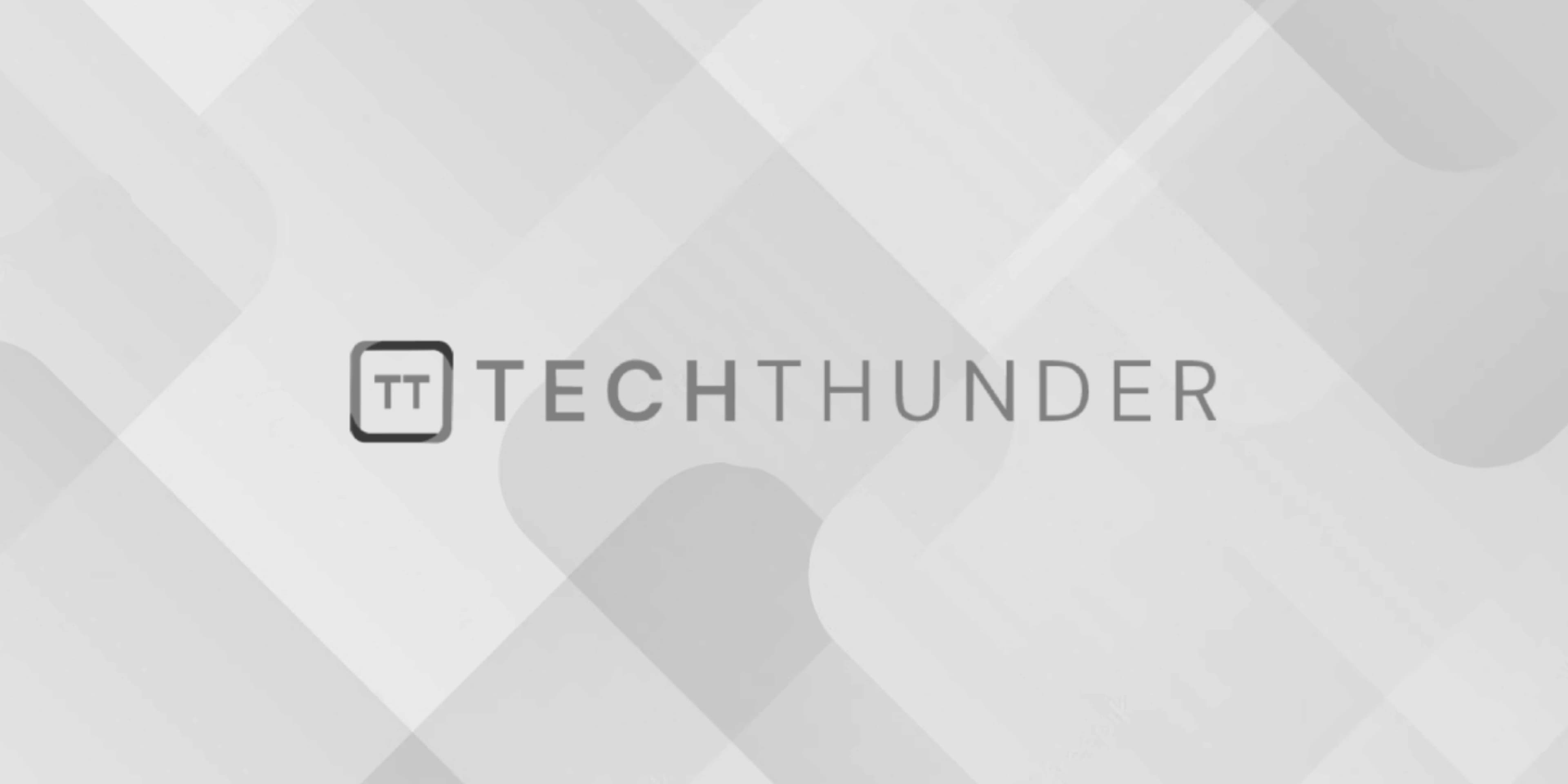
List Paired Devices in Android
The list paired Bluetooth devices in an Android app, you can use the Android Bluetooth API. Here’s a step-by-step guide on how to do it:
1. Add Bluetooth Permissions:
In your AndroidManifest.xml
file, add the necessary permissions to use Bluetooth:
<uses-permission android:name="android.permission.BLUETOOTH" />
<uses-permission android:name="android.permission.BLUETOOTH_ADMIN" />
2. Check Bluetooth Availability:
Before listing paired devices, check if Bluetooth is available and enabled on the device. You can do this programmatically in your activity or fragment:
BluetoothAdapter bluetoothAdapter = BluetoothAdapter.getDefaultAdapter();
if (bluetoothAdapter == null) {
// Device doesn't support Bluetooth
// Handle accordingly
} else {
if (!bluetoothAdapter.isEnabled()) {
// Bluetooth is not enabled; you can prompt the user to enable it
// or do so programmatically with an Intent
} else {
// Bluetooth is available and enabled
// Proceed to list paired devices
}
}
3. List Paired Devices:
You can retrieve the list of paired Bluetooth devices using the BluetoothAdapter
class. Here’s an example of how to do this:
Set<BluetoothDevice> pairedDevices = bluetoothAdapter.getBondedDevices();
if (pairedDevices.size() > 0) {
for (BluetoothDevice device : pairedDevices) {
String deviceName = device.getName();
String deviceAddress = device.getAddress();
// You can display or use the deviceName and deviceAddress as needed
}
}
This code snippet retrieves a set of paired devices and iterates through them, extracting the device name and address.
4. Display the List:
You can display the list of paired devices in your app’s UI, such as in a RecyclerView or a ListView, depending on your design.
Here’s a complete example of an activity that checks for Bluetooth availability, lists paired devices, and displays them in a simple ListView:
import android.bluetooth.BluetoothAdapter;
