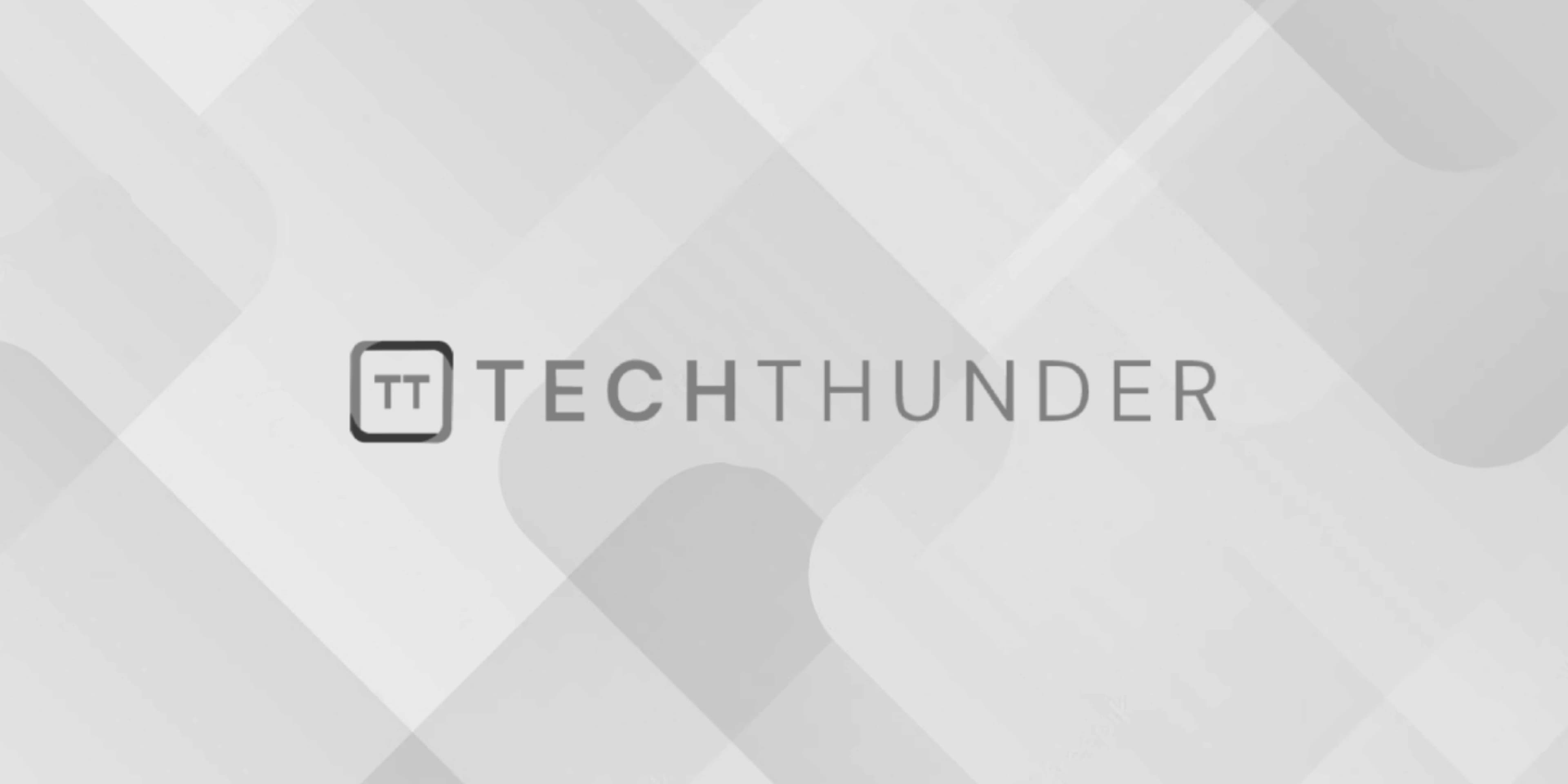
SQLite Tutorial in Android
SQLite is a lightweight and embedded relational database management system that is commonly used in Android app development. It provides a local database for storing and managing structured data within Android applications. Here’s a basic SQLite tutorial for Android:
1. Create a New Android Project:
Start by creating a new Android project in Android Studio. Choose an appropriate project template and configure your project settings.
2. Define the Database Schema:
Before you can work with SQLite in Android, you need to define the database schema. The schema includes the tables, columns, and data types for your database.
// Example: Define a simple table for storing contacts
public class ContactsContract {
public static final String TABLE_NAME = "contacts";
public static final String COLUMN_ID = "id";
public static final String COLUMN_NAME = "name";
public static final String COLUMN_PHONE = "phone";
}
3. Create a Database Helper Class:
To create and manage the SQLite database, create a subclass of SQLiteOpenHelper
. This class handles database creation and version management.
public class DatabaseHelper extends SQLiteOpenHelper {
private static final String DATABASE_NAME = "contacts.db";
private static final int DATABASE_VERSION = 1;
// SQL statement to create the contacts table
private static final String TABLE_CREATE =
"CREATE TABLE " + ContactsContract.TABLE_NAME + " (" +
ContactsContract.COLUMN_ID + " INTEGER PRIMARY KEY AUTOINCREMENT, " +
ContactsContract.COLUMN_NAME + " TEXT, " +
ContactsContract.COLUMN_PHONE + " TEXT);";
public DatabaseHelper(Context context) {
super(context, DATABASE_NAME, null, DATABASE_VERSION);
}
@Override
public void onCreate(SQLiteDatabase db) {
// Create the database table
db.execSQL(TABLE_CREATE);
}
@Override
public void onUpgrade(SQLiteDatabase db, int oldVersion, int newVersion) {
// Upgrade the database if needed (e.g., change the schema)
db.execSQL("DROP TABLE IF EXISTS " + ContactsContract.TABLE_NAME);
onCreate(db);
}
}
4. Open and Close the Database:
To work with the database, you need to open and close it using an instance of SQLiteDatabase
.
DatabaseHelper dbHelper = new DatabaseHelper(context);
SQLiteDatabase db = dbHelper.getWritableDatabase(); // Open the database for writing
// Use the database for CRUD operations (Insert, Select, Update, Delete)
db.close(); // Close the database when done
5. Performing CRUD Operations:
Now that you have opened the database, you can perform CRUD (Create, Read, Update, Delete) operations:
- Insert Data:
ContentValues values = new ContentValues();
values.put(ContactsContract.COLUMN_NAME, "John Doe");
values.put(ContactsContract.COLUMN_PHONE, "123-456-7890");
long newRowId = db.insert(ContactsContract.TABLE_NAME, null, values);
- Query Data:
String[] projection = {
ContactsContract.COLUMN_ID,
ContactsContract.COLUMN_NAME,
ContactsContract.COLUMN_PHONE
};
Cursor cursor = db.query(
ContactsContract.TABLE_NAME,
projection,
null,
null,
null,
null,
null
);
- Update Data:
ContentValues values = new ContentValues();
values.put(ContactsContract.COLUMN_PHONE, "987-654-3210");
int rowsUpdated = db.update(
ContactsContract.TABLE_NAME,
values,
ContactsContract.COLUMN_NAME + " = ?",
new String[]{"John Doe"}
);
- Delete Data:
int rowsDeleted = db.delete(
ContactsContract.TABLE_NAME,
ContactsContract.COLUMN_NAME + " = ?",
new String[]{"John Doe"}
);
6. Handling Transactions (Optional):
You can use transactions to group multiple database operations into a single unit. This ensures that the database remains in a consistent state in case of failures.
db.beginTransaction();
try {
// Perform multiple operations
db.setTransactionSuccessful(); // Mark the transaction as successful
} finally {
db.endTransaction(); // End the transaction
}
7. Close the Database:
Always remember to close the database when you’re done using it to release system resources.
db.close();
This is a basic overview of using SQLite in Android. Depending on your app’s requirements, you may need to implement more complex database operations and queries. Be sure to follow Android’s best practices for database management and use content providers when sharing data across apps.