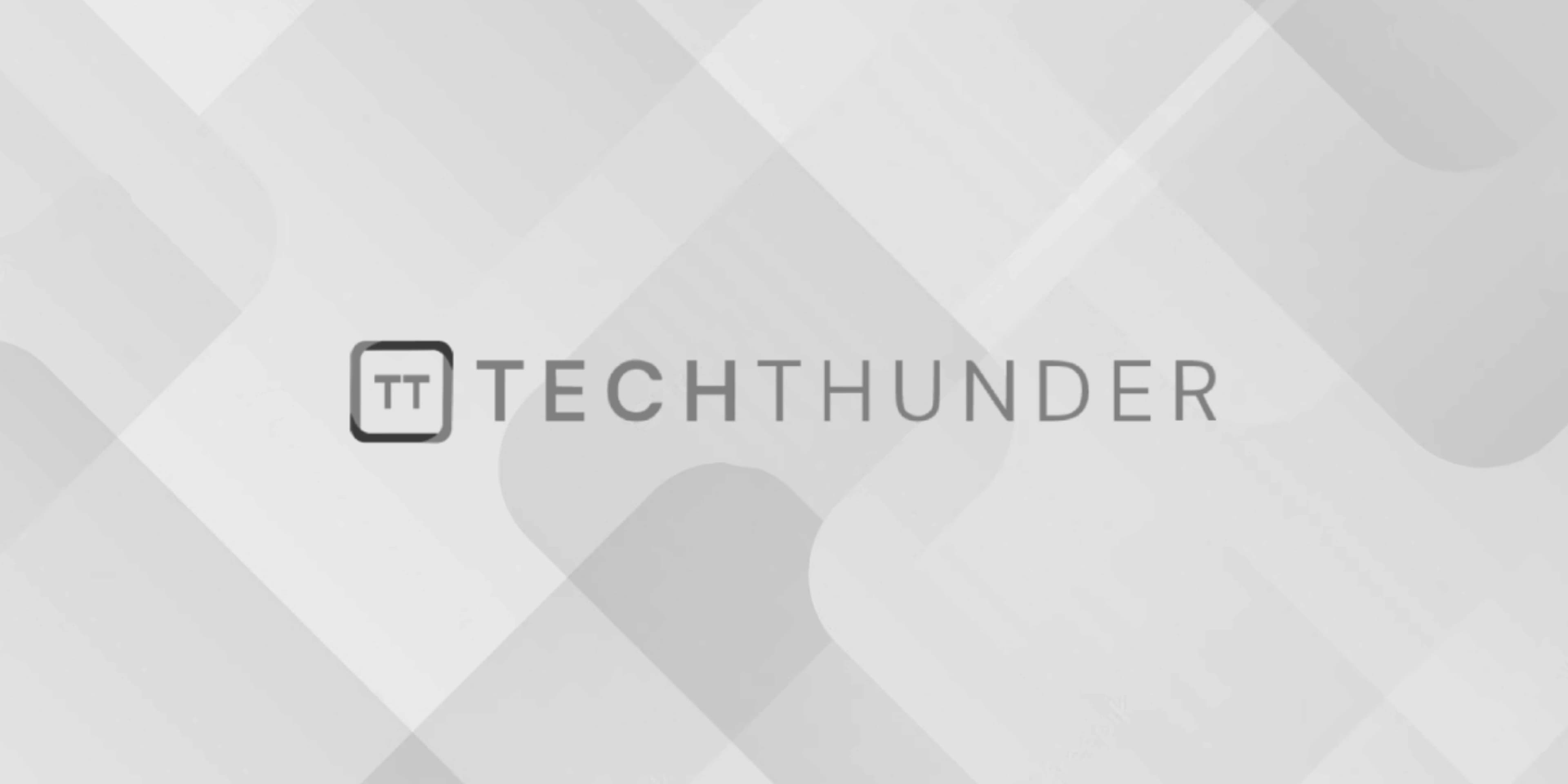
Dynamic RadioButton in Android
The Android dynamic RadioButtons within a RadioGroup programmatically. This can be useful when you need to generate a list of options dynamically based on user input or data from a source like a database or an API. Here’s how you can create dynamic RadioButtons in Android:
Create a RadioGroup in Your XML Layout: In your XML layout file, add a RadioGroup
element where you want to display the dynamic RadioButtons. You can also add a TextView
to provide a label for the group:
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Select an Option" />
<RadioGroup
android:id="@+id/radio_group"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical">
</RadioGroup>
</LinearLayout>
Create RadioButtons Dynamically: In your Java or Kotlin code (usually within your activity or fragment), obtain a reference to the RadioGroup
, and then create and add dynamic RadioButtons to it. You can use a loop to create multiple options based on your data or requirements. Here’s an example in Kotlin:
val radioGroup = findViewById<RadioGroup>(R.id.radio_group)
val options = listOf("Option 1", "Option 2", "Option 3", "Option 4")
for (option in options) {
val radioButton = RadioButton(this)
radioButton.text = option
radioButton.id = View.generateViewId() // Generate a unique ID for each RadioButton
radioGroup.addView(radioButton)
}
- Replace
options
with your list of dynamic options.
Set RadioButton Click Listener (Optional): If you want to perform an action when a RadioButton is clicked, you can set an OnClickListener
for each RadioButton:
for (option in options) {
val radioButton = RadioButton(this)
radioButton.text = option
radioButton.id = View.generateViewId()
radioGroup.addView(radioButton)
radioButton.setOnClickListener {
val selectedOption = radioButton.text.toString()
// Perform an action based on the selected option
Toast.makeText(this, "Selected: $selectedOption", Toast.LENGTH_SHORT).show()
}
}
This code snippet shows a Toast message when a RadioButton is clicked. You can replace it with your desired action.
Retrieve Selected RadioButton: To retrieve the selected RadioButton from the RadioGroup, you can use the checkedRadioButtonId
property of the RadioGroup:
val selectedRadioButtonId = radioGroup.checkedRadioButtonId
if (selectedRadioButtonId != -1) {
val selectedRadioButton = findViewById<RadioButton>(selectedRadioButtonId)
val selectedOption = selectedRadioButton.text.toString()
// Perform an action based on the selected option
Toast.makeText(this, "Selected: $selectedOption", Toast.LENGTH_SHORT).show()
}
This code retrieves the selected RadioButton’s text when needed.
You can create dynamic RadioButtons within a RadioGroup in your Android app. Users can select one option from the list, and you can handle their selection based on your application’s logic.