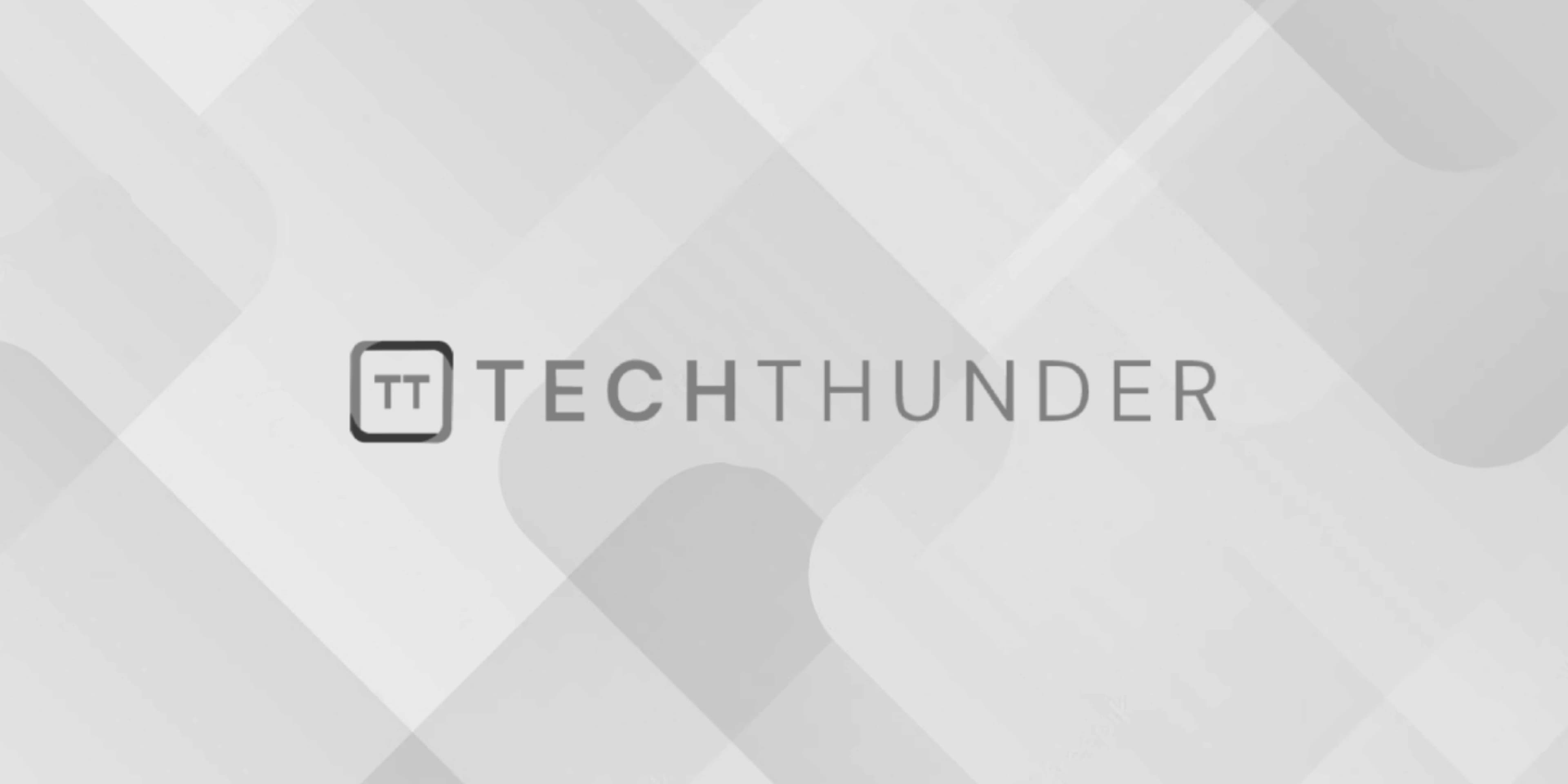
TextToSpeech2 in Android
The Android, the Text-to-Speech (TTS) functionality allows you to convert text into speech, providing accessibility features and enhancing the user experience. Here, I’ll provide a more detailed example of how to use Text-to-Speech in Android.
1. Add TTS Permissions:
In your AndroidManifest.xml
file, add the necessary permissions:
<uses-permission android:name="android.permission.INTERNET" />
<uses-permission android:name="android.permission.ACCESS_NETWORK_STATE" />
<uses-permission android:name="android.permission.MODIFY_AUDIO_SETTINGS" />
2. Initialize TTS Engine:
In your activity or fragment, you need to initialize the Text-to-Speech engine and check if it’s available. You should also implement the TextToSpeech.OnInitListener
interface to handle the initialization process.
import android.os.Bundle;
import android.speech.tts.TextToSpeech;
import android.speech.tts.TextToSpeech.OnInitListener;
import android.util.Log;
import androidx.annotation.Nullable;
import androidx.appcompat.app.AppCompatActivity;
import java.util.Locale;
public class TTSActivity extends AppCompatActivity implements OnInitListener {
private TextToSpeech textToSpeech;
@Override
protected void onCreate(@Nullable Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_tts);
textToSpeech = new TextToSpeech(this, this);
}
@Override
public void onInit(int status) {
if (status == TextToSpeech.SUCCESS) {
// TTS engine is initialized successfully
int result = textToSpeech.setLanguage(Locale.US); // Set your desired language here
if (result == TextToSpeech.LANG_MISSING_DATA || result == TextToSpeech.LANG_NOT_SUPPORTED) {
// Handle the case where the desired language is not available or not supported
Log.e("TTS", "Language not supported");
} else {
// TTS engine is ready for speech synthesis
String textToSpeak = "Hello, I am Text-to-Speech!";
textToSpeech.speak(textToSpeak, TextToSpeech.QUEUE_FLUSH, null, null);
}
} else {
// Handle TTS initialization error
Log.e("TTS", "Initialization failed");
}
}
@Override
protected void onDestroy() {
if (textToSpeech != null) {
textToSpeech.stop();
textToSpeech.shutdown();
}
super.onDestroy();
}
}
In this example:
- We initialize the Text-to-Speech engine in the
onCreate
method and passthis
as theOnInitListener
. - In the
onInit
method, we check if the initialization was successful and set the desired language (in this case, English). If the language is not supported, we handle the error. - Finally, we use the
speak
method to synthesize speech from the provided text.
3. Handling Different Languages:
You can change the language by modifying the Locale
used in setLanguage
. For example, to use Spanish, you would use Locale("es")
.
4. Cleanup:
Always remember to stop and shut down the Text-to-Speech engine in the onDestroy
method to release resources.
5. Testing:
Test your TTS implementation on various devices and languages to ensure it works as expected.
This example demonstrates the basic usage of Text-to-Speech in Android. Depending on your app’s requirements, you can customize the language, pitch, speech rate, and add more advanced features like callbacks for speech completion or error handling.