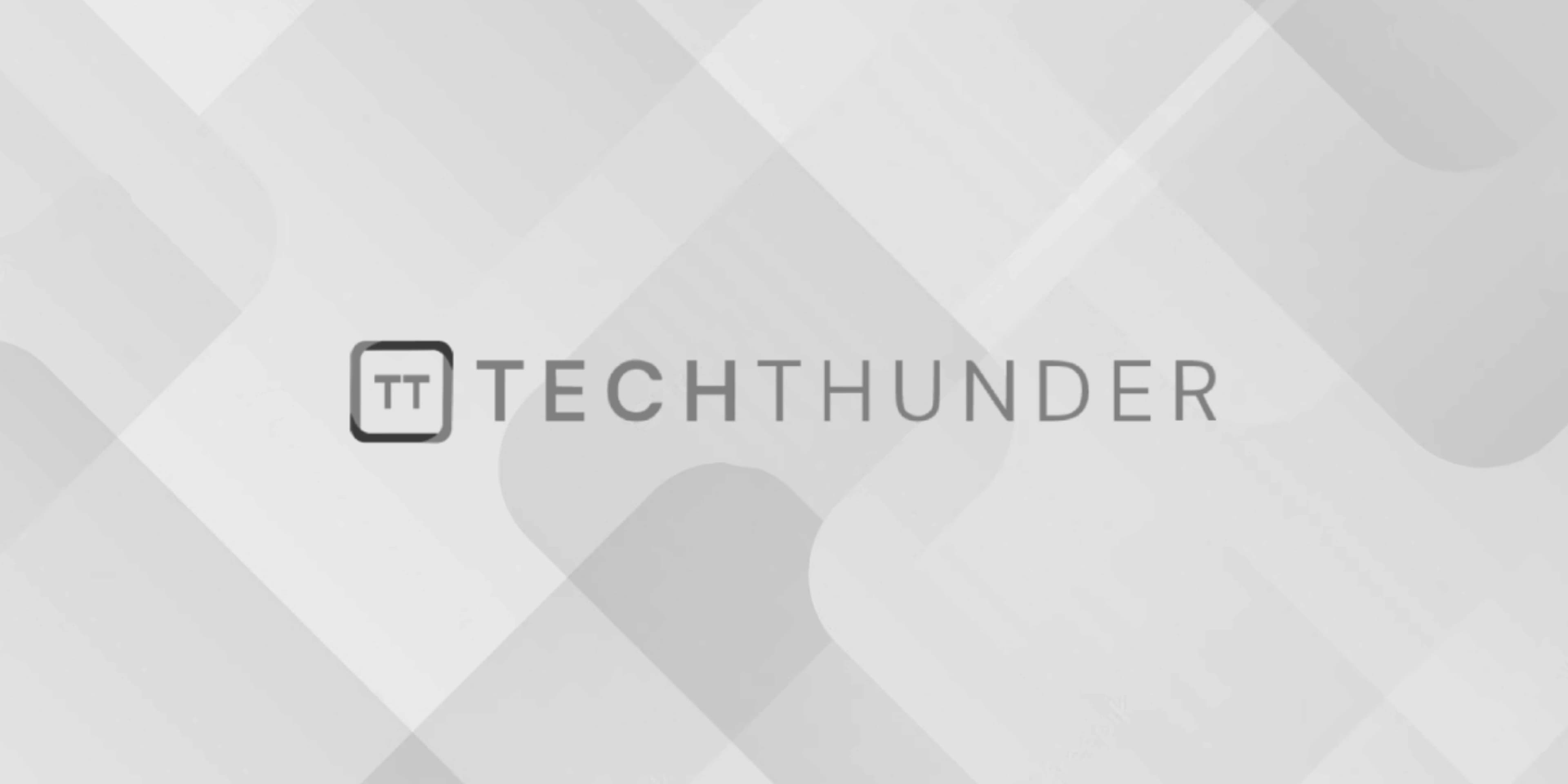
StartActivityForResult in Android
The startActivityForResult()
method is used to start a new activity and receive a result from that activity when it finishes. This is typically used when you want to launch a sub-activity or a child activity to perform a specific task and then receive data or feedback from that sub-activity in the parent activity. The child activity is started with an expectation that it will return a result to the parent activity.
Here’s how startActivityForResult()
is typically used:
- In the Parent Activity:
- You call
startActivityForResult()
to start the child activity, passing anIntent
as a parameter. - You also specify a request code (an integer value) that helps you identify the result when it’s returned.
// Start the child activity
Intent intent = new Intent(this, ChildActivity.class);
startActivityForResult(intent, requestCode);
- In the Child Activity:
- In the child activity, you perform the desired task.
- When the task is complete and you want to send data back to the parent activity, you create an
Intent
and set the result data usingsetResult()
.
// In the child activity when the task is complete
Intent resultIntent = new Intent();
resultIntent.putExtra("key", data);
setResult(RESULT_OK, resultIntent);
finish();
Here, RESULT_OK
indicates that the task was successful, and you can also attach data to the result intent.
- In the Parent Activity:
- The parent activity receives the result in the
onActivityResult()
method, which you need to override. - You can check the request code to identify which child activity has sent the result.
- You can extract the result data from the received
Intent
.
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
super.onActivityResult(requestCode, resultCode, data);
if (requestCode == requestCode) {
if (resultCode == RESULT_OK) {
// Handle the result data from the child activity
String resultData = data.getStringExtra("key");
// Do something with the result data
} else if (resultCode == RESULT_CANCELED) {
// Handle the case where the child activity was canceled
}
}
}
In this method, you can check the request code and the result code to determine whether the task was successful or canceled. You can then extract any relevant data from the received Intent
.
This mechanism allows you to pass data and control flow between different activities in a structured way, making it useful for scenarios like capturing user input, selecting options from a list, or any other multi-step process where you need to communicate between activities.