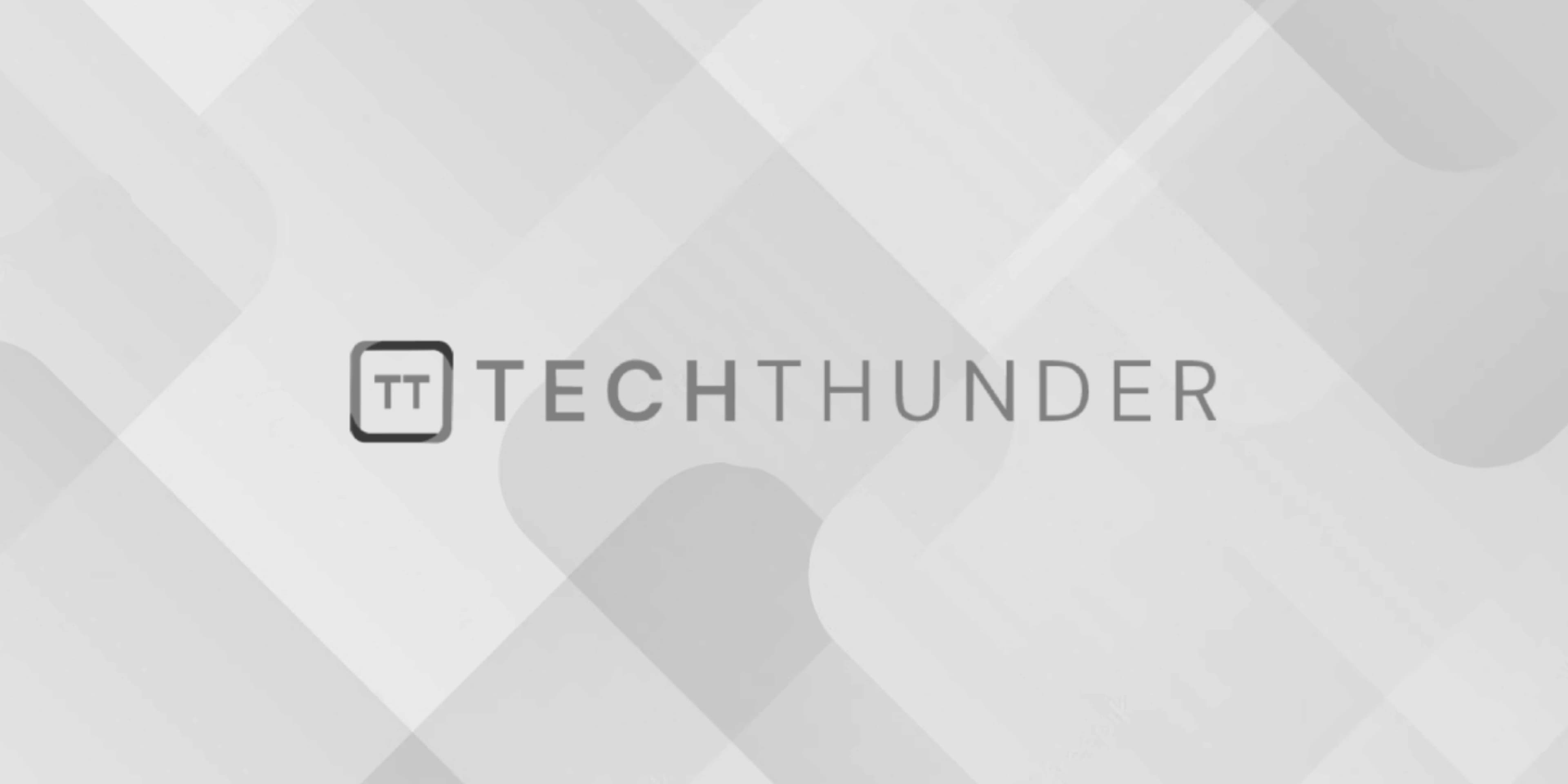
Get Call State in Android
The Android can monitor the call state using a PhoneStateListener
. This allows your app to receive callbacks when the phone call state changes, such as when a call is incoming, outgoing, or idle. Here’s how to get the call state in Android:
1. Create a PhoneStateListener
You need to create a class that extends PhoneStateListener
and override its onCallStateChanged
method to receive call state updates.
import android.telephony.PhoneStateListener;
import android.telephony.TelephonyManager;
public class MyPhoneStateListener extends PhoneStateListener {
@Override
public void onCallStateChanged(int state, String phoneNumber) {
super.onCallStateChanged(state, phoneNumber);
switch (state) {
case TelephonyManager.CALL_STATE_IDLE:
// Call is idle (no call)
break;
case TelephonyManager.CALL_STATE_RINGING:
// Incoming call
// The phoneNumber parameter contains the caller's number
break;
case TelephonyManager.CALL_STATE_OFFHOOK:
// Call is in progress (outgoing or incoming)
break;
}
}
}
2. Register the PhoneStateListener
You should register your PhoneStateListener
in your activity or service to start receiving call state updates. You can do this in the onCreate
method of your activity or the onCreate
method of your service.
import android.Manifest;
import android.content.pm.PackageManager;
import android.os.Bundle;
import android.telephony.TelephonyManager;
import androidx.annotation.NonNull;
import androidx.appcompat.app.AppCompatActivity;
import androidx.core.app.ActivityCompat;
public class MainActivity extends AppCompatActivity {
private static final int PERMISSION_REQUEST_CODE = 123;
private MyPhoneStateListener phoneStateListener;
private TelephonyManager telephonyManager;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// Request the CALL_PHONE permission if not granted
if (ActivityCompat.checkSelfPermission(this, Manifest.permission.READ_PHONE_STATE)
!= PackageManager.PERMISSION_GRANTED) {
ActivityCompat.requestPermissions(this,
new String[]{Manifest.permission.READ_PHONE_STATE}, PERMISSION_REQUEST_CODE);
}
// Initialize the TelephonyManager and MyPhoneStateListener
telephonyManager = (TelephonyManager) getSystemService(TELEPHONY_SERVICE);
phoneStateListener = new MyPhoneStateListener();
// Register the PhoneStateListener
telephonyManager.listen(phoneStateListener, PhoneStateListener.LISTEN_CALL_STATE);
}
// Handle permission request result
@Override
public void onRequestPermissionsResult(int requestCode, @NonNull String[] permissions,
@NonNull int[] grantResults) {
if (requestCode == PERMISSION_REQUEST_CODE) {
if (grantResults.length > 0 && grantResults[0] == PackageManager.PERMISSION_GRANTED) {
// Permission granted, you can now register the PhoneStateListener
telephonyManager.listen(phoneStateListener, PhoneStateListener.LISTEN_CALL_STATE);
} else {
// Permission denied, handle accordingly
}
}
}
}
3. Unregister the PhoneStateListener
Don’t forget to unregister your PhoneStateListener
when you’re done to prevent resource leaks. You can do this in the onDestroy
method of your activity or service.
@Override
protected void onDestroy() {
super.onDestroy();
telephonyManager.listen(phoneStateListener, PhoneStateListener.LISTEN_NONE);
}
With this setup, your app will receive call state updates through the onCallStateChanged
method of your MyPhoneStateListener
. You can then take actions or update your app’s UI based on the call state.