import android.bluetooth.BluetoothDevice;
import android.content.Intent;
import android.os.Bundle;
import android.widget.ArrayAdapter;
import android.widget.ListView;
import androidx.appcompat.app.AppCompatActivity;
import java.util.ArrayList;
import java.util.List;
import java.util.Set;
public class MainActivity extends AppCompatActivity {
private BluetoothAdapter bluetoothAdapter;
private ListView pairedDevicesListView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
pairedDevicesListView = findViewById(R.id.listView);
bluetoothAdapter = BluetoothAdapter.getDefaultAdapter();
if (bluetoothAdapter == null) {
// Device doesn't support Bluetooth
// Handle accordingly
} else {
if (!bluetoothAdapter.isEnabled()) {
// Bluetooth is not enabled; you can prompt the user to enable it
Intent enableBtIntent = new Intent(BluetoothAdapter.ACTION_REQUEST_ENABLE);
startActivityForResult(enableBtIntent, 1);
} else {
// Bluetooth is available and enabled
listPairedDevices();
}
}
}
private void listPairedDevices() {
Set<BluetoothDevice> pairedDevices = bluetoothAdapter.getBondedDevices();
List<String> deviceNames = new ArrayList<>();
if (pairedDevices.size() > 0) {
for (BluetoothDevice device : pairedDevices) {
String deviceName = device.getName();
String deviceAddress = device.getAddress();
deviceNames.add(deviceName + "\n" + deviceAddress);
}
}
ArrayAdapter<String> adapter = new ArrayAdapter<>(this, android.R.layout.simple_list_item_1, deviceNames);
pairedDevicesListView.setAdapter(adapter);
}
}
The simple ListView is used to display the paired devices. Make sure to create a corresponding XML layout file (e.g., activity_main.xml
) that includes a ListView with the id listView
or update the layout references accordingly.
The Bluetooth operations can be asynchronous, so you should handle Bluetooth-related actions and errors gracefully in your app. Additionally, you can implement click listeners on the paired devices to perform actions such as connecting to a selected device.