCallback {
private MyAdapter adapter;
public SwipeToDeleteCallback(MyAdapter adapter) {
super(0, ItemTouchHelper.LEFT | ItemTouchHelper.RIGHT);
this.adapter = adapter;
}
@Override
public boolean onMove(RecyclerView recyclerView, RecyclerView.ViewHolder viewHolder, RecyclerView.ViewHolder target) {
return false;
}
@Override
public void onSwiped(RecyclerView.ViewHolder viewHolder, int direction) {
int position = viewHolder.getAdapterPosition();
adapter.deleteItem(position);
}
}
- Attach ItemTouchHelper to RecyclerView:
In your activity or fragment, create an instance ofItemTouchHelper
with yourSwipeToDeleteCallback
and attach it to theRecyclerView
.
RecyclerView recyclerView = findViewById(R.id.recyclerView);
MyAdapter adapter = new MyAdapter(dataList); // Replace with your adapter and data
recyclerView.setAdapter(adapter);
SwipeToDeleteCallback swipeHandler = new SwipeToDeleteCallback(adapter);
ItemTouchHelper itemTouchHelper = new ItemTouchHelper(swipeHandler);
itemTouchHelper.attachToRecyclerView(recyclerView);
- Implement Delete Method in Adapter:
In your adapter (MyAdapter
in this example), implement a method to delete items from your dataset and notify the adapter of the changes. For example:
public class MyAdapter extends RecyclerView.Adapter<MyAdapter.ViewHolder> {
// ...
public void deleteItem(int position) {
data.remove(position);
notifyItemRemoved(position);
}
// ...
}
Now, when you swipe an item left or right in your RecyclerView
, it will trigger the onSwiped
method in your SwipeToDeleteCallback
, which in turn calls the deleteItem
method in your adapter to remove the item from the dataset and update the UI.
Remember to adapt the code to your specific use case, including customizing the appearance of swiped items, adding confirmation dialogs, or handling other actions besides deletion if needed.