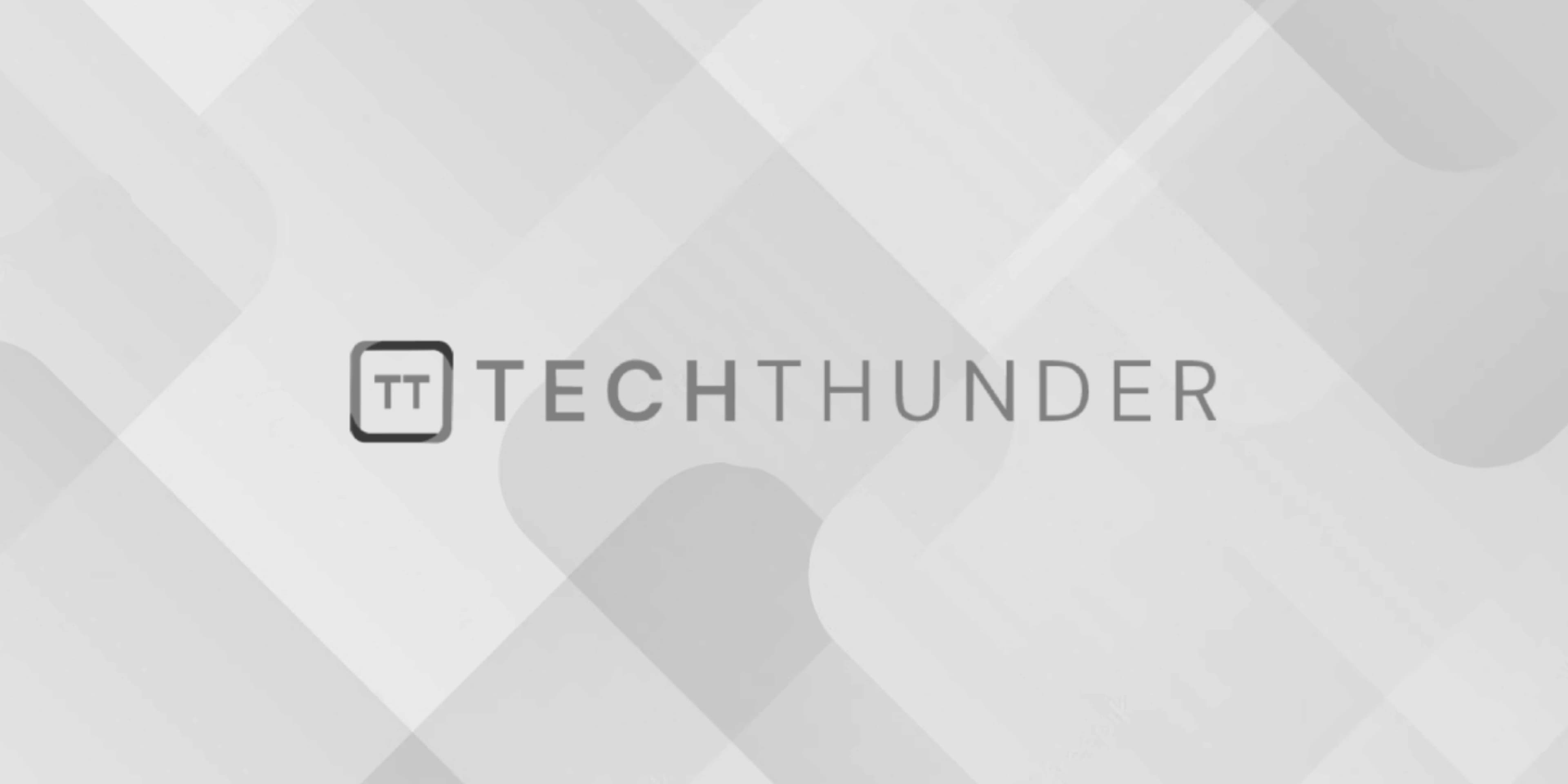
170 views
AutoCompleteTextView in Android
The AutoCompleteTextView
in Android is a specialized EditText
widget that provides auto-suggestions or auto-completion to users as they type. It is commonly used when you have a large dataset or a list of suggestions that you want to display to users while they are typing. Here’s how to use AutoCompleteTextView
in your Android app:
- Add an
AutoCompleteTextView
to Your Layout: In your XML layout file, add anAutoCompleteTextView
element where you want to include the auto-suggest input field:
XML
<AutoCompleteTextView
android:id="@+id/autoCompleteTextView"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="Type here..."
/>
android:id
: Assign a unique ID to theAutoCompleteTextView
for later reference.android:layout_width
andandroid:layout_height
: Adjust these attributes based on your layout requirements.android:hint
: Set the hint text that appears in the field before the user starts typing.
- Define Data Source: In your activity or fragment, define the data source for the auto-suggestions. This can be an array of strings, a list of objects, or any other data source. For example, let’s say you have an array of strings:
Java
String[] suggestions = {"Apple", "Banana", "Cherry", "Date", "Fig", "Grape", "Lemon", "Mango", "Orange", "Peach"};
- Create an ArrayAdapter: Create an
ArrayAdapter
to bind the data source to theAutoCompleteTextView
. TheArrayAdapter
is responsible for showing suggestions as the user types.
Java
ArrayAdapter<String> adapter = new ArrayAdapter<>(this, android.R.layout.simple_dropdown_item_1line, suggestions);
this
: Pass the current context (usually the activity).android.R.layout.simple_dropdown_item_1line
: This is a built-in layout resource provided by Android for displaying the suggestions in a dropdown style.
- Set the Adapter: Apply the adapter to the
AutoCompleteTextView
:
Java
AutoCompleteTextView autoCompleteTextView = findViewById(R.id.autoCompleteTextView);
autoCompleteTextView.setAdapter(adapter);
- Run Your App: When you run your app, the
AutoCompleteTextView
will display suggestions to the user as they type. Users can select an item from the dropdown or continue typing. - Handle Item Selection (Optional): If you want to perform an action when an item is selected from the auto-suggestions, you can set an
OnItemClickListener
:
Java
autoCompleteTextView.setOnItemClickListener(new AdapterView.OnItemClickListener() {
@Override
public void onItemClick(AdapterView<?> parent, View view, int position, long id) {
String selectedText = (String) parent.getItemAtPosition(position);
// Do something with the selected text
}
});
In the onItemClick
method, you can access the selected item using parent.getItemAtPosition(position)
.
You can easily implement an AutoCompleteTextView
in your Android app to provide auto-suggestions to users as they type, making it easier for them to input data efficiently.