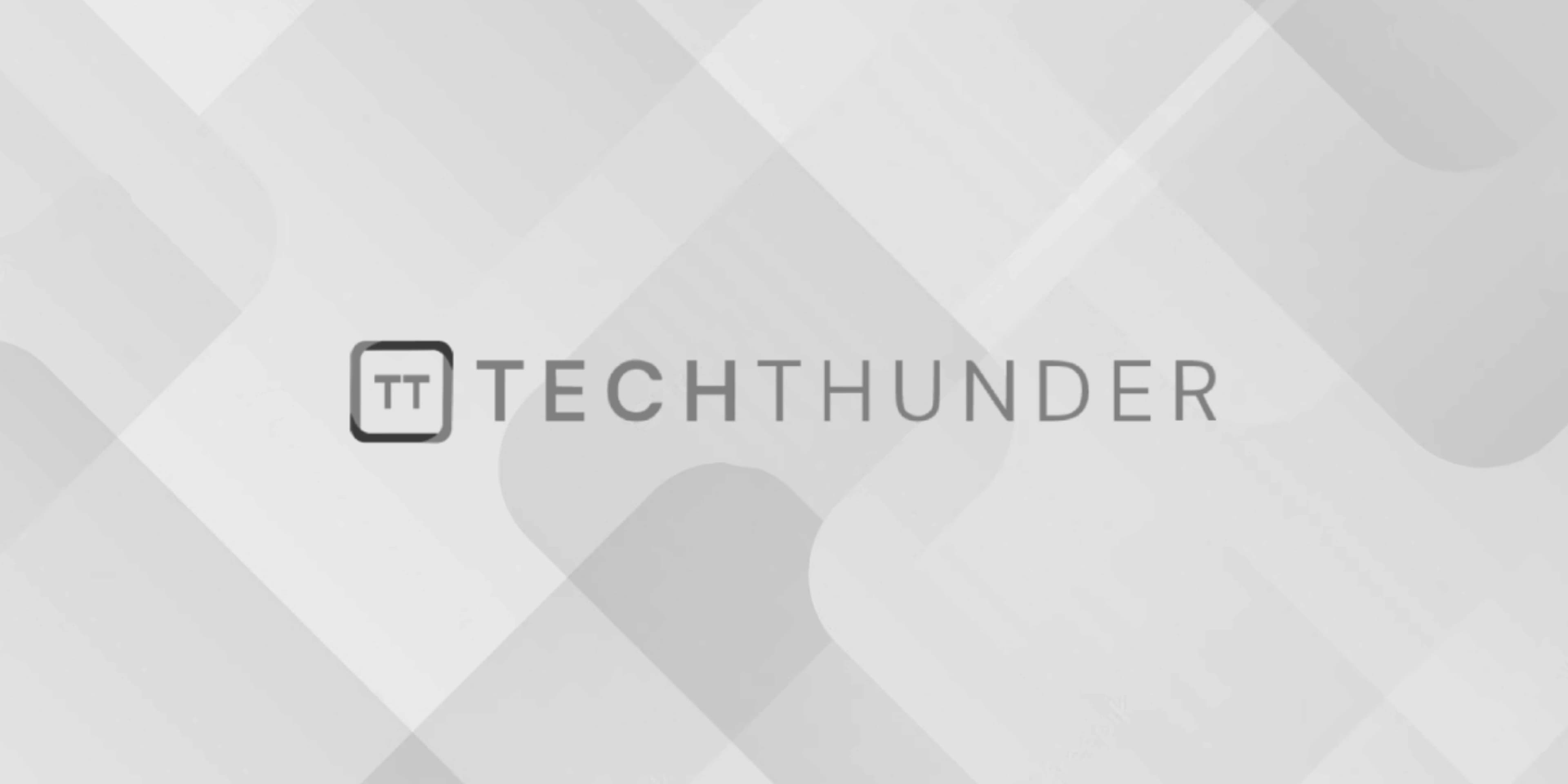
Send Email in Android
To send an email from an Android app, you can use the Android Intent system to launch the user’s preferred email client (e.g., Gmail, Outlook) with pre-filled information, such as the recipient’s email address, subject, and message body. Here’s how to do it:
public void sendEmail() {
// Create an intent to send an email
Intent emailIntent = new Intent(Intent.ACTION_SEND);
emailIntent.setType("text/plain");
// Set the recipient email address
emailIntent.putExtra(Intent.EXTRA_EMAIL, new String[]{"[email protected]"});
// Set the email subject
emailIntent.putExtra(Intent.EXTRA_SUBJECT, "Subject of the Email");
// Set the email message body
emailIntent.putExtra(Intent.EXTRA_TEXT, "This is the message body of the email.");
try {
// Start the email client activity
startActivity(Intent.createChooser(emailIntent, "Send Email"));
} catch (ActivityNotFoundException ex) {
// Handle the case where no email app is available
Toast.makeText(this, "No email app installed on this device.", Toast.LENGTH_SHORT).show();
}
}
In this code:
- We create an
Intent
with the actionIntent.ACTION_SEND
, which indicates that we want to send data. - We specify the data type as
"text/plain"
to indicate that we are sending plain text content. - We use
putExtra
to add recipient email addresses, the subject, and the message body to the intent. - We use
Intent.createChooser
to present the user with a list of email apps to choose from. The user can then select their preferred email client to send the email.
Make sure to replace "[email protected]"
, "Subject of the Email"
, and "This is the message body of the email."
with your actual recipient’s email address, subject, and message body.
You should also add the necessary permissions to your AndroidManifest.xml
file:
<uses-permission android:name="android.permission.INTERNET" />
This permission is required to send emails via the user’s email client.
Remember that this code relies on the user having an email client installed on their device. If the user doesn’t have one, you should handle this case gracefully, as shown in the code example.