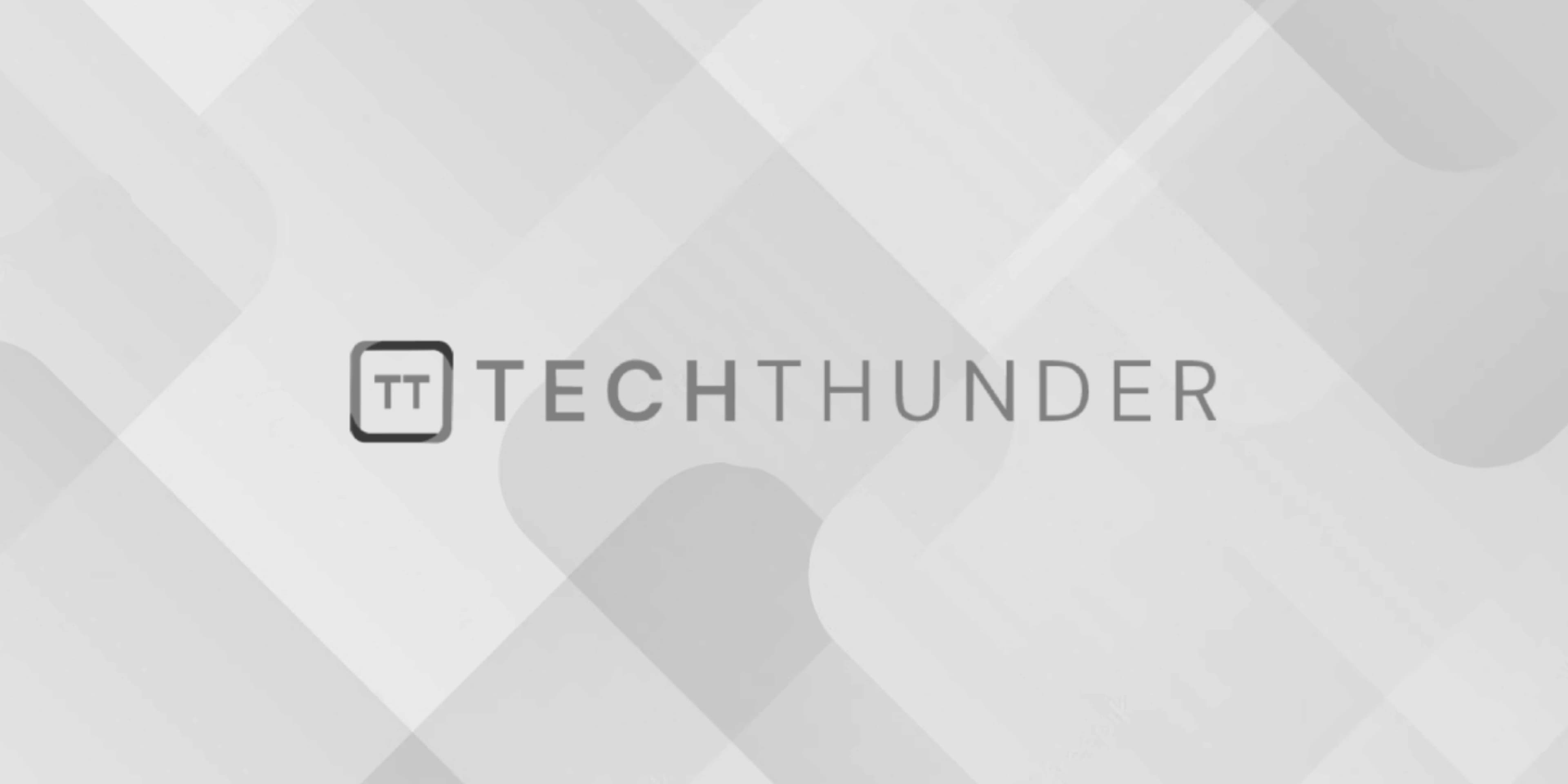
Camera Tutorial in Android
The camera in an Android app allows you to capture photos and record videos, which can be a valuable feature for various types of applications. Here’s a step-by-step tutorial on how to integrate the camera into your Android app:
Creating a camera application in Android involves several steps, including obtaining permissions, setting up the camera preview, capturing images, and handling camera events. Below is a basic outline for creating a simple camera application in Android.
Step 1: Add Permissions to AndroidManifest.xml
Ensure that you have the necessary permissions in your AndroidManifest.xml
file:
<uses-feature android:name="android.hardware.camera" />
<uses-permission android:name="android.permission.CAMERA" />
<uses-feature android:name="android.hardware.camera.autofocus" />
Step 2: Design the Layout (activity_main.xml)
Create a layout file for your main activity (e.g., res/layout/activity_main.xml
):
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<SurfaceView
android:id="@+id/surfaceView"
android:layout_width="match_parent"
android:layout_height="match_parent" />
<Button
android:id="@+id/btnCapture"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerHorizontal="true"
android:layout_alignParentBottom="true"
android:text="Capture" />
</RelativeLayout>
Step 3: Implement MainActivity.java
Create the main activity file (e.g., MainActivity.java
):
import android.app.Activity;
import android.content.pm.PackageManager;
import android.hardware.Camera;
import android.os.Bundle;
import android.view.SurfaceHolder;
import android.view.SurfaceView;
import android.view.View;
import android.widget.Button;
import android.widget.Toast;
import java.io.IOException;
public class MainActivity extends Activity implements SurfaceHolder.Callback {
private Camera camera;
private SurfaceView surfaceView;
private SurfaceHolder surfaceHolder;
private Button captureButton;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
surfaceView = findViewById(R.id.surfaceView);
surfaceHolder = surfaceView.getHolder();
surfaceHolder.addCallback(this);
captureButton = findViewById(R.id.btnCapture);
captureButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
captureImage();
}
});
}
private void captureImage() {
if (camera != null) {
camera.takePicture(null, null, new Camera.PictureCallback() {
@Override
public void onPictureTaken(byte[] data, Camera camera) {
// Handle captured image data here
// You can save the image to a file or process it as needed
Toast.makeText(MainActivity.this, "Image Captured!", Toast.LENGTH_SHORT).show();
camera.startPreview(); // Restart preview after capturing
}
});
}
}
@Override
public void surfaceCreated(SurfaceHolder holder) {
try {
camera = Camera.open();
camera.setDisplayOrientation(90); // Adjust orientation as needed
camera.setPreviewDisplay(holder);
camera.startPreview();
} catch (IOException e) {
e.printStackTrace();
}
}
@Override
public void surfaceChanged(SurfaceHolder holder, int format, int width, int height) {
if (surfaceHolder.getSurface() == null) {
return;
}
try {
camera.stopPreview();
camera.setPreviewDisplay(surfaceHolder);
camera.startPreview();
} catch (Exception e) {
e.printStackTrace();
}
}
@Override
public void surfaceDestroyed(SurfaceHolder holder) {
if (camera != null) {
camera.stopPreview();
camera.release();
camera = null;
}
}
private boolean checkCameraHardware() {
if (getPackageManager().hasSystemFeature(PackageManager.FEATURE_CAMERA)) {
return true;
} else {
Toast.makeText(this, "No camera on this device", Toast.LENGTH_SHORT).show();
return false;
}
}
@Override
protected void onResume() {
super.onResume();
if (checkCameraHardware()) {
if (camera == null) {
camera = Camera.open();
camera.setDisplayOrientation(90); // Adjust orientation as needed
}
}
}
@Override
protected void onPause() {
super.onPause();
if (camera != null) {
camera.stopPreview();
camera.release();
camera = null;
}
}
}
This example demonstrates the basics of capturing images using the camera in Android. Make sure to handle permissions properly, and consider using the Camera2 API for newer Android versions.
Remember to replace "YOUR_BANNER_AD_UNIT_ID"
with your actual AdMob unit ID and handle runtime permissions based on the Android version you are targeting.