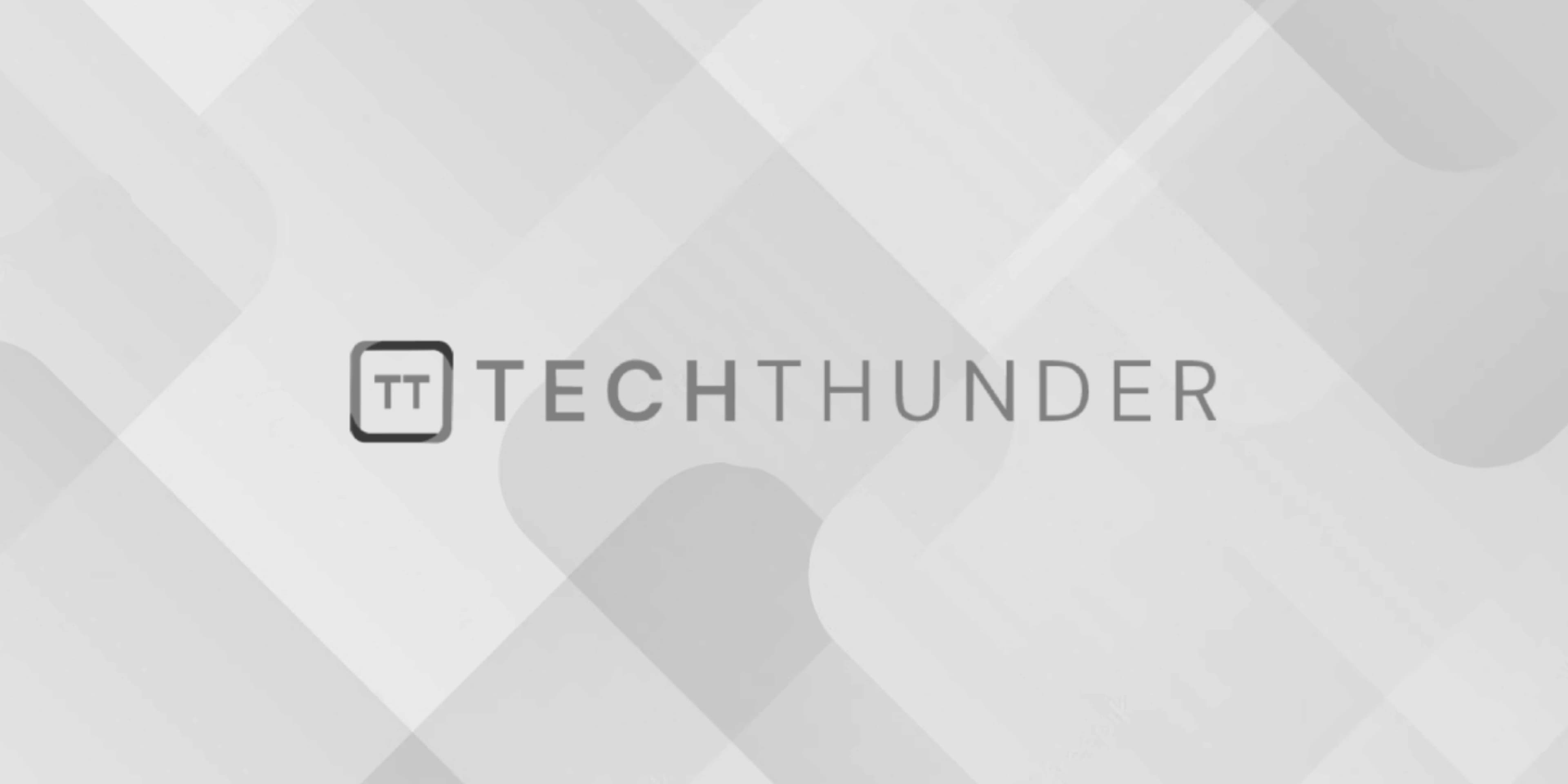
ProgressBar in Android
The Android ProgressBar
is a user interface component used to indicate the progress of an ongoing task or operation. You can use a ProgressBar
to show users that something is happening in the background and to give them feedback on the progress of the task. Android provides two main types of ProgressBar
: the determinate ProgressBar
, which displays progress as a percentage, and the indeterminate ProgressBar
, which shows an ongoing process without indicating a specific completion percentage.
Here’s how to use both types of ProgressBar
in Android:
Determinate ProgressBar:
- Add the Determinate ProgressBar to Your Layout: In your XML layout file, add a
ProgressBar
element with the style attribute set to@android:style/Widget.ProgressBar.Horizontal
to create a horizontal determinateProgressBar
. You can adjust the width, height, and other attributes to fit your layout:
<ProgressBar
android:id="@+id/determinate_progress_bar"
style="@android:style/Widget.ProgressBar.Horizontal"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_gravity="center"
/>
android:id
: Assign a unique ID to theProgressBar
for later reference.style
: Use the style attribute to specify the style of theProgressBar
. Setting it to@android:style/Widget.ProgressBar.Horizontal
creates a horizontal determinateProgressBar
.android:layout_width
andandroid:layout_height
: Adjust these attributes based on your layout requirements.
- Access the Determinate ProgressBar in Your Activity or Fragment: In your Java or Kotlin code (usually within your activity or fragment), obtain a reference to the
ProgressBar
using its ID:
ProgressBar determinateProgressBar = findViewById(R.id.determinate_progress_bar);
- Update Progress: To update the progress of the determinate
ProgressBar
, you can use thesetProgress
method:
int progressValue = 50; // Set the progress value (0-100)
determinateProgressBar.setProgress(progressValue);
You can call setProgress
at various points during the execution of your task to update the progress.
Indeterminate ProgressBar:
- Add the Indeterminate ProgressBar to Your Layout: In your XML layout file, add a
ProgressBar
element with the style attribute set to@android:style/Widget.ProgressBar
. This creates an indeterminateProgressBar
:
<ProgressBar
android:id="@+id/indeterminate_progress_bar"
style="@android:style/Widget.ProgressBar"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center"
/>
android:id
: Assign a unique ID to theProgressBar
for later reference.style
: Use the style attribute to specify the style of theProgressBar
. Setting it to@android:style/Widget.ProgressBar
creates an indeterminateProgressBar
.android:layout_width
andandroid:layout_height
: Adjust these attributes based on your layout requirements.
- Access the Indeterminate ProgressBar in Your Activity or Fragment: In your Java or Kotlin code (usually within your activity or fragment), obtain a reference to the
ProgressBar
using its ID:
ProgressBar indeterminateProgressBar = findViewById(R.id.indeterminate_progress_bar);
- Start and Stop Animation: The indeterminate
ProgressBar
animates automatically when it’s displayed. You can start and stop the animation programmatically if needed. For example, to start the animation:
indeterminateProgressBar.setVisibility(View.VISIBLE); // Make the ProgressBar visible
To stop the animation:
indeterminateProgressBar.setVisibility(View.INVISIBLE); // Hide the ProgressBar
You can implement both determinate and indeterminate ProgressBar
in your Android app to provide visual feedback to users about ongoing tasks or operations.