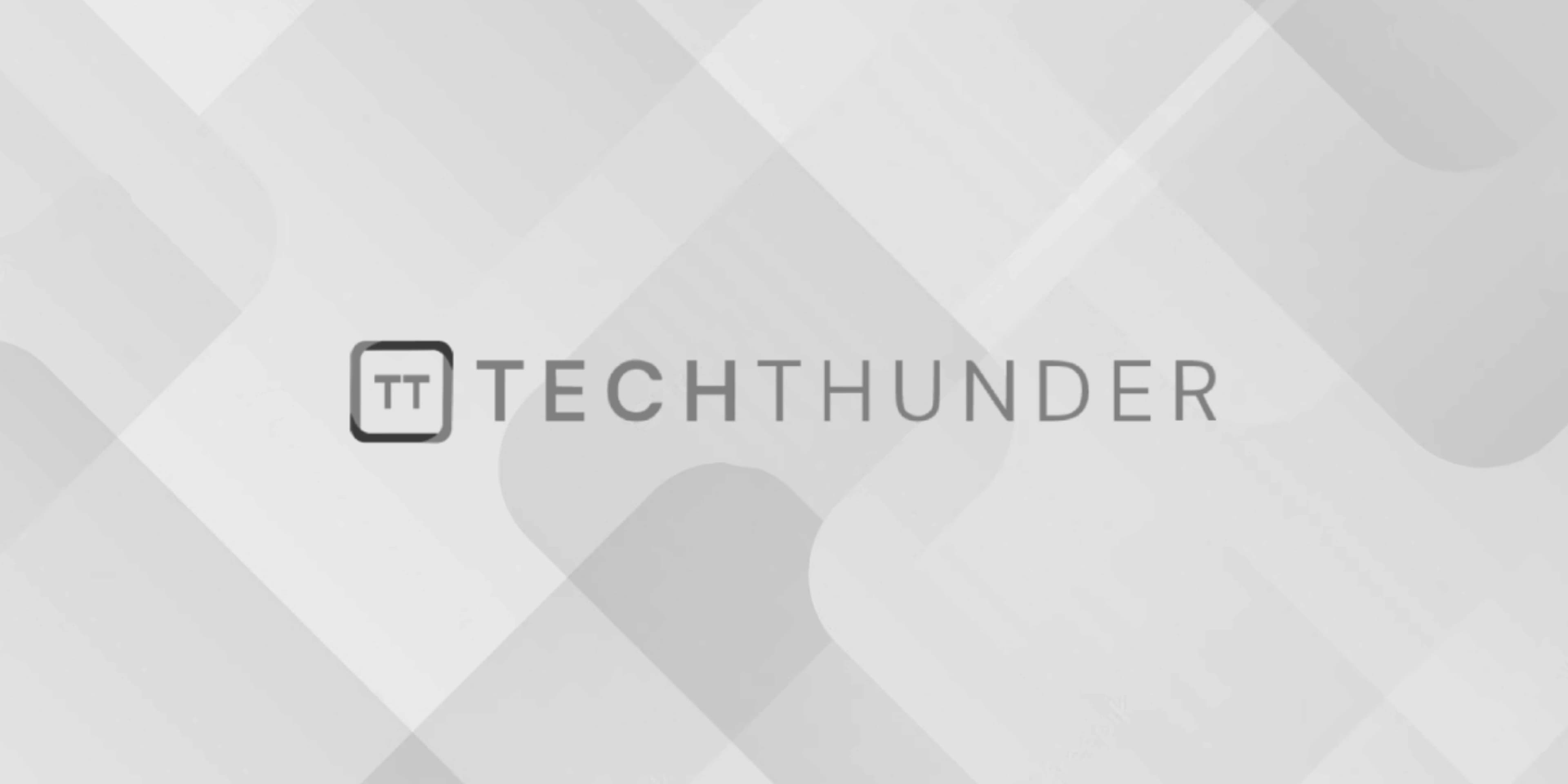
Swipe Refresh Activity in Android
A SwipeRefreshLayout is a common UI pattern in Android applications that allows users to trigger a refresh of a content view by swiping down from the top of the screen. To implement a SwipeRefreshLayout in your Android app, follow these steps:
- Add the SwipeRefreshLayout to Your Layout XML: Open your layout XML file (e.g.,
activity_main.xml
) and wrap the content you want to refresh with aSwipeRefreshLayout
element. Here’s an example:
<?xml version="1.0" encoding="utf-8"?>
<androidx.swiperefreshlayout.widget.SwipeRefreshLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/swipeRefreshLayout"
android:layout_width="match_parent"
android:layout_height="match_parent">
<!-- Your content goes here, e.g., a RecyclerView or ScrollView -->
<androidx.recyclerview.widget.RecyclerView
android:id="@+id/recyclerView"
android:layout_width="match_parent"
android:layout_height="match_parent" />
</androidx.swiperefreshlayout.widget.SwipeRefreshLayout>
- Initialize SwipeRefreshLayout in Your Activity/Fragment: In your activity or fragment where you want to use the SwipeRefreshLayout, find the SwipeRefreshLayout view and set up the refresh listener. For example, in an activity:
SwipeRefreshLayout swipeRefreshLayout = findViewById(R.id.swipeRefreshLayout);
RecyclerView recyclerView = findViewById(R.id.recyclerView);
// Set up the refresh listener
swipeRefreshLayout.setOnRefreshListener(() -> {
// Perform the refresh operation here (e.g., fetch new data)
fetchData();
// Stop the refreshing animation when done
swipeRefreshLayout.setRefreshing(false);
});
In this code, fetchData()
represents the operation you want to perform when the user triggers a refresh. When the operation is complete, you should call setRefreshing(false)
to stop the refreshing animation.
- Handle Refresh Operation: Inside the
fetchData()
method, you should perform the data refresh operation. This may involve fetching new data from a server, updating a database, or any other operation relevant to your app.
private void fetchData() {
// Simulate fetching new data
new Handler().postDelayed(() -> {
// Update your data source here
// For example, if you're using a RecyclerView, notify the adapter of data changes
adapter.notifyDataSetChanged();
}, 2000); // Delayed for 2 seconds to simulate network request
}
In this example, we simulate fetching new data with a delay of 2 seconds. In a real app, you would replace this code with the actual logic to fetch and update your data.
- Permission Handling and Network Calls: Ensure that you have the necessary permissions for network access (e.g., INTERNET permission) and handle network operations on a background thread to avoid blocking the main UI thread.
- Customize SwipeRefreshLayout (Optional): You can customize the appearance of the SwipeRefreshLayout, such as changing the refresh indicator color or style. To do this, use attributes like
app:layout_behavior
andapp:refreshing
. - Testing: Test your SwipeRefreshLayout by running your app and verifying that the refresh operation works as expected when you swipe down from the top of the screen.
By following these steps, you can implement a SwipeRefreshLayout in your Android app to allow users to refresh the content view with a simple swipe gesture. This pattern is commonly used for refreshing lists, grids, or other types of content that may change over time.