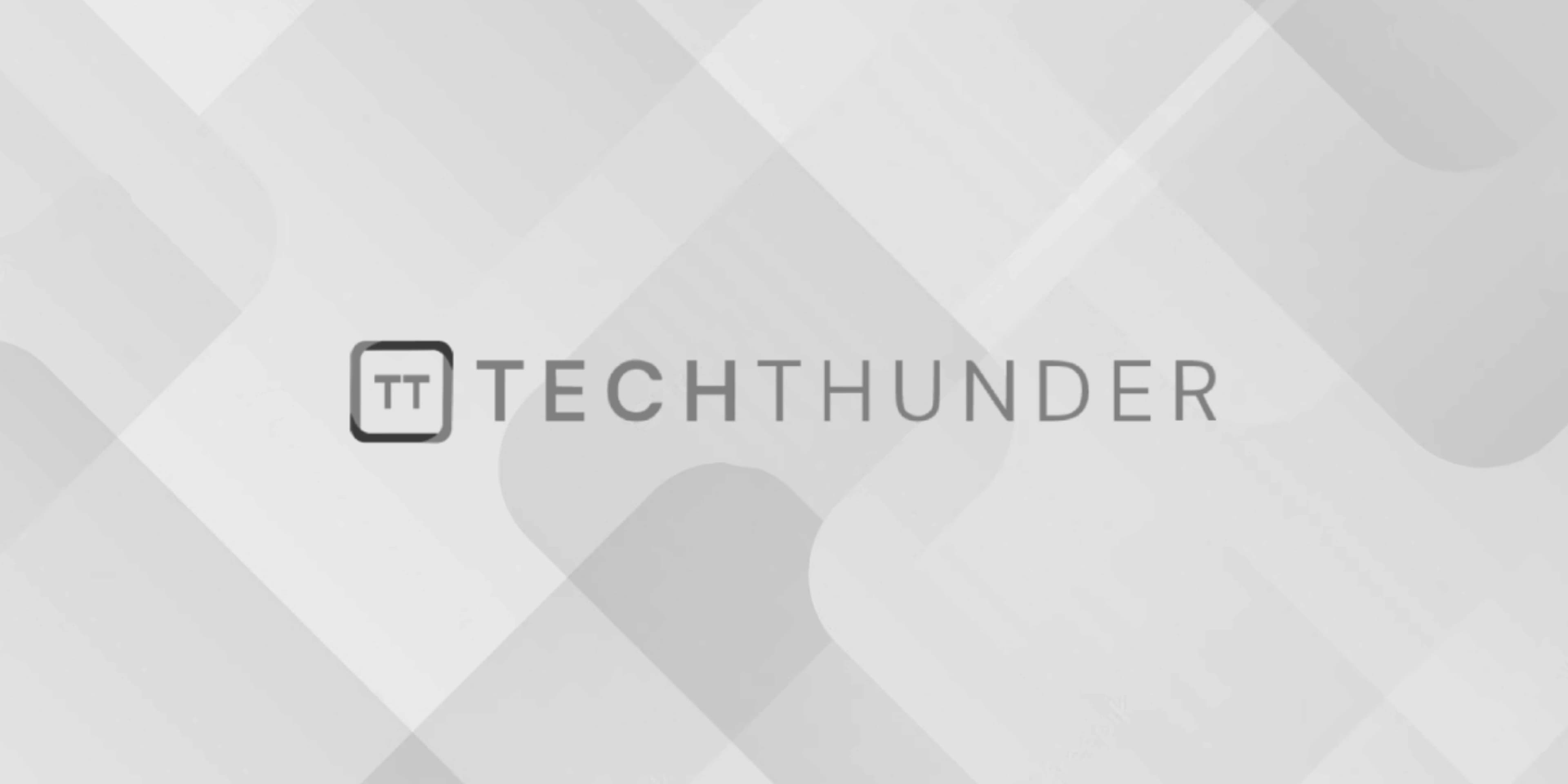
296 views
Android Architecture
The Android operating system is built upon a layered architecture that provides a structured framework for developing and running Android applications. Understanding the Android architecture is crucial for Android developers. The key components of the Android architecture include:
- Linux Kernel:
- At the core of Android is the Linux kernel, which provides low-level hardware abstraction, process management, and security features.
- The Linux kernel serves as the foundation for Android’s multitasking, memory management, and hardware drivers.
- Hardware Abstraction Layer (HAL):
- Above the Linux kernel is the Hardware Abstraction Layer (HAL), which bridges the gap between the hardware drivers and the Android framework.
- It provides a standardized interface for accessing hardware components like camera, sensors, and audio.
- Native Libraries:
- Native libraries consist of essential libraries written in C and C++ that provide core functionality for Android.
- These libraries include the Standard C Library (libc), SQLite (database engine), and OpenGL ES (graphics rendering), among others.
- Android Runtime (ART):
- ART is the runtime environment responsible for executing Android applications.
- Starting with Android 5.0 (Lollipop), ART replaced the earlier Dalvik VM as the default runtime.
- ART uses Ahead-of-Time (AOT) compilation to convert app bytecode into native machine code at installation, which improves app performance.
- Core Libraries and Android Framework:
- Android provides a set of core libraries and the Android framework that simplifies app development.
- These libraries offer reusable components for tasks such as UI rendering, data storage, network communication, and more.
- Developers use Java or Kotlin to build Android apps and leverage these libraries for various functionalities.
- Application Framework:
- The Application Framework layer provides a set of high-level services and components that developers can use to build Android apps.
- Key components include activities, services, content providers, broadcast receivers, and the user interface framework.
- The framework also manages app lifecycle, inter-component communication, and user interface rendering.
- System Apps and User Apps:
- Android includes a set of system apps that come pre-installed on devices and provide core system functionality (e.g., Phone, Settings, Browser).
- User apps are the apps that users install from app stores like Google Play.
- Both system and user apps run within the Android framework and interact with the underlying layers.
- User Interface (UI):
- Android’s user interface is based on a hierarchical view system, with layouts and views defining the app’s UI structure.
- Developers can create UIs using XML layout files or programmatically through Java or Kotlin code.
- Application Package (APK):
- Android apps are packaged as APK files containing compiled code (in .dex format), resources, and a manifest file.
- The manifest file specifies the app’s components, permissions, and entry points.
- Dalvik Debug Monitor Server (DDMS):
- DDMS is a debugging tool used for monitoring and debugging Android applications.
- It provides features for tracking memory usage, debugging processes, and interacting with running apps.
- Google Play Services:
- Google Play Services is a collection of APIs and services provided by Google for Android developers.
- It offers features such as location services, Google Maps integration, in-app purchasing, and cloud-based services.
- Security Model:
- Android’s security model includes various layers of protection, including app sandboxing, permissions, and encryption.
- Users can control app permissions, and apps are isolated from one another to enhance security.
Understanding this layered architecture is essential for Android developers to design and build apps that work seamlessly with the Android platform and provide a rich user experience.