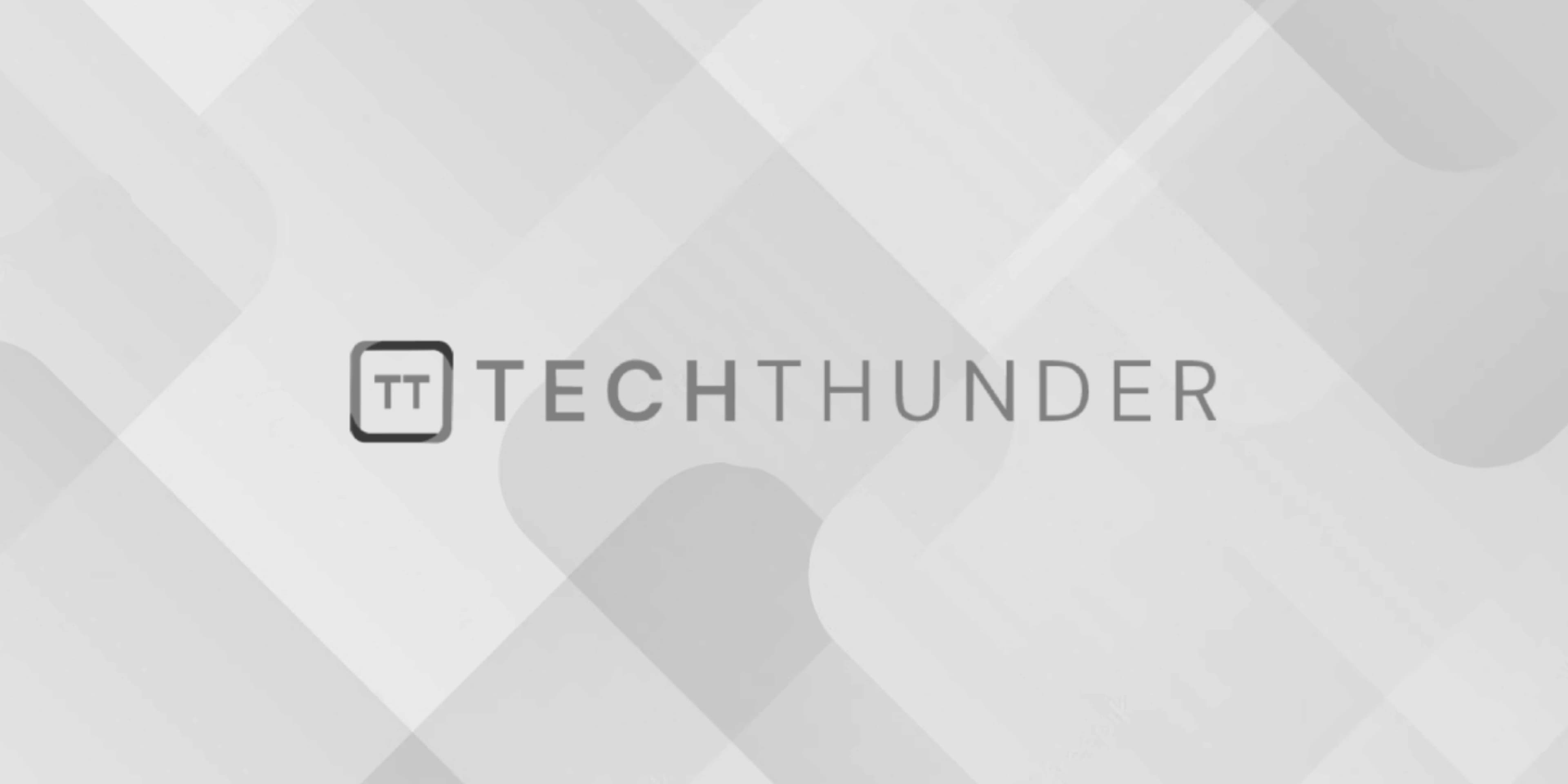
XML Parsing SAX in Android
XML parsing using the Simple API for XML (SAX) in Android allows you to parse and process XML data in a streaming manner, making it efficient for large XML files. Here’s a step-by-step guide on how to perform XML parsing using SAX in Android:
1. Create an XML Document:
Let’s assume you have an XML document named data.xml
that you want to parse. You can place it in the res/xml
directory of your Android project.
2. Initialize SAXParserFactory:
In your Android activity or class, initialize a SAXParserFactory
instance:
import javax.xml.parsers.SAXParser;
import javax.xml.parsers.SAXParserFactory;
SAXParserFactory saxParserFactory = SAXParserFactory.newInstance();
3. Create a Custom Handler:
To handle the parsing events, you need to create a custom DefaultHandler
subclass that overrides methods like startElement
, endElement
, and characters
. These methods are called when the SAX parser encounters XML elements and text content.
import org.xml.sax.Attributes;
import org.xml.sax.SAXException;
import org.xml.sax.helpers.DefaultHandler;
public class MyHandler extends DefaultHandler {
private StringBuilder stringBuilder;
private boolean parsingElement;
@Override
public void startElement(String uri, String localName, String qName, Attributes attributes) throws SAXException {
if (localName.equals("element_name")) { // Replace with the actual XML element name
parsingElement = true;
stringBuilder = new StringBuilder();
}
}
@Override
public void characters(char[] ch, int start, int length) throws SAXException {
if (parsingElement) {
stringBuilder.append(new String(ch, start, length));
}
}
@Override
public void endElement(String uri, String localName, String qName) throws SAXException {
if (parsingElement) {
String elementValue = stringBuilder.toString().trim();
// Process the element value here
parsingElement = false;
}
}
}
Replace "element_name"
with the name of the XML element you want to parse.
4. Parse the XML File:
Use the SAXParser
to parse the XML file with your custom handler:
try {
SAXParser saxParser = saxParserFactory.newSAXParser();
MyHandler handler = new MyHandler();
// Replace "R.xml.data" with the resource identifier of your XML file
InputStream inputStream = getResources().openRawResource(R.xml.data);
saxParser.parse(inputStream, handler);
} catch (Exception e) {
e.printStackTrace();
}
In this code:
MyHandler
is the custom handler you created earlier.getResources().openRawResource(R.xml.data)
opens the XML file as an input stream from the resources.
5. Process the XML Data:
Inside the endElement
method of your custom handler, you can process the parsed XML data as needed. You can store it in data structures or perform any other required actions.
6. Error Handling (Optional):
Be sure to add appropriate error handling to handle exceptions that may occur during parsing.
7. Permissions (if reading from external storage):
If you are reading the XML file from external storage, you may need to request appropriate permissions in your AndroidManifest.xml file and handle them at runtime.
That’s it! You have successfully parsed an XML file using SAX in Android.