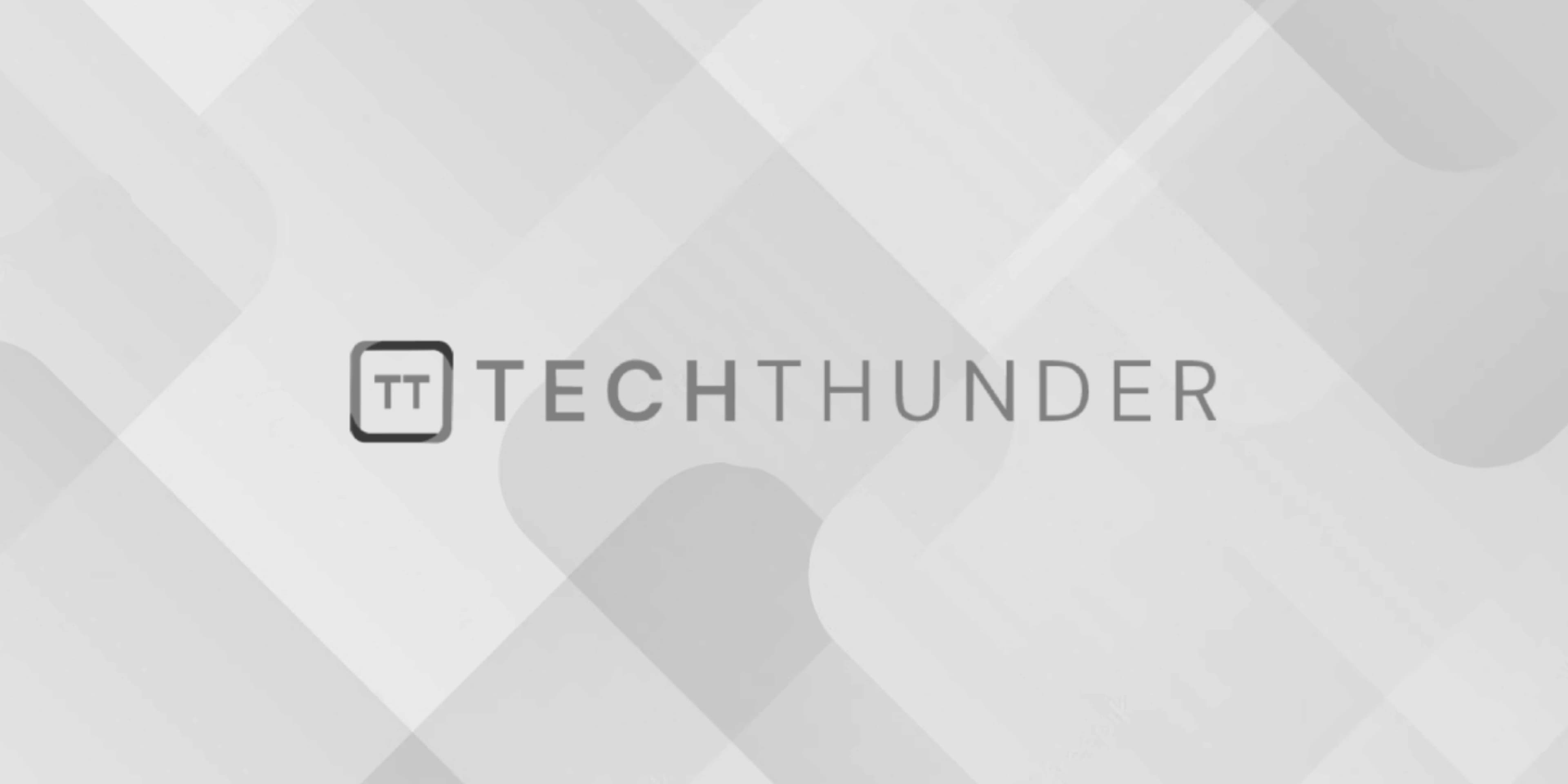
Custom Toast in Android
The Android custom Toast messages to display information or notifications to users with a custom layout or appearance. Custom Toasts allow you to style the message and content according to your app’s design. Here’s how to create a custom Toast in Android:
- Create a Custom Layout: First, you need to create a custom XML layout file for your Toast. This layout file will define how your Toast message should appear. For example, create a file named
custom_toast.xml
in your app’sres/layout
directory:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:orientation="horizontal"
android:padding="16dp"
android:background="@drawable/custom_toast_background">
<ImageView
android:layout_width="48dp"
android:layout_height="48dp"
android:src="@drawable/ic_check" />
<TextView
android:id="@+id/custom_toast_text"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Custom Toast"
android:textColor="#FFFFFF"
android:textSize="16sp" />
</LinearLayout>
In this example, the custom layout contains an ImageView
for an icon and a TextView
for text. You can customize this layout as needed.
- Create a Custom Toast: In your Java or Kotlin code, you can create a custom Toast and set its custom layout:
// Inflate the custom layout
LayoutInflater inflater = getLayoutInflater();
View layout = inflater.inflate(R.layout.custom_toast, findViewById(R.id.custom_toast_layout));
// Find the ImageView and TextView in the custom layout
ImageView imageView = layout.findViewById(R.id.custom_toast_icon);
TextView textView = layout.findViewById(R.id.custom_toast_text);
// Customize the content
imageView.setImageResource(R.drawable.ic_check);
textView.setText("Custom Toast Message");
// Create and display the custom Toast
Toast toast = new Toast(getApplicationContext());
toast.setDuration(Toast.LENGTH_SHORT);
toast.setView(layout);
toast.show();
- Replace
R.layout.custom_toast
with the name of your custom layout file. - Modify the
ImageView
andTextView
to customize the content as needed. - You can customize the duration of the Toast using
toast.setDuration()
.
- Run Your App: When you run your app and trigger the custom Toast, it will display your custom layout with the specified content.
- Customize the Toast Appearance (Optional): You can further customize the appearance of the custom Toast by defining a custom background drawable in the
custom_toast_background
attribute in your custom layout XML, or by applying styling to the components in the layout. For example, to set a custom background for the Toast, create a drawable resource file (e.g.,custom_toast_background.xml
) in yourres/drawable
directory and reference it in your custom layout XML.
<!-- custom_toast_background.xml -->
<shape xmlns:android="http://schemas.android.com/apk/res/android"
android:shape="rectangle">
<corners android:radius="8dp" />
<solid android:color="#FF5722" />
</shape>
Then, in your custom layout XML, set the android:background
attribute to @drawable/custom_toast_background
to apply this background to your custom Toast.
<!-- custom_toast.xml -->
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:orientation="horizontal"
android:padding="16dp"
android:background="@drawable/custom_toast_background">
<!-- ... rest of the layout ... -->
</LinearLayout>
You can create and display custom Toast messages in your Android app with a custom layout and appearance. This allows you to provide a more tailored and visually appealing notification experience for your users.