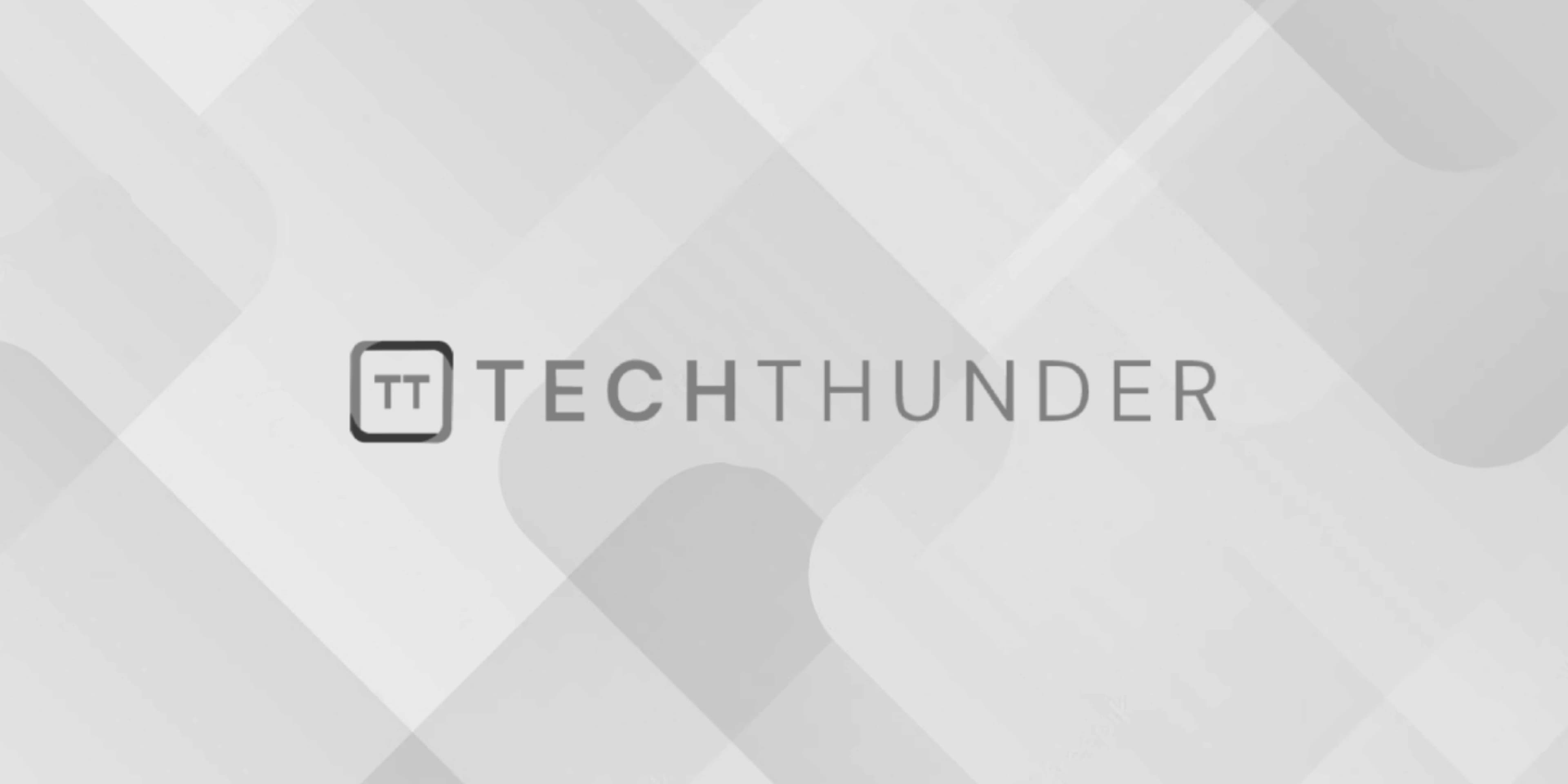
245 views
RatingBar in Android
The Android RatingBar
is a user interface component that allows users to rate items on a scale by selecting a number of stars. It’s commonly used for collecting user ratings or reviews within an app. Here’s how you can use a RatingBar
in Android:
- Add the
RatingBar
to Your Layout: In your XML layout file, add aRatingBar
element where you want to include the rating functionality:
XML
<RatingBar
android:id="@+id/rating_bar"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:numStars="5" <!-- Number of stars in the RatingBar -->
android:rating="0" <!-- Initial rating -->
android:stepSize="1.0" <!-- Step size between ratings -->
android:layout_gravity="center" />
android:id
: Assign a unique ID to theRatingBar
for later reference.android:layout_width
andandroid:layout_height
: Adjust these attributes according to your layout requirements.android:numStars
: Set the number of stars in theRatingBar
.android:rating
: Set the initial rating value (usually 0).android:stepSize
: Set the step size between ratings (e.g., 0.5 to allow half-star ratings).android:layout_gravity
: Adjust the gravity to position theRatingBar
within its parent.
- Access the
RatingBar
in Your Activity or Fragment: In your Java or Kotlin code (usually within your activity or fragment), obtain a reference to theRatingBar
using its ID:
Java
RatingBar ratingBar = findViewById(R.id.rating_bar);
- Rating Change Listener: To respond to rating changes by the user, set a
RatingBar.OnRatingBarChangeListener
:
Java
ratingBar.setOnRatingBarChangeListener(new RatingBar.OnRatingBarChangeListener() {
@Override
public void onRatingChanged(RatingBar ratingBar, float rating, boolean fromUser) {
// Handle the rating change here
// 'rating' contains the new rating value
// 'fromUser' indicates whether the change was initiated by the user
}
});
The onRatingChanged
method is called when the user changes the rating. You can perform actions based on the new rating value.
- Run Your App: When you run your app, the
RatingBar
will be displayed, and users can tap on the stars to select a rating. TheonRatingChanged
method will be called when the user changes the rating. - Customization (Optional): You can customize the appearance of the
RatingBar
by defining custom star drawables, adjusting the size of the stars, or changing the colors. You can do this using XML attributes or programmatically.
XML
<!-- Customizing the RatingBar -->
<RatingBar
android:id="@+id/rating_bar"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:numStars="5"
android:rating="0"
android:stepSize="1.0"
android:layout_gravity="center"
android:progressDrawable="@drawable/custom_progress_drawable"
android:indeterminateDrawable="@drawable/custom_indeterminate_drawable"
android:secondaryProgress="0"
android:isIndicator="false" />
Replace custom_progress_drawable
and custom_indeterminate_drawable
with your custom drawables for the stars.
By following these steps, you can easily implement a RatingBar
in your Android app to collect user ratings or reviews and respond to rating changes as needed.