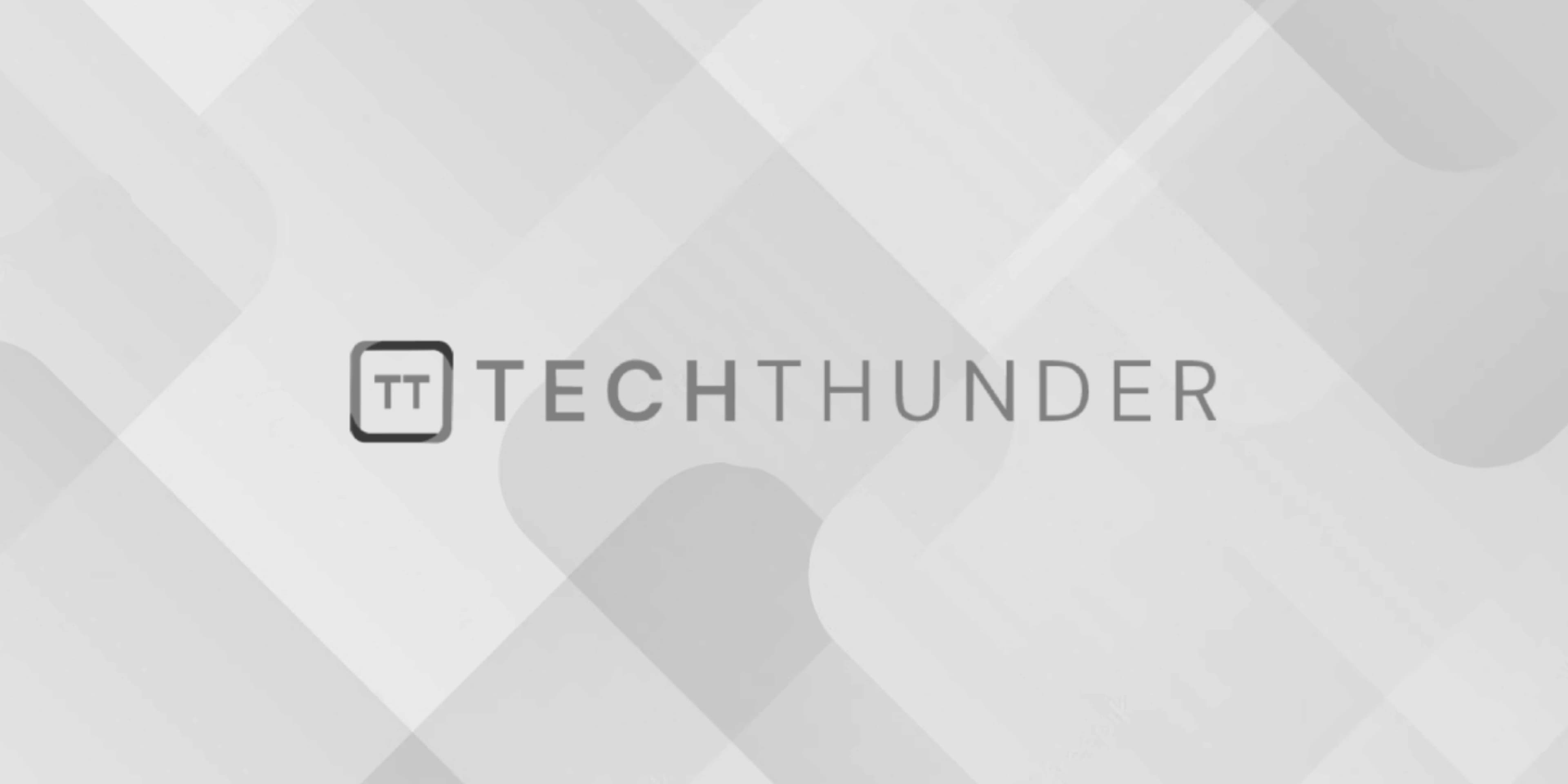
TextToSpeech1 in Android
Text-to-Speech (TTS) in Android allows your app to convert text into spoken words, providing accessibility features and enhancing the user experience. Here’s a step-by-step guide on how to implement TTS in an Android app:
1. Add TTS Permission:
In your AndroidManifest.xml
file, add the following permission to use TTS:
<uses-permission android:name="android.permission.INTERNET" />
2. Check for TTS Engine Availability:
Before using TTS, you should check if a TTS engine is installed on the device:
private TextToSpeech textToSpeech;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
textToSpeech = new TextToSpeech(this, new TextToSpeech.OnInitListener() {
@Override
public void onInit(int status) {
if (status == TextToSpeech.SUCCESS) {
// TTS engine is initialized successfully
} else {
// Handle TTS initialization error
Toast.makeText(MainActivity.this, "TTS initialization failed", Toast.LENGTH_SHORT).show();
}
}
});
}
3. Implement TTS Text Conversion:
You can use the TextToSpeech
object to convert text to speech. For example, you can add a button to your layout and use it to trigger TTS conversion:
Button speakButton = findViewById(R.id.speakButton);
EditText editText = findViewById(R.id.editText);
speakButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
String textToRead = editText.getText().toString();
if (!TextUtils.isEmpty(textToRead)) {
textToSpeech.speak(textToRead, TextToSpeech.QUEUE_FLUSH, null, null);
}
}
});
In this example, we use the speak
method to convert the text from an EditText
widget to speech. You can adapt this code to your specific use case.
4. Handle TTS Engine Language:
You can set the language for TTS output:
Locale desiredLocale = Locale.US; // Change this to the desired locale
int result = textToSpeech.setLanguage(desiredLocale);
if (result == TextToSpeech.LANG_MISSING_DATA || result == TextToSpeech.LANG_NOT_SUPPORTED) {
// Handle the case where the desired language is not available or not supported
}
5. Shut Down TTS Engine:
It’s essential to release the TTS engine when you’re done using it to free up resources:
@Override
protected void onDestroy() {
if (textToSpeech != null) {
textToSpeech.stop();
textToSpeech.shutdown();
}
super.onDestroy();
}
6. Testing:
Test your TTS implementation on various devices and language settings to ensure it works as expected.
This basic example covers the essentials of implementing TTS in an Android app. You can further customize TTS settings, handle pitch and speech rate, and provide more advanced features to enhance the user experience.