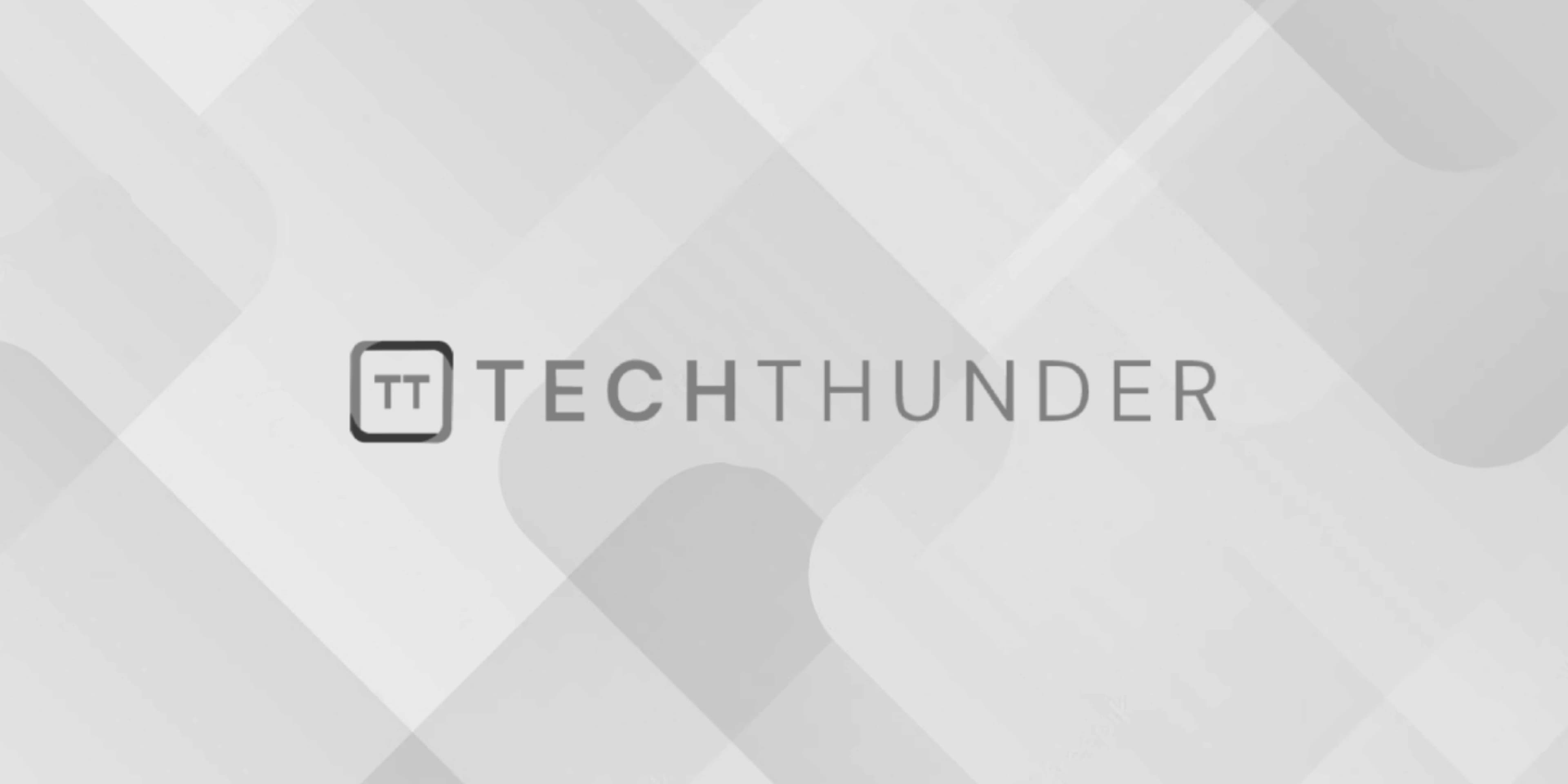
Android Graphics
Android provides a powerful framework for working with graphics and rendering in your Android applications. You can create visually appealing user interfaces, draw custom shapes, images, animations, and more. Here’s an overview of how to work with graphics in Android:
1. Views and Layouts:
The foundation of Android UI development is Views and Layouts. Views are the building blocks of your app’s user interface, and Layouts are used to arrange these Views on the screen.
- Common Views: Android provides various built-in Views such as TextView, ImageView, Button, EditText, etc., which you can use to display text, images, buttons, and user input fields.
- Layouts: Android offers a variety of layout managers like LinearLayout, RelativeLayout, ConstraintLayout, and FrameLayout to organize Views in your app’s UI.
2. Drawing on a Canvas:
To create custom graphics or drawings, you can use the Canvas class. Here’s a basic example of drawing on a Canvas within a custom View:
public class CustomView extends View {
private Paint paint;
public CustomView(Context context) {
super(context);
paint = new Paint();
paint.setColor(Color.BLUE);
paint.setStrokeWidth(5f);
}
@Override
protected void onDraw(Canvas canvas) {
super.onDraw(canvas);
canvas.drawCircle(100, 100, 50, paint); // Draw a blue circle
}
}
3. Bitmaps and Images:
You can display images in your Android app using the ImageView widget or by drawing them on a Canvas. BitmapFactory is commonly used to load images into Bitmap objects.
Bitmap bitmap = BitmapFactory.decodeResource(getResources(), R.drawable.my_image);
ImageView imageView = findViewById(R.id.imageView);
imageView.setImageBitmap(bitmap);
4. Animation:
Android provides animation support for creating fluid and interactive user interfaces. You can use View animations, Property animations, or create custom animations using the Animation framework.
5. OpenGL ES:
For advanced 2D and 3D graphics, you can use the OpenGL ES (Embedded Systems) API, which is a subset of the OpenGL graphics library optimized for mobile devices. It allows you to create high-performance, hardware-accelerated graphics.
6. Vector Graphics:
Android also supports vector graphics through the VectorDrawable class, which enables you to create scalable, resolution-independent graphics that can adapt to different screen sizes and densities.
7. Custom Views:
You can create custom Views by extending existing View classes or by creating entirely new custom Views. This allows you to design unique and interactive UI components tailored to your app’s needs.
8. Graphics Libraries:
You can use third-party graphics libraries for more advanced graphics tasks. Some popular options include OpenGL, Skia, and libraries like Glide and Picasso for image loading and caching.
9. Game Development:
If you’re interested in game development, Android provides the Android Game SDK and libraries like LibGDX, Unity, and Cocos2d-x, which are popular for creating games on the Android platform.
10. UI Theming:
You can customize the look and feel of your app’s graphics by defining themes and styles in XML, allowing for consistent UI design.
Working with graphics in Android offers a wide range of possibilities, from creating simple custom views to developing complex games or visual applications. Depending on your project’s requirements, you can choose the appropriate approach and libraries to achieve your desired graphical effects and interactions.