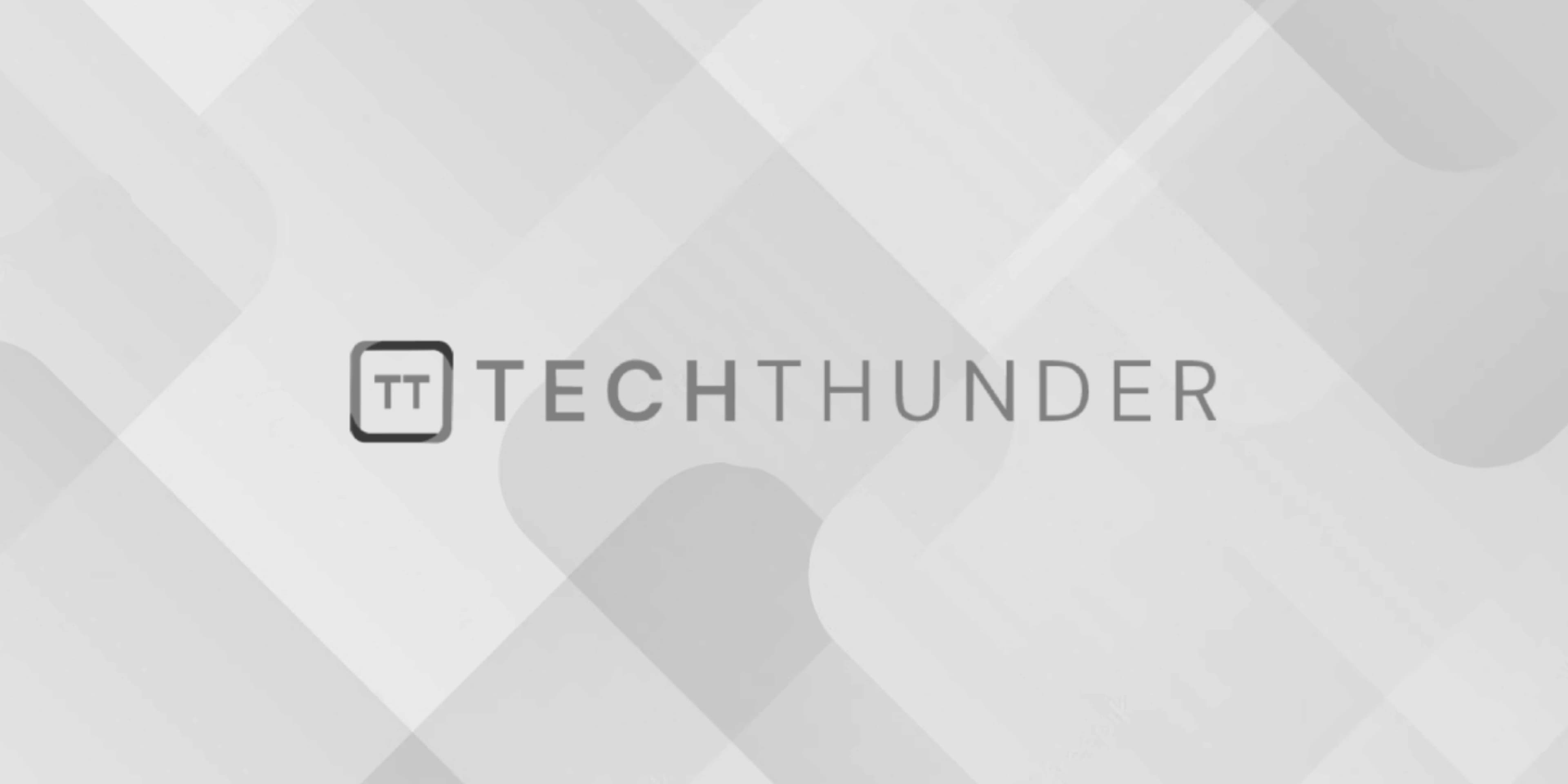
Bluetooth Tutorial in Android
Using Bluetooth in an Android app allows you to communicate with other devices wirelessly. This tutorial will guide you through the process of implementing Bluetooth functionality in your Android app:
1. Add Bluetooth Permissions:
In your AndroidManifest.xml
file, add the necessary permissions to use Bluetooth:
<uses-permission android:name="android.permission.BLUETOOTH" />
<uses-permission android:name="android.permission.BLUETOOTH_ADMIN" />
<uses-permission android:name="android.permission.BLUETOOTH_CONNECT" />
<uses-permission android:name="android.permission.BLUETOOTH_SCAN" />
<uses-permission android:name="android.permission.BLUETOOTH_ADVERTISE" />
Make sure to request these permissions at runtime for devices running Android 6.0 (API level 23) or higher.
2. Check Bluetooth Availability:
Before using Bluetooth, check if it’s available and enabled on the device:
BluetoothAdapter bluetoothAdapter = BluetoothAdapter.getDefaultAdapter();
if (bluetoothAdapter == null) {
// Device doesn't support Bluetooth
// Handle accordingly
} else {
if (!bluetoothAdapter.isEnabled()) {
// Bluetooth is not enabled; you can prompt the user to enable it
Intent enableBtIntent = new Intent(BluetoothAdapter.ACTION_REQUEST_ENABLE);
startActivityForResult(enableBtIntent, REQUEST_ENABLE_BT);
} else {
// Bluetooth is available and enabled
// Proceed with Bluetooth operations
}
}
3. Discover Nearby Devices:
To discover nearby Bluetooth devices, you can use the BluetoothAdapter’s startDiscovery
method or use Bluetooth Low Energy (BLE) scanning APIs for BLE devices. Here’s an example using classic Bluetooth discovery:
// Register a BroadcastReceiver to listen for discovered devices
private final BroadcastReceiver discoveryReceiver = new BroadcastReceiver() {
@Override
public void onReceive(Context context, Intent intent) {
String action = intent.getAction();
if (BluetoothDevice.ACTION_FOUND.equals(action)) {
BluetoothDevice device = intent.getParcelableExtra(BluetoothDevice.EXTRA_DEVICE);
// Handle the discovered device
}
}
};
// Start device discovery
if (bluetoothAdapter.startDiscovery()) {
// Discovery started successfully
// Register the BroadcastReceiver to receive discovered devices
IntentFilter filter = new IntentFilter(BluetoothDevice.ACTION_FOUND);
registerReceiver(discoveryReceiver, filter);
} else {
// Discovery failed to start; handle accordingly
}
Remember to unregister the BroadcastReceiver when you’re done with device discovery.
4. Pairing with a Device:
To pair with a discovered Bluetooth device, you can use the BluetoothDevice.createBond
method:
BluetoothDevice device = ... // Get the BluetoothDevice you want to pair with
device.createBond();
You can listen for pairing events using a BroadcastReceiver registered with the BluetoothDevice.ACTION_BOND_STATE_CHANGED
action.
5. Connecting to a Device:
To establish a Bluetooth connection with another device, you can use BluetoothSocket for classic Bluetooth or GATT (Generic Attribute Profile) for BLE devices. Here’s a simplified example for classic Bluetooth:
BluetoothDevice device = ... // Get the BluetoothDevice you want to connect to
BluetoothSocket socket = device.createRfcommSocketToServiceRecord(MY_UUID);
try {
socket.connect();
// Connection successful; perform data exchange
// Don't forget to close the socket when done
} catch (IOException e) {
// Connection failed; handle accordingly
}
6. Exchange Data:
Once a connection is established, you can exchange data with the connected device using input and output streams. For example:
OutputStream outputStream = socket.getOutputStream();
outputStream.write("Hello, Bluetooth!".getBytes());
InputStream inputStream = socket.getInputStream();
byte[] buffer = new byte[1024];
int bytesRead = inputStream.read(buffer);
String receivedData = new String(buffer, 0, bytesRead);
7. Bluetooth Permissions (Runtime):
For devices running Android 6.0 and above, you need to request runtime permissions for Bluetooth operations. You can use the ActivityCompat.requestPermissions
method to do this.
8. Cleanup:
Remember to clean up resources, such as closing sockets and unregistering BroadcastReceivers, when you’re done with Bluetooth operations to avoid resource leaks.
This tutorial covers the basics of implementing Bluetooth functionality in your Android app. Depending on your use case, you may need to explore more advanced Bluetooth features like Bluetooth Low Energy (BLE) communication, managing multiple connections, or building a Bluetooth server.