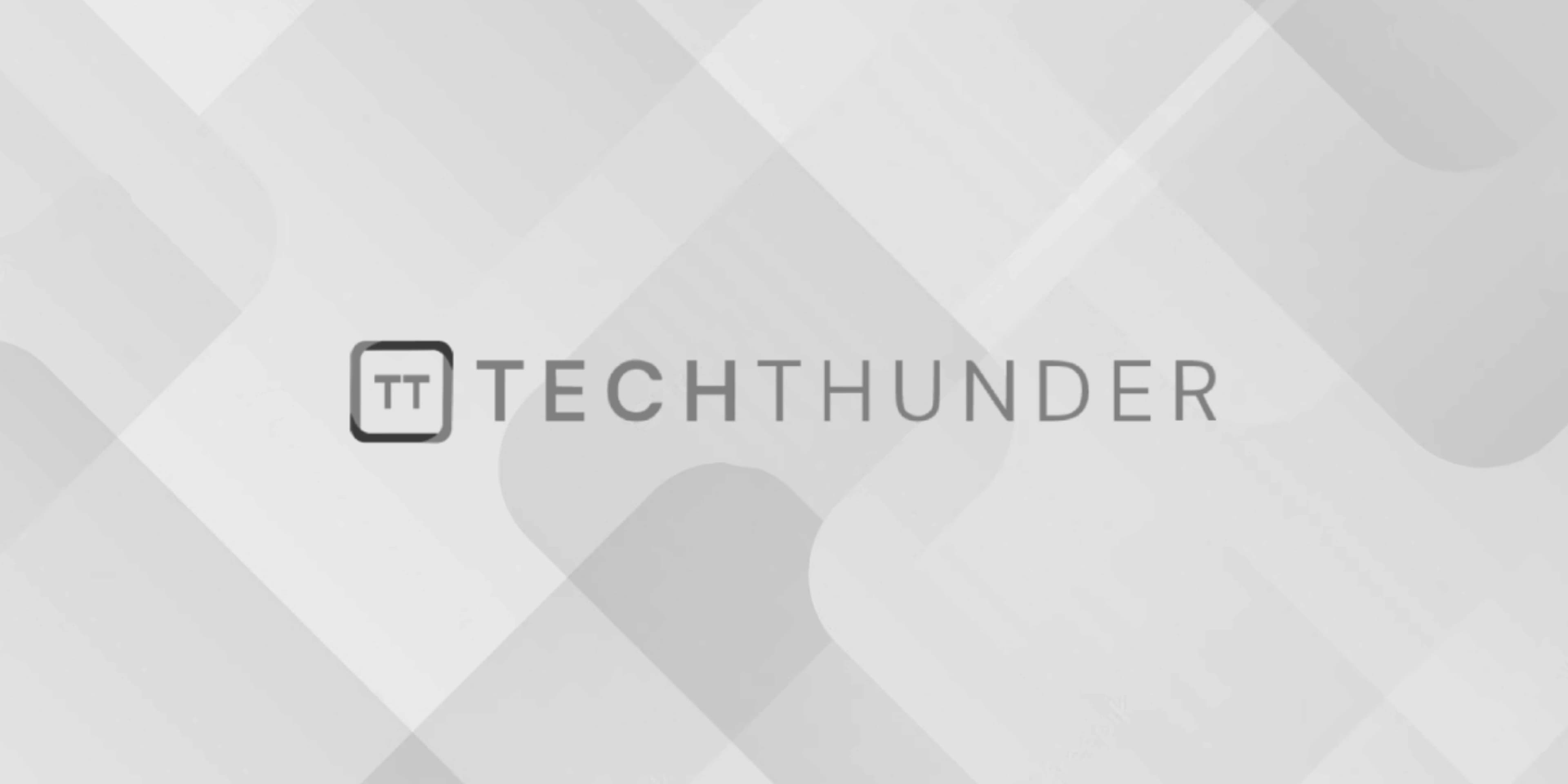
External Storage in Android
External storage in Android refers to a secondary storage medium that is not part of the device’s built-in internal storage. It can be a physical SD card or any other removable storage device. External storage provides a place to store files that can be accessed by multiple apps and can persist even if the app is uninstalled. Here’s how to work with external storage in Android:
1. Check External Storage Availability:
Before using external storage, it’s essential to check if it’s available and accessible. You can do this with the following code:
String state = Environment.getExternalStorageState();
if (Environment.MEDIA_MOUNTED.equals(state)) {
// External storage is available and can be read or written
} else if (Environment.MEDIA_MOUNTED_READ_ONLY.equals(state)) {
// External storage is available in read-only mode
} else {
// External storage is not available
}
2. Request Permissions:
If your app targets Android 6.0 (API level 23) or higher, and you intend to read or write to external storage, you need to request runtime permissions from the user.
// Example: Request write permission
if (ContextCompat.checkSelfPermission(this, Manifest.permission.WRITE_EXTERNAL_STORAGE) != PackageManager.PERMISSION_GRANTED) {
ActivityCompat.requestPermissions(this, new String[]{Manifest.permission.WRITE_EXTERNAL_STORAGE}, REQUEST_CODE);
}
Make sure to handle permission requests and responses appropriately.
3. Write to External Storage:
To write data to external storage, you need to specify the path to the directory where you want to create or store files. Here’s an example of writing a file to the external storage’s public directory:
String filename = "example.txt";
String content = "Hello, world!";
File dir = Environment.getExternalStoragePublicDirectory(Environment.DIRECTORY_DOCUMENTS);
File file = new File(dir, filename);
try {
FileOutputStream fos = new FileOutputStream(file);
fos.write(content.getBytes());
fos.close();
} catch (IOException e) {
e.printStackTrace();
}
4. Read from External Storage:
To read data from external storage, locate the file using its path and read its contents. Here’s an example:
String filename = "example.txt";
File dir = Environment.getExternalStoragePublicDirectory(Environment.DIRECTORY_DOCUMENTS);
File file = new File(dir, filename);
try {
FileInputStream fis = new FileInputStream(file);
InputStreamReader isr = new InputStreamReader(fis);
BufferedReader br = new BufferedReader(isr);
StringBuilder sb = new StringBuilder();
String line;
while ((line = br.readLine()) != null) {
sb.append(line);
}
String content = sb.toString();
br.close();
isr.close();
fis.close();
} catch (IOException e) {
e.printStackTrace();
}
5. Delete from External Storage:
To delete a file from external storage, use the delete
method:
String filename = "example.txt";
File dir = Environment.getExternalStoragePublicDirectory(Environment.DIRECTORY_DOCUMENTS);
File file = new File(dir, filename);
boolean deleted = file.delete();
if (deleted) {
// File deleted successfully
} else {
// File not found or unable to delete
}
6. Directory Paths:
The Environment
class provides constants for various public directory paths on external storage. For example, Environment.DIRECTORY_DOCUMENTS
represents the “Documents” directory.
7. Be Mindful of Permissions and Security:
Be aware of security considerations when working with external storage, as it is shared among apps and the user. Sensitive data should be stored securely, and you should respect user privacy and permissions.
8. Handle Errors and Exceptions:
Make sure to handle exceptions, such as IOException
, that may occur when reading or writing to external storage.
9. Backup and Restore:
Consider implementing backup and restore functionality for your app’s data stored in external storage, as it may be lost during device resets or data wipes.
Working with external storage in Android allows you to store data that is accessible and shareable among apps and persists even after app uninstallation. However, it comes with responsibilities regarding data privacy and security.