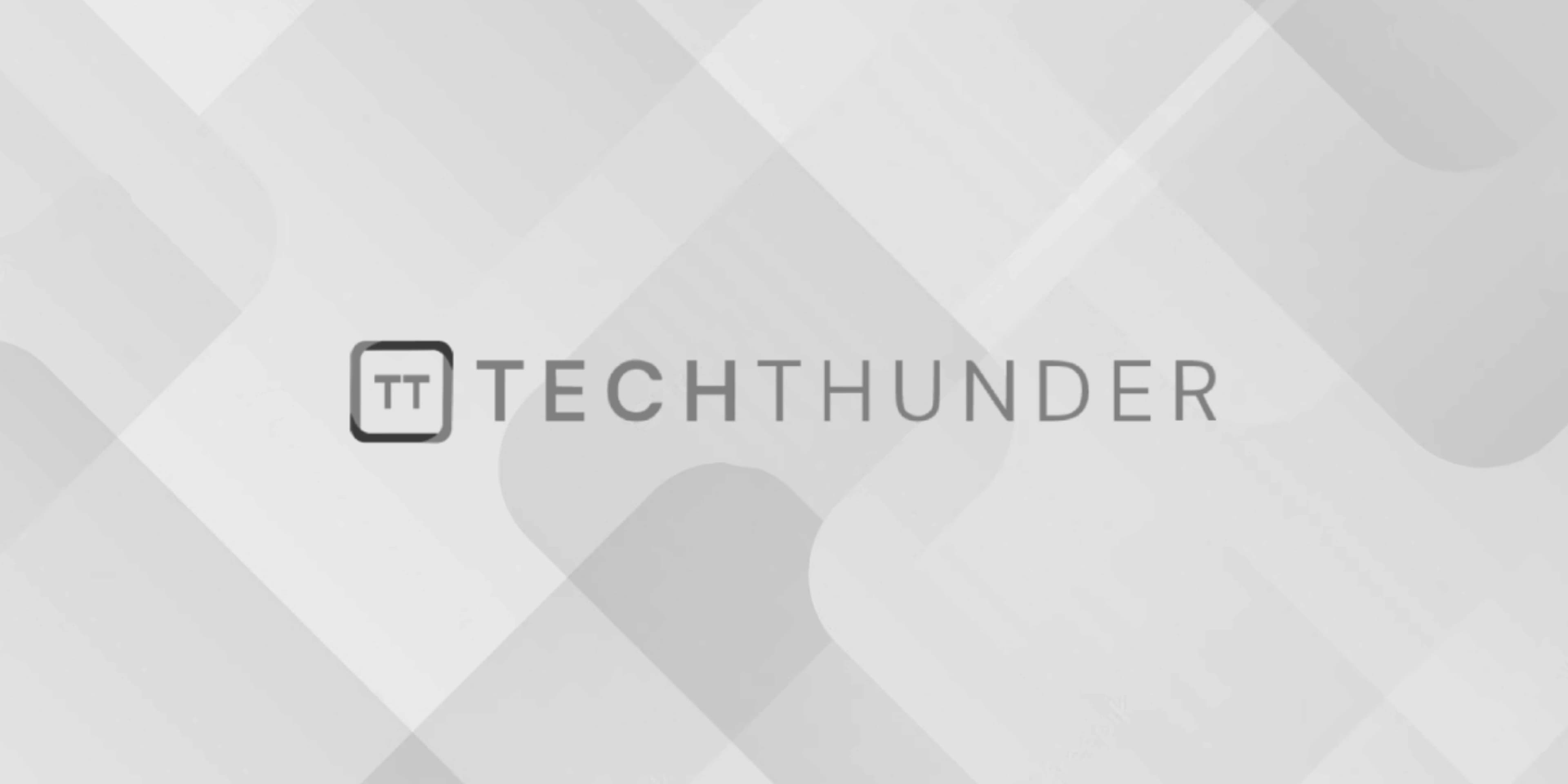
Hello Android example
Creating a “Hello, Android” example is a common starting point for beginners to get familiar with Android app development. In this example, we’ll create a simple Android app that displays the text “Hello, Android!” on the screen. Here are the steps to create this basic Android app:
Set Up Your Development Environment: Before you begin, make sure you have Android Studio installed and configured on your computer. You can download Android Studio from the official Android developer website.
Create a New Android Project: Open Android Studio and click on “Start a new Android Studio project” or go to File > New > New Project
.
- Choose an appropriate project template, such as “Empty Activity” or “Basic Activity.”
- Set a name for your project and choose a package name.
- Select the programming language you prefer (Java or Kotlin).
- Click “Finish” to create your project.
Design the Layout: Open the XML layout file for your main activity (activity_main.xml
) in the res/layout
directory. Replace the existing layout with a TextView
to display the “Hello, Android!” text:
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:padding="16dp"
tools:context=".MainActivity">
<TextView
android:id="@+id/helloTextView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Hello, Android!"
android:textSize="24sp"
android:layout_centerInParent="true" />
</RelativeLayout>
Update the Activity Code: Open the main activity file (usually MainActivity.java
or MainActivity.kt
) in the src
directory. Add the following code to set up the TextView
and display the “Hello, Android!” message: Java:
import android.os.Bundle;
import android.support.v7.app.AppCompatActivity;
import android.widget.TextView;
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// Find the TextView by its ID
TextView helloTextView = findViewById(R.id.helloTextView);
// Set the text for the TextView
helloTextView.setText("Hello, Android!");
}
}
import android.os.Bundle
import androidx.appcompat.app.AppCompatActivity
import kotlinx.android.synthetic.main.activity_main.*
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
// Set the text for the TextView
helloTextView.text = "Hello, Android!"
}
}
Run Your App: Connect a physical Android device to your computer or use an Android Virtual Device (AVD) from Android Studio. Click the “Run” button in Android Studio to build and run your app. After a moment, your app will launch on the device or emulator, and you should see the “Hello, Android!” message displayed on the screen.
Finally! You’ve created a basic “Hello, Android” app. This simple example demonstrates the essential steps of creating an Android app, including setting up the layout, modifying the activity code, and running the app on a device or emulator. You can use this as a starting point to learn and explore more advanced Android app development concepts.