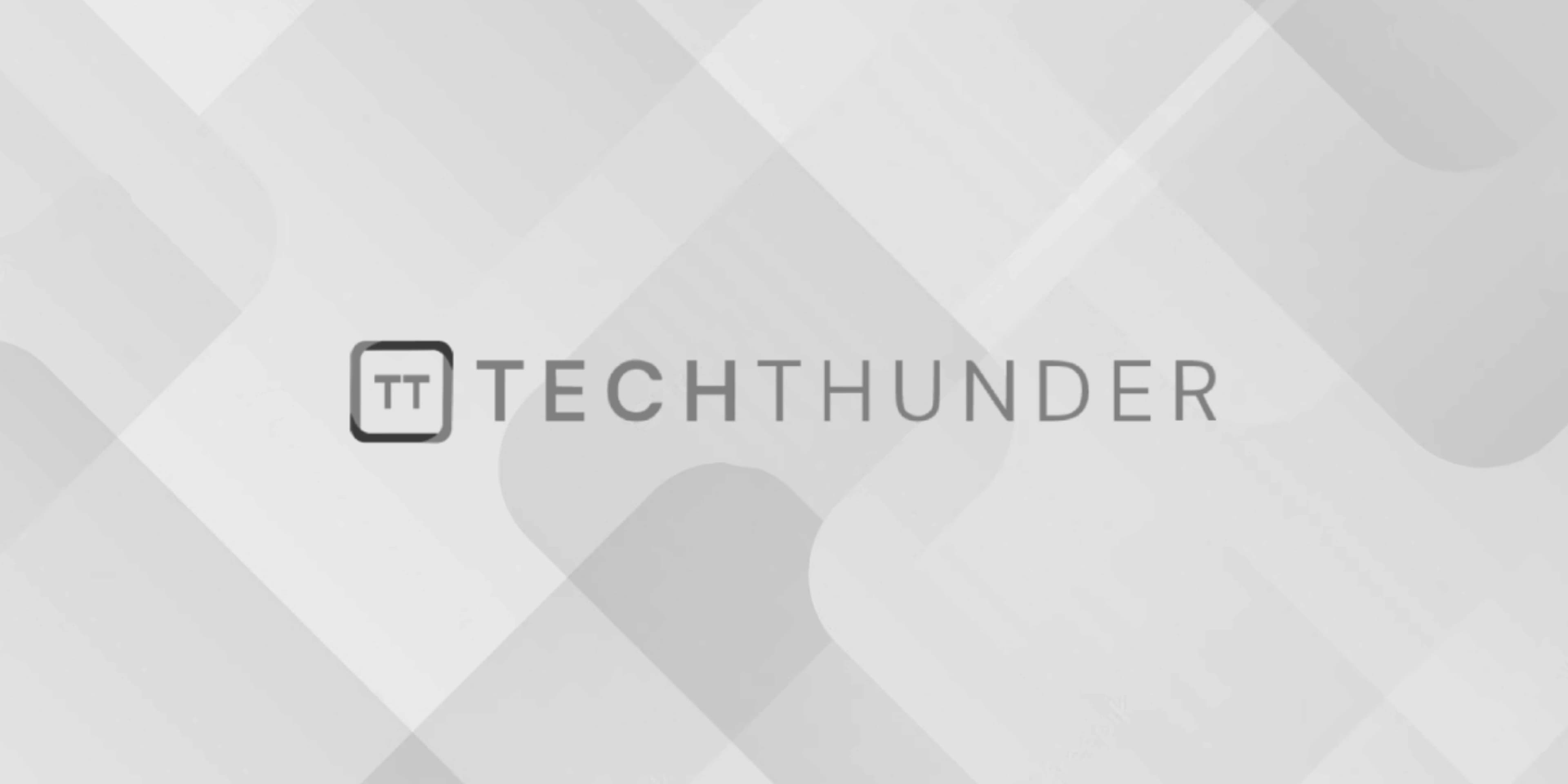
Android Notification
Android notifications are a way for Android apps to provide timely information and updates to users. Notifications can appear in various forms, such as text messages, alerts, or icons in the notification bar, and they can be used to inform users about new messages, emails, events, app updates, and more. Here are some key concepts and components related to Android notifications:
Notification Components:
- Notification Title: A short, descriptive title for the notification.
- Notification Content: The main text or message of the notification.
- Notification Icon: An icon that represents the app or notification.
- Notification Actions: Buttons or options that allow users to interact with the notification (e.g., “Reply,” “Dismiss”).
- Notification Intent: The action to be performed when the user interacts with the notification (e.g., opening an app, launching a specific activity).
Notification Channels:
- Introduced in Android 8.0 (API level 26) and later, notification channels allow you to categorize notifications and give users more control over which notifications they receive from your app. Users can customize notification settings for each channel, including sound, vibration, and importance level.
Notification Manager:
- The
NotificationManager
class is responsible for managing notifications. You use it to create, update, and cancel notifications.
Notification Builder:
- You typically create notifications using the
NotificationCompat.Builder
class. This builder allows you to set various notification attributes, such as title, content, icon, and actions, and then build the notification using thebuild()
method.
PendingIntent:
- To specify the action to be taken when the user interacts with the notification, you create a
PendingIntent
. APendingIntent
represents an intent that will be executed in the future, typically launching an activity, service, or broadcast.
Notification Channels (Android 8.0 and later):
- Starting with Android 8.0 (Oreo), you should use notification channels to categorize and group your notifications. This allows users to manage notifications more effectively.
Notification Actions:
- You can add actions to notifications that allow users to perform actions directly from the notification, such as replying to a message or snoozing a reminder.
BigTextStyle and InboxStyle:
- These styles allow you to create expandable notifications that display longer text content or a list of messages.
Heads-up Notifications (Priority):
- Notifications can have different levels of priority. High-priority notifications can appear as “heads-up” notifications that briefly appear at the top of the screen to get the user’s attention.
- Custom Notifications:
- You can create custom notifications with custom layouts, styles, and behaviors to match the look and feel of your app.
- Notification Groups:
- You can group related notifications together to provide a cleaner and more organized notification shade for users.
- Notification Badges (App Icon Badges):
- Some Android launchers and devices support notification badges, which display a count of unread notifications on the app icon.
To create and manage notifications in your Android app, you’ll typically use the Android Notification API in conjunction with the NotificationManager, NotificationCompat.Builder, PendingIntent, and other related classes. Android’s notification system is versatile and allows you to provide a seamless and informative user experience in your app.