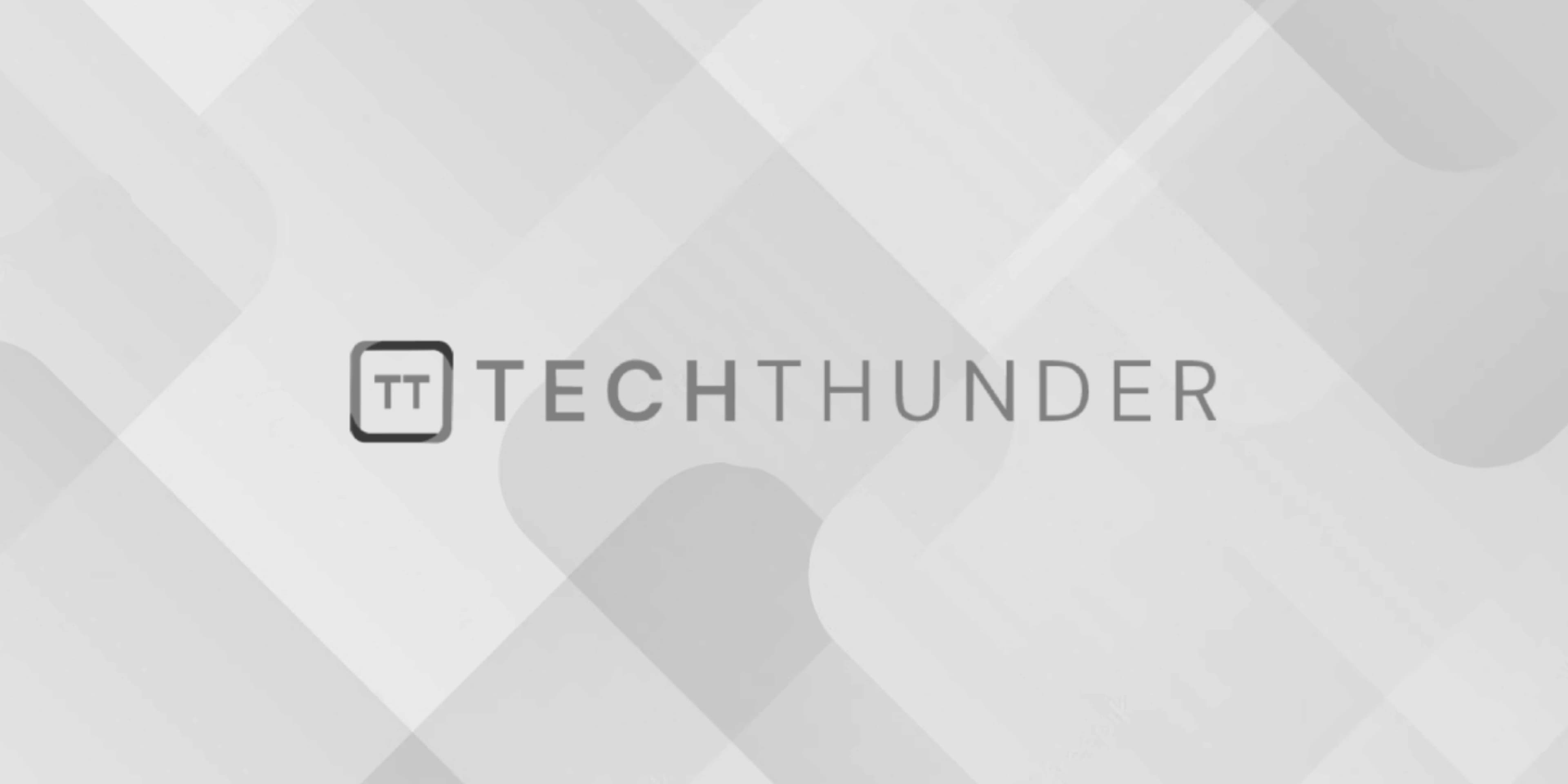
Spinner in Android
The Android Spinner
is a drop-down menu that allows users to select an item from a list of options. It is a commonly used UI component for selecting items when there are multiple choices available. Here’s how you can use a Spinner
in your Android app:
Add a Spinner to Your Layout: In your XML layout file, add a Spinner
element where you want to include the drop-down menu:
<Spinner
android:id="@+id/spinner"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
android:id
: Assign a unique ID to theSpinner
for later reference.android:layout_width
andandroid:layout_height
: Adjust these attributes based on your layout requirements.
Define Data for the Spinner: In your activity or fragment, define the data that you want to populate the Spinner
with. This can be an array of strings, a list of objects, or any other data source. For example, let’s say you have an array of strings:
String[] items = {"Option 1", "Option 2", "Option 3", "Option 4"};
Create an ArrayAdapter: You need an ArrayAdapter
to bind the data to the Spinner
. The ArrayAdapter
converts the data into view items that the Spinner
can display.
ArrayAdapter<String> adapter = new ArrayAdapter<>(this, android.R.layout.simple_spinner_item, items);
adapter.setDropDownViewResource(android.R.layout.simple_spinner_dropdown_item);
this
: Pass the current context (usually the activity).android.R.layout.simple_spinner_item
: This is a built-in layout resource provided by Android for the individual items in the spinner.android.R.layout.simple_spinner_dropdown_item
: This is a built-in layout resource provided by Android for the dropdown items.
Set the Adapter: Apply the adapter to the Spinner
:
Spinner spinner = findViewById(R.id.spinner);
spinner.setAdapter(adapter);
Handle Item Selection: You can set an OnItemSelectedListener
to handle the selection of items:
spinner.setOnItemSelectedListener(new AdapterView.OnItemSelectedListener() {
@Override
public void onItemSelected(AdapterView<?> parent, View view, int position, long id) {
String selectedItem = (String) parent.getItemAtPosition(position);
// Do something with the selected item
}
@Override
public void onNothingSelected(AdapterView<?> parent) {
// Handle no item selected case
}
});
In the onItemSelected
method, you can get the selected item using parent.getItemAtPosition(position)
.
Run Your App: When you run your app, the Spinner
will be displayed. Clicking on it will open a drop-down menu with the available options.
You can easily implement a Spinner
in your Android app to allow users to select an item from a list of options.