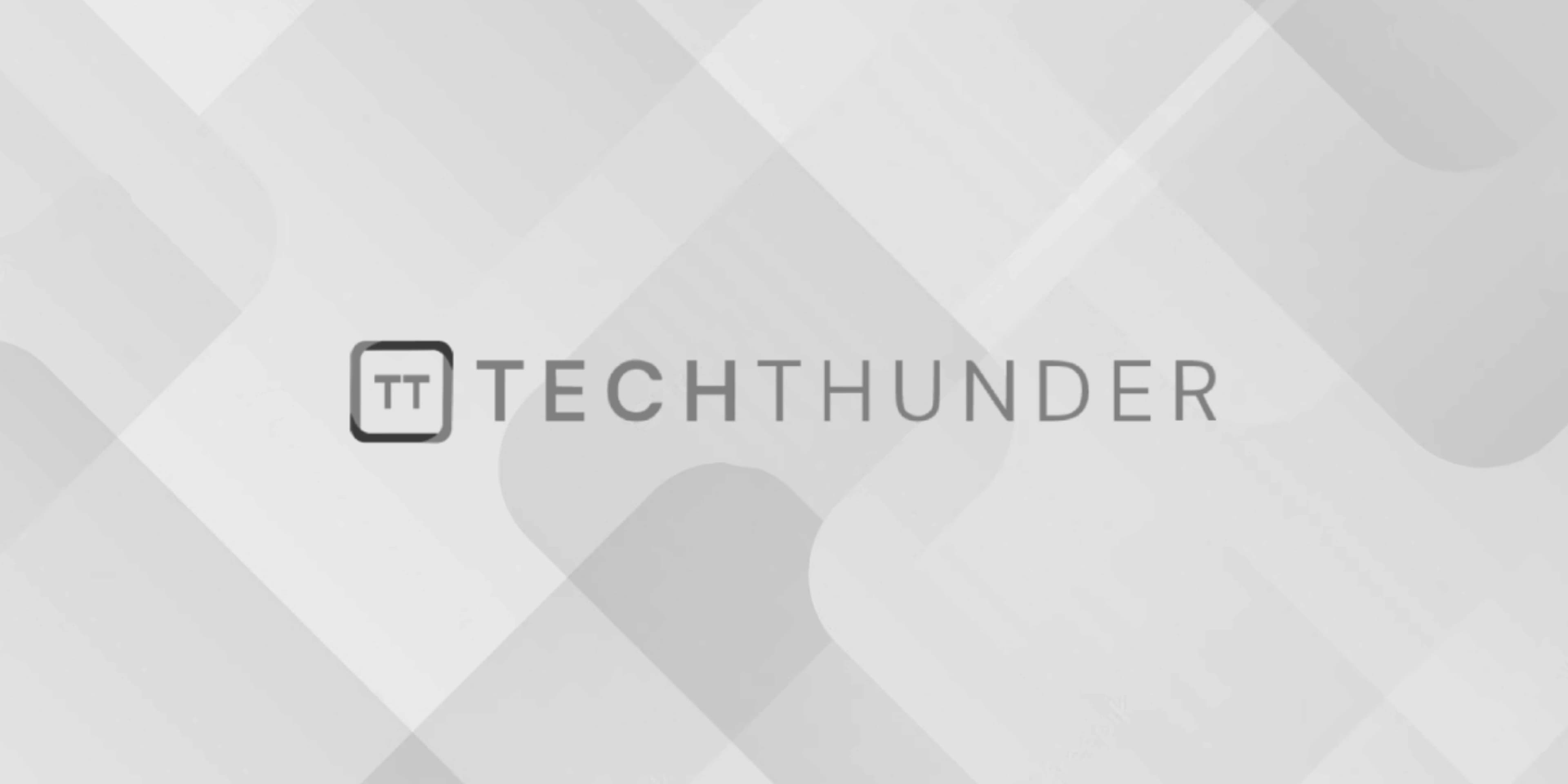
ListView in Android
The Android ListView
is a view group that displays a list of scrollable items. Each item in the list is typically an instance of View
, which can be a TextView
, ImageView
, or any other view type. The ListView
is useful for displaying large sets of data in a structured manner.
Here’s how you can implement a basic ListView
in Android:
- Create an Activity: Start by creating an activity in your Android project where you want to display the
ListView
. - Define a Layout for Each List Item: Create an XML layout file that represents the layout for each item in your
ListView
. This layout file defines the visual appearance and structure of each list item. For example, you can create a file namedlist_item.xml
:
<!-- list_item.xml -->
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical"
android:padding="16dp">
<TextView
android:id="@+id/item_text_view"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textSize="18sp"
android:textColor="@android:color/black" />
</LinearLayout>
In this example, we have a simple LinearLayout
with a TextView
to display text for each list item.
- Create an Adapter: To populate the
ListView
with data, you need an adapter. The adapter acts as a bridge between the data source (e.g., an array, list) and theListView
. You can use an existing adapter likeArrayAdapter
, or you can create a custom adapter. Here’s an example usingArrayAdapter
:
import android.app.Activity;
import android.os.Bundle;
import android.widget.ArrayAdapter;
import android.widget.ListView;
public class MyActivity extends Activity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_my);
// Data source (in this case, an array of strings)
String[] items = {"Item 1", "Item 2", "Item 3", "Item 4", "Item 5"};
// Create an ArrayAdapter to populate the ListView
ArrayAdapter<String> adapter = new ArrayAdapter<>(this, R.layout.list_item, R.id.item_text_view, items);
// Get a reference to the ListView
ListView listView = findViewById(R.id.my_list_view);
// Set the adapter on the ListView
listView.setAdapter(adapter);
}
}
In this example, R.layout.list_item
refers to the layout file for each list item, and R.id.item_text_view
is the ID of the TextView
in the list item layout.
- Set Up the ListView in XML: In the XML layout file of your activity (e.g.,
activity_my.xml
), add theListView
:
<!-- activity_my.xml -->
<ListView
xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/my_list_view"
android:layout_width="match_parent"
android:layout_height="match_parent" />
- Run Your App: When you run your app, the
ListView
will display the items from your data source, and each item will be displayed according to the layout defined inlist_item.xml
.
This is a basic example of how to implement a ListView
in Android using an ArrayAdapter
. Depending on your specific use case, you may need to customize the adapter or use a different data source. Additionally, you can add event handlers to respond to user interactions with the list items.