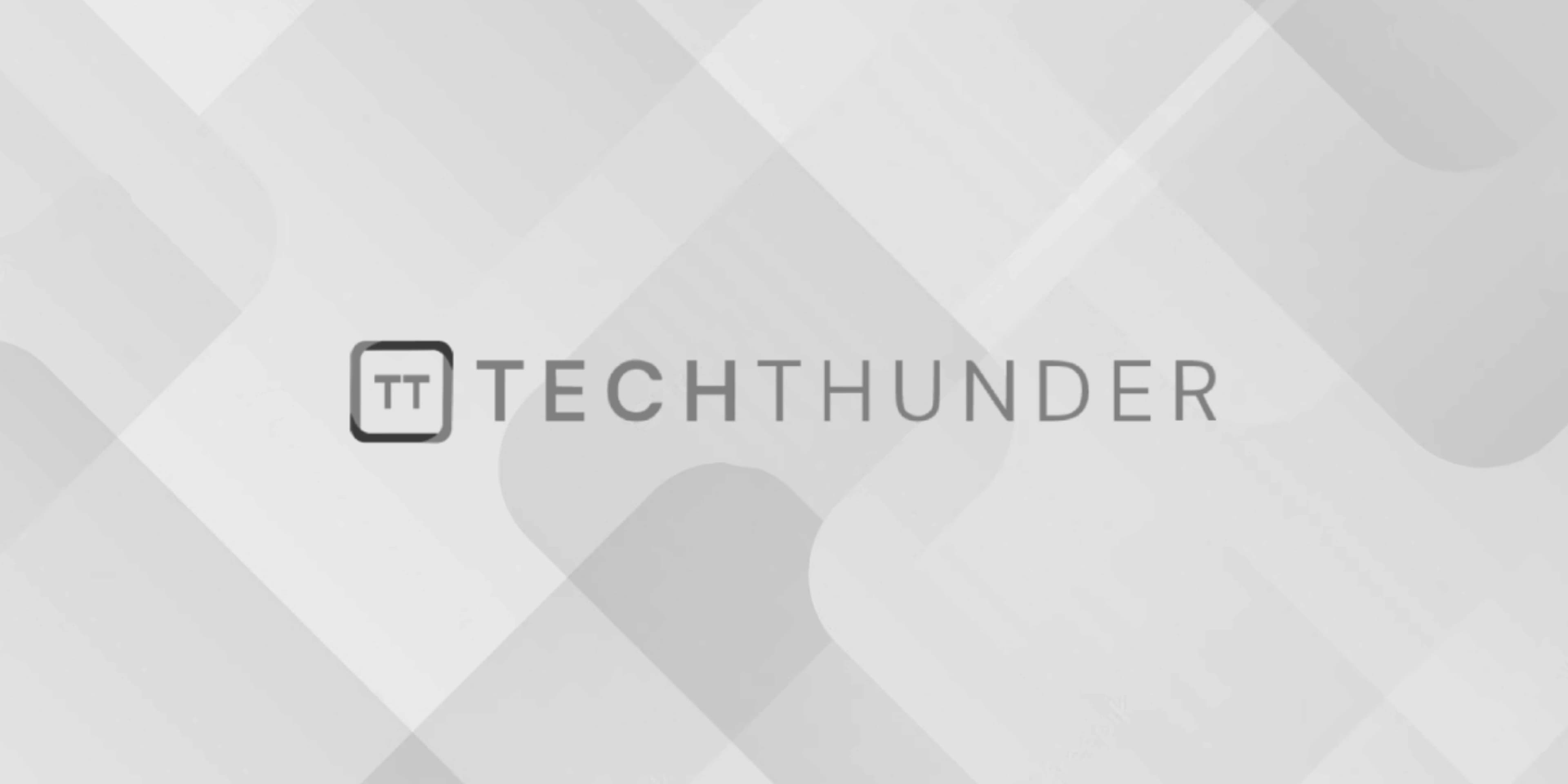
Toast in Android
The Android Toast
is a simple feedback mechanism that displays a brief message to the user at the bottom of the screen. It is often used to provide temporary information, notifications, or feedback without interrupting the user’s current interaction with the app. Here’s how you can create and display a Toast
in Android:
// Create and display a short-duration Toast
Toast.makeText(getApplicationContext(), "This is a short-duration Toast", Toast.LENGTH_SHORT).show();
In this example:
Toast.makeText()
: This method is used to create aToast
object.getApplicationContext()
: Pass the application context as the first argument. You can also usethis
if you are inside an activity."This is a short-duration Toast"
: This is the message you want to display in theToast
.Toast.LENGTH_SHORT
: This specifies the duration of theToast
. You can also useToast.LENGTH_LONG
for a longer duration.
To display a Toast
, you can call the show()
method on the Toast
object. The message will appear at the bottom of the screen and automatically disappear after the specified duration.
Here’s a complete example of displaying a Toast
when a button is clicked:
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.Toast;
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Button button = findViewById(R.id.button);
button.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// Display a short-duration Toast when the button is clicked
Toast.makeText(getApplicationContext(), "Button clicked!", Toast.LENGTH_SHORT).show();
}
});
}
}
We set an OnClickListener
on a button. When the button is clicked, a Toast
with the message “Button clicked!” is displayed for a short duration.
You can customize the content, duration, and position of the Toast
as needed for your app’s requirements.