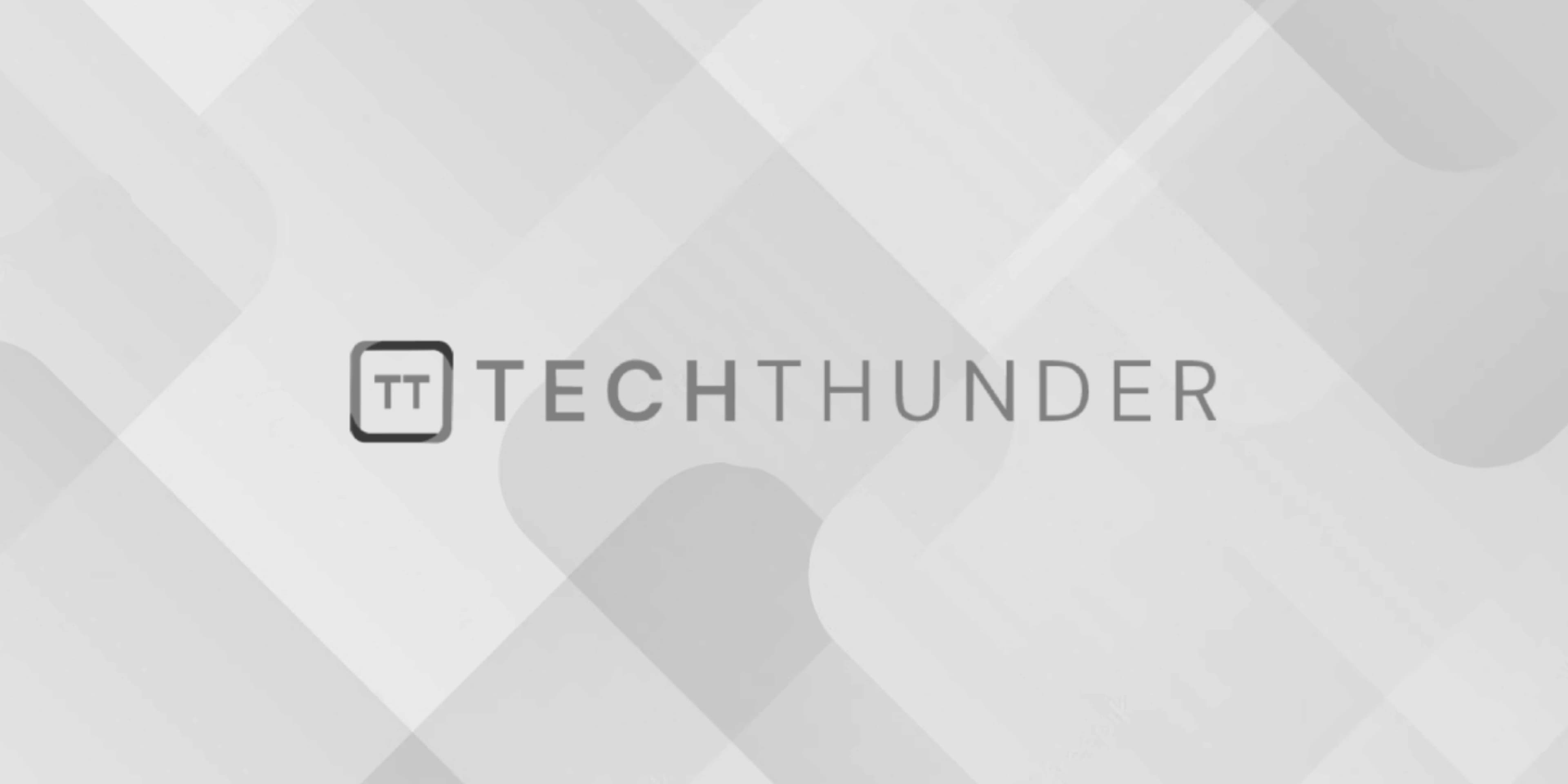
XML Parsing DOM in Android
XML parsing using the Document Object Model (DOM) in Android allows you to load an entire XML document into memory as a tree-like structure, making it easy to navigate and manipulate. Here’s how to perform XML parsing using DOM in Android:
1. Create an XML Document:
Let’s assume you have an XML document named data.xml
that you want to parse. You can place it in the res/xml
directory of your Android project.
2. Initialize a DocumentBuilder:
In your Android activity or class, initialize a DocumentBuilder
instance from the DocumentBuilderFactory
:
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance();
DocumentBuilder builder = factory.newDocumentBuilder();
3. Parse the XML Document:
Load the XML document and parse it using the DocumentBuilder
:
try {
// Replace "R.xml.data" with the resource identifier of your XML file
InputStream is = getResources().openRawResource(R.xml.data);
Document doc = builder.parse(is);
} catch (Exception e) {
e.printStackTrace();
}
In this code:
getResources().openRawResource(R.xml.data)
opens the XML file as an input stream from the resources.
4. Navigate the DOM Tree:
You can navigate and manipulate the parsed XML document as a tree structure. For example, you can access elements, attributes, and text content. Here’s an example:
// Assuming you have a root element named "root" in your XML
Element rootElement = doc.getDocumentElement();
// Access elements within the root element
NodeList itemNodes = rootElement.getElementsByTagName("item");
for (int i = 0; i < itemNodes.getLength(); i++) {
Element itemElement = (Element) itemNodes.item(i);
// Access element attributes
String id = itemElement.getAttribute("id");
// Access element text content
String content = itemElement.getTextContent();
// Process the data as needed
}
In this example:
getDocumentElement()
gets the root element of the XML document.getElementsByTagName("item")
retrieves a list of elements with the specified tag name.getAttribute("id")
retrieves the value of an attribute.getTextContent()
retrieves the text content of an element.
5. Error Handling (Optional):
Be sure to add appropriate error handling to handle exceptions like ParserConfigurationException
, SAXException
, IOException
, and others that may occur during parsing.
6. Finish Parsing:
Release any resources associated with the input stream when you finish parsing:
is.close(); // Close the input stream
7. Permissions (if reading from external storage):
If you are reading the XML file from external storage, you may need to request appropriate permissions in your AndroidManifest.xml file and handle them at runtime.
That’s it! You have successfully parsed an XML file using DOM in Android. You can now work with the parsed data as needed.