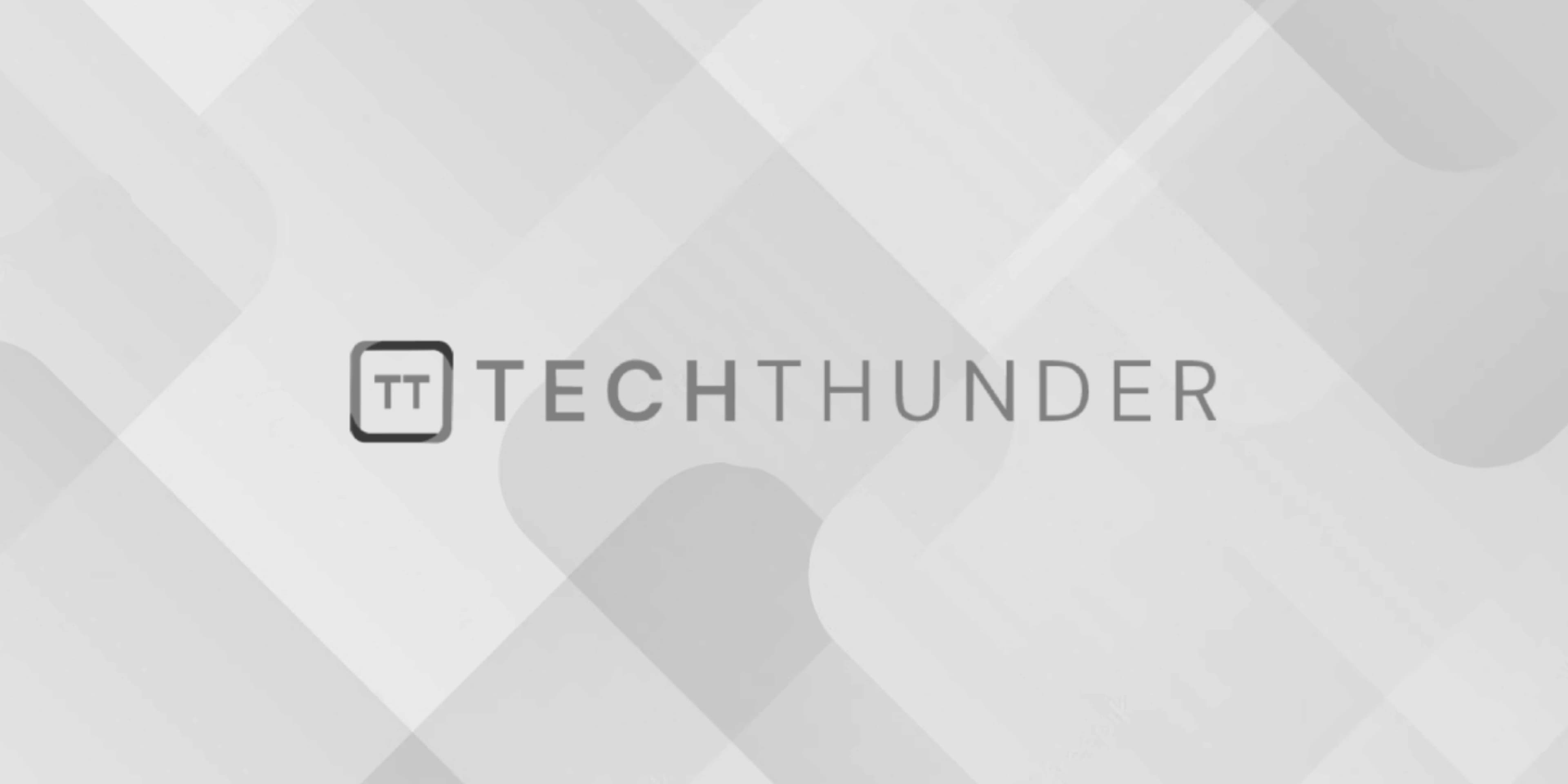
JSON Parsing in Android
JSON parsing in Android allows you to retrieve and manipulate data from JSON (JavaScript Object Notation) responses typically received from web services, APIs, or local JSON files. Here’s a step-by-step guide on how to parse JSON in Android:
1. Get JSON Data:
You need JSON data to parse. This data can come from various sources, such as a web API, a local file, or a network response.
2. Choose a JSON Parsing Approach:
Android provides multiple ways to parse JSON data, including:
- JSONObject and JSONArray: These classes are part of the Android SDK and allow you to parse JSON data in a straightforward manner.
- Gson Library: Google’s Gson library simplifies JSON parsing by automatically mapping JSON objects to Java objects and vice versa.
- Jackson Library: The Jackson library is another popular choice for JSON parsing and object mapping.
- Volley or Retrofit: These networking libraries can handle JSON parsing as part of their request/response processing.
3. Parse JSON Data:
Here’s how to parse JSON using the built-in JSONObject and JSONArray classes:
try {
String jsonString = "{ \"name\": \"John\", \"age\": 30, \"city\": \"New York\" }";
JSONObject jsonObject = new JSONObject(jsonString);
String name = jsonObject.getString("name");
int age = jsonObject.getInt("age");
String city = jsonObject.getString("city");
// Now you can use the parsed data (name, age, city) in your app
} catch (JSONException e) {
e.printStackTrace();
// Handle JSON parsing error
}
For parsing JSON arrays, you can use JSONArray:
try {
String jsonArrayString = "[{\"name\": \"John\"}, {\"name\": \"Alice\"}]";
JSONArray jsonArray = new JSONArray(jsonArrayString);
for (int i = 0; i < jsonArray.length(); i++) {
JSONObject item = jsonArray.getJSONObject(i);
String name = item.getString("name");
// Use the parsed data (name) as needed
}
} catch (JSONException e) {
e.printStackTrace();
// Handle JSON parsing error
}
4. Error Handling:
Always handle exceptions that may occur during JSON parsing. JSON parsing can fail if the JSON data is malformed or doesn’t match your expectations. Handling errors gracefully is essential to prevent app crashes.
5. Integrate with Network Requests:
If you’re fetching JSON data from a web service or API, you should use a networking library like Volley, Retrofit, or OkHttp to make the network request and parse the JSON response. These libraries often include features for background threading, caching, and error handling.
6. Model Classes (Optional):
For more complex JSON structures, you can create Java classes that match the JSON structure and use a library like Gson or Jackson to automatically map JSON data to Java objects. This is especially useful when dealing with nested JSON data.
7. Display Data:
Once you’ve parsed the JSON data, you can display it in your app’s UI or use it for various purposes, such as populating lists, updating UI components, or performing calculations.
8. Test:
Always thoroughly test your JSON parsing code with various JSON data formats to ensure robustness and correctness.
JSON parsing is a fundamental skill when working with web services and APIs in Android apps. Depending on your project’s complexity, you can choose the JSON parsing approach that best suits your needs, whether it’s simple manual parsing with JSONObject and JSONArray or using a library for more advanced scenarios